Vibe Coders Ain’t Developer, Bro
let’s talk about something that’s been grinding my gears lately. You got these folks out here calling themselves “software engineers” just ‘cause they can slap together some code in VS Code with a lo-fi playlist in the background. Bruh, that’s not engineering—that’s vibe coding. And it’s time we call it out. Real software engineering isn’t about “vibing” with your IDE or copy-pasting from Stack Overflow. It’s about understanding the why behind the code, not just the how. These vibe coders? They’re out here faking it, and it shows when you dig even a little deep. Look, I’m 24, I’ve been grinding through codebases, debugging at 3 a.m., and actually learning the foundations of computer science. I’m not gatekeeping—anyone can learn this stuff! But you gotta put in the work. Vibe coders? They skip the hard stuff, chase the aesthetic, and think they’re the next Elon Musk ‘cause they made a Todo app with React. Nah, fam, that’s not how it works. They Don’t Get the Basics Here’s the thing: vibe coders are allergic to foundational knowledge. They’ll hype up their “full-stack” skills on LinkedIn, but ask them about something basic like time complexity, and they’re ghosting you faster than a bad Tinder date. Let’s break it down with an example. Say you’re writing a function to search for an item in a list. A vibe coder might whip up something like this: def find_item(lst, item): for x in lst: if x == item: return True return False Cool, it works. They’ll push it to GitHub, add some emojis to the commit message, and call it a day. But hold up—what’s the time complexity of that? It’s O(n), meaning if your list has a million items, it’s gonna chug through every single one in the worst case. A real engineer knows that if the list is sorted, you can use binary search to drop that to O(log n). That’s a massive difference in performance. But vibe coders? They don’t even know what “sorted” means in this context, let alone how to implement binary search. Here’s what a binary search looks like, for the uninitiated: def binary_search(lst, item): left, right = 0, len(lst) - 1 while left
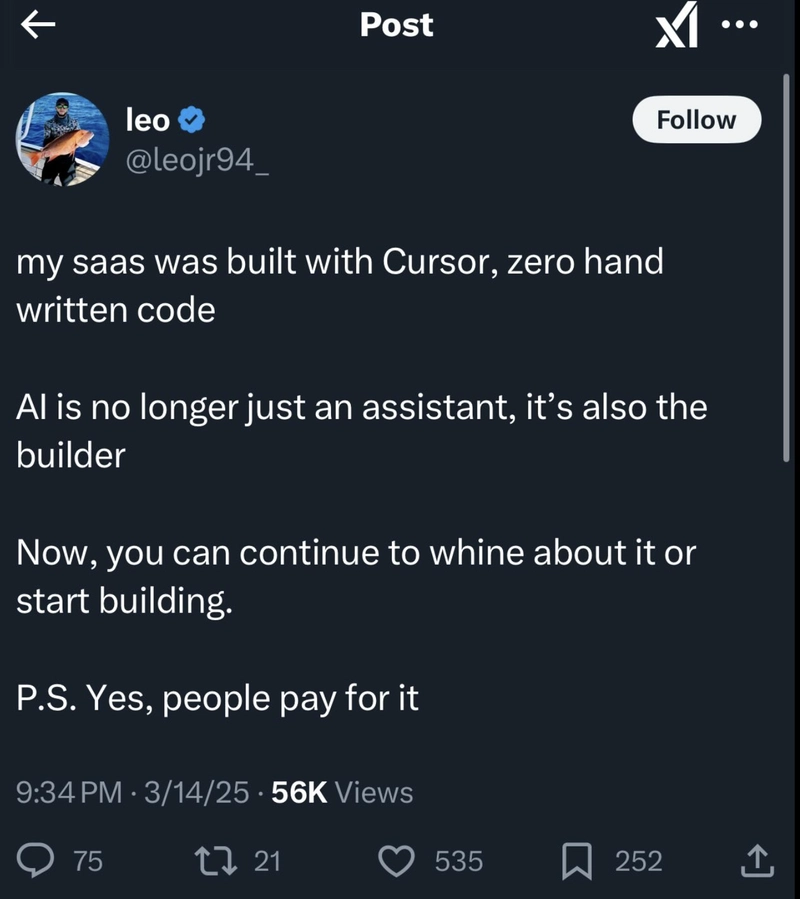
let’s talk about something that’s been grinding my gears lately. You got these folks out here calling themselves “software engineers” just ‘cause they can slap together some code in VS Code with a lo-fi playlist in the background. Bruh, that’s not engineering—that’s vibe coding. And it’s time we call it out. Real software engineering isn’t about “vibing” with your IDE or copy-pasting from Stack Overflow. It’s about understanding the why behind the code, not just the how. These vibe coders? They’re out here faking it, and it shows when you dig even a little deep.
Look, I’m 24, I’ve been grinding through codebases, debugging at 3 a.m., and actually learning the foundations of computer science. I’m not gatekeeping—anyone can learn this stuff! But you gotta put in the work. Vibe coders? They skip the hard stuff, chase the aesthetic, and think they’re the next Elon Musk ‘cause they made a Todo app with React. Nah, fam, that’s not how it works.
They Don’t Get the Basics
Here’s the thing: vibe coders are allergic to foundational knowledge. They’ll hype up their “full-stack” skills on LinkedIn, but ask them about something basic like time complexity, and they’re ghosting you faster than a bad Tinder date. Let’s break it down with an example.
Say you’re writing a function to search for an item in a list. A vibe coder might whip up something like this:
def find_item(lst, item):
for x in lst:
if x == item:
return True
return False
Cool, it works. They’ll push it to GitHub, add some emojis to the commit message, and call it a day. But hold up—what’s the time complexity of that? It’s O(n), meaning if your list has a million items, it’s gonna chug through every single one in the worst case. A real engineer knows that if the list is sorted, you can use binary search to drop that to O(log n). That’s a massive difference in performance. But vibe coders? They don’t even know what “sorted” means in this context, let alone how to implement binary search.
Here’s what a binary search looks like, for the uninitiated:
def binary_search(lst, item):
left, right = 0, len(lst) - 1
while left <= right:
mid = (left + right) // 2
if lst[mid] == item:
return True
elif lst[mid] < item:
left = mid + 1
else:
right = mid - 1
return False
This is foundational computer science, y’all. It’s not sexy, it’s not “vibes,” but it’s what separates the engineers from the posers. Vibe coders don’t know this stuff ‘cause they never cracked open an algorithms book or took a data structures class. They’re too busy tweaking their VS Code theme to match their Spotify playlist.
It’s Not Just Code, It’s Craft
Software engineering is a craft. It’s about building systems that scale, that don’t crash when 10,000 users hit it at once, that don’t make the next dev wanna yeet their laptop out a window. Vibe coders don’t get that. They’ll throw together a Node.js app with 17 nested callbacks, call it “functional,” and act like they invented microservices. Meanwhile, a real engineer is thinking about memory management, concurrency, and how to make the code maintainable for the poor soul who inherits it.
And don’t get me started on their “learn to code in 30 days” bootcamp energy. Look, bootcamps can be dope for getting started, but they’re not a shortcut to being an engineer. You can’t skip the part where you learn how a CPU actually executes your code or why your database query is taking 10 seconds. That stuff takes time, not just vibes.
The Vibe Coder Aesthetic Ain’t It
You know what else bugs me? The vibe coder aesthetic. They’re out here with their RGB keyboards, posting “coding setup” pics on Instagram like it’s a personality trait. Bro, your dual monitors and neon lights don’t make you an engineer. Writing clean, efficient, well-documented code does. Stop flexing your Arch Linux install and go learn what a hash table is.
Real Talk: You Can Do Better
If you’re a vibe coder reading this, I’m not hating on you—I’m calling you to level up. You don’t need a CS degree to be a software engineer, but you do need to understand the foundations. Pick up a book like “Cracking the Coding Interview” or watch some MIT OpenCourseWare lectures. Learn about Big-O notation, data structures, and how operating systems work. It’s not glamorous, but it’s what makes you legit.
To the real engineers out there grinding, keep doing you. We see you debugging that gnarly race condition while vibe coders are busy tweeting about their “side hustle.” You’re the ones building the future, not just chasing clout.
Rant over. Now go write some code that doesn’t suck. ✌️`