What con-estado do for your deeply nested React App state?
State management in React can be complex, especially when dealing with deeply nested data structures. Enter con-estado - a powerful state management library that makes handling complex state both elegant and efficient. Managing state effectively: import { ChangeEvent } from 'react'; import { useCon, ConStateKeys } from 'con-estado'; // Define your initial state const initialState = { user: { name: 'John', preferences: { theme: 'light' as 'light' | 'dark', notifications: { email: true, }, }, }, }; export default function App() { const [state, { acts }] = useCon(initialState, { acts: ({ set, setWrap }) => ({ onChangeInput: ( ev: ChangeEvent ) => { const name = ev.target.name as ConStateKeys; const value = ev.target.value; set(name, value); }, onClickNotifyEmail: setWrap( 'user.preferences.notifications.email', (props) => { props.draft = !props.draft; } ), }), }); return ( Welcome {state.user.name} Toggle Email Notifications:{' '} {state.user.preferences.notifications.email ? 'OFF' : 'ON'} Light Dark ); } Direct Path Updates: Forget about spreading multiple levels deep. con-estado lets you update nested properties using simple dot notation or arrays: set('user.preferences.theme', 'dark') Instead of: setState(prev => ({ ...prev, user: { ...prev.user, preferences: { ...prev.preferences, theme: 'dark' } } })) Performance By Design Built on Mutative (faster than Immer), con-estado ensures: Only modified portions of state create new references Prevents unnecessary re-renders through smart subscriptions Optimized selector-based consumption Type Safety Without Compromise Full TypeScript support means: Strongly inferred state types Type-safe selectors Autocomplete for state paths Real-World Benefits For Teams Reduced boilerplate means faster development Type safety prevents runtime errors Consistent patterns across the codebase Easy to understand and maintain For Performance Efficient updates to large state trees Granular re-renders Optimized subscriptions Memory efficient For Developers Intuitive API Flexible action creators Powerful selector system Great developer experience When to Choose con-estado con-estado shines when your application has: Complex nested state structures Performance-sensitive operations Frequent partial updates Need for type safety Team collaboration Getting Started npm i con-estado The Edge Over Alternatives While other state management solutions exist, con-estado combines: The speed of Mutative The simplicity of dot notation The power of selectors The safety of TypeScript This makes it an excellent choice for modern React applications that need both power and simplicity. Ready to transform your state management? Con-estado delivers the perfect balance of performance, type safety, and developer experience. Try it in your next project and experience the difference. Get started with con-estado today
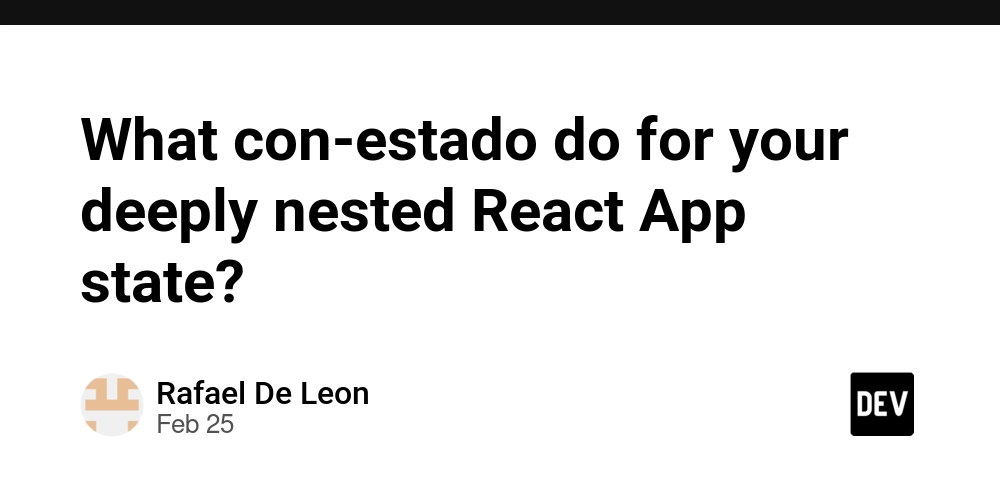
State management in React can be complex, especially when dealing with deeply nested data structures. Enter con-estado
- a powerful state management library that makes handling complex state both elegant and efficient.
Managing state effectively:
import { ChangeEvent } from 'react';
import { useCon, ConStateKeys } from 'con-estado';
// Define your initial state
const initialState = {
user: {
name: 'John',
preferences: {
theme: 'light' as 'light' | 'dark',
notifications: {
email: true,
},
},
},
};
export default function App() {
const [state, { acts }] = useCon(initialState, {
acts: ({ set, setWrap }) => ({
onChangeInput: (
ev: ChangeEvent<HTMLInputElement | HTMLSelectElement>
) => {
const name = ev.target.name as ConStateKeys<typeof initialState>;
const value = ev.target.value;
set(name, value);
},
onClickNotifyEmail: setWrap(
'user.preferences.notifications.email',
(props) => {
props.draft = !props.draft;
}
),
}),
});
return (
<div>
<h1>Welcome {state.user.name}h1>
<input
type="text"
name="user.name"
value={state.user.name}
onChange={acts.onChangeInput}
/>
<button onClick={acts.onClickNotifyEmail}>
Toggle Email Notifications:{' '}
{state.user.preferences.notifications.email ? 'OFF' : 'ON'}
button>
<select
value={state.user.preferences.theme}
name="user.preferences.theme"
onChange={acts.onChangeInput}
>
<option value="light">Lightoption>
<option value="dark">Darkoption>
select>
div>
);
}
Direct Path Updates:
Forget about spreading multiple levels deep. con-estado
lets you update nested properties using simple dot notation or arrays:
set('user.preferences.theme', 'dark')
Instead of:
setState(prev => ({
...prev,
user: {
...prev.user,
preferences: {
...prev.preferences,
theme: 'dark'
}
}
}))
Performance By Design
Built on Mutative (faster than Immer), con-estado
ensures:
- Only modified portions of state create new references
- Prevents unnecessary re-renders through smart subscriptions
- Optimized selector-based consumption
Type Safety Without Compromise
Full TypeScript support means:
- Strongly inferred state types
- Type-safe selectors
- Autocomplete for state paths
Real-World Benefits
For Teams
- Reduced boilerplate means faster development
- Type safety prevents runtime errors
- Consistent patterns across the codebase
- Easy to understand and maintain
For Performance
- Efficient updates to large state trees
- Granular re-renders
- Optimized subscriptions
- Memory efficient
For Developers
- Intuitive API
- Flexible action creators
- Powerful selector system
- Great developer experience
When to Choose con-estado
con-estado
shines when your application has:
- Complex nested state structures
- Performance-sensitive operations
- Frequent partial updates
- Need for type safety
- Team collaboration
Getting Started
npm i con-estado
The Edge Over Alternatives
While other state management solutions exist, con-estado
combines:
- The speed of Mutative
- The simplicity of dot notation
- The power of selectors
- The safety of TypeScript
This makes it an excellent choice for modern React applications that need both power and simplicity.
Ready to transform your state management? Con-estado delivers the perfect balance of performance, type safety, and developer experience. Try it in your next project and experience the difference.