Using Observables for Reactive Programming
Using Observables for Reactive Programming: An In-Depth Guide Introduction The evolution of JavaScript and its ecosystem over the last two decades has catalyzed the rise of various programming paradigms, notably reactive programming. Observables have emerged as a cornerstone of this paradigm, especially with the advent of libraries like RxJS (Reactive Extensions for JavaScript). This article aims to explore the historical context of reactive programming and Observables, provide multiple sophisticated code examples, investigate nuances and edge cases, and discuss real-world applications, performance considerations, optimization strategies, and advanced debugging techniques. Historical Context Reactive programming as a concept gained traction in the early 2000s with the advancement of asynchronous programming paradigms. While the traditional imperative programming model operates on static data streams, reactive programming focuses on data as flows or streams that can be observed and reacted to. Early Concepts The roots of reactive programming can be traced back to functional programming languages such as Haskell and Scala, where the notions of immutability and higher-order functions are foundational. Libraries and frameworks that emerged in the late 2000s and early 2010s, such as CommonJS's async and later promises introduced in ECMAScript 6 (ES6), made asynchronous programming more manageable but lacked built-in support for complex data flows and event handling. Emergence of RxJS In 2010, Microsoft introduced RxJS as part of the Reactive Extensions project. RxJS encapsulated the observer pattern to manage asynchronous data streams by providing a consistent API for composing asynchronous and event-based programs. The term "Observable" was born here, introducing a powerful abstraction for working with streams of data. The Observable Pattern Definition An Observable is an object that represents a collection of future values or events. It defines a subscription model where an observer subscribes to an observable to receive notifications of new data, errors, or completion. Core Components Observable: A representation of a stream of values over time. Observer: An object that defines callback functions to handle emissions from the observable (next, error, complete). Subscription: The connection between an observer and an observable which allows the observer to receive data. Operators: Functions that enable you to transform, filter, and compose observables. Basic Observable Example import { Observable } from 'rxjs'; const observable = new Observable(subscriber => { subscriber.next('Hello'); subscriber.next('World'); subscriber.complete(); }); observable.subscribe({ next(x) { console.log(x); }, error(err) { console.error('Something went wrong: ' + err); }, complete() { console.log('Completed'); } }); In this example, the observable emits two strings and then completes. Advanced Code Examples Creating Custom Observables Advanced use cases often require the creation of more complex observables. Below is an example of a custom observable loader that simulates fetching data from an API. import { Observable } from 'rxjs'; function fetchData(url) { return new Observable(subscriber => { const xhr = new XMLHttpRequest(); xhr.open('GET', url, true); xhr.onload = () => { if (xhr.status === 200) { subscriber.next(JSON.parse(xhr.responseText)); subscriber.complete(); } else { subscriber.error(`Error: ${xhr.status}`); } }; xhr.onerror = () => subscriber.error('Error fetching the data'); xhr.send(); // Cleanup if the subscription is closed return () => xhr.abort(); }); } fetchData('https://api.example.com/data') .subscribe({ next(data) { console.log('Data received:', data); }, error(err) { console.error(err); }, complete() { console.log('Data fetching complete'); } }); Composing Multiple Observables One of the greatest strengths of Observables is composing them using operators. The following example shows how to combine multiple observables using merge and forkJoin. import { of, merge, forkJoin } from 'rxjs'; import { delay } from 'rxjs/operators'; const obs1 = of('Hello').pipe(delay(1000)); const obs2 = of('World').pipe(delay(2000)); const mergedObservable = merge(obs1, obs2); mergedObservable.subscribe(value => console.log(value)); const forkedObservable = forkJoin([obs1, obs2]); forkedObservable.subscribe(values => console.log('Forked:', values)); Error Handling with Observables Error handling can be managed using the catchError operator. Here’s an example that includes err
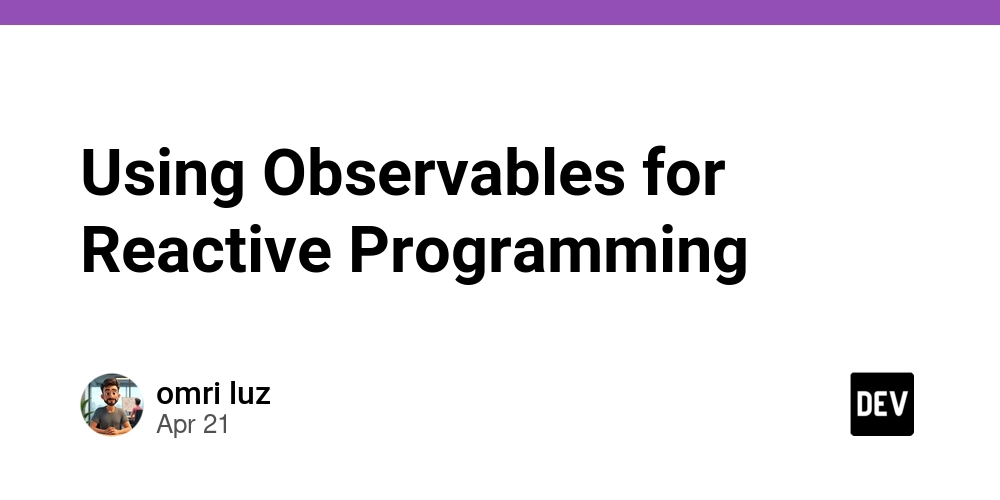
Using Observables for Reactive Programming: An In-Depth Guide
Introduction
The evolution of JavaScript and its ecosystem over the last two decades has catalyzed the rise of various programming paradigms, notably reactive programming. Observables have emerged as a cornerstone of this paradigm, especially with the advent of libraries like RxJS (Reactive Extensions for JavaScript). This article aims to explore the historical context of reactive programming and Observables, provide multiple sophisticated code examples, investigate nuances and edge cases, and discuss real-world applications, performance considerations, optimization strategies, and advanced debugging techniques.
Historical Context
Reactive programming as a concept gained traction in the early 2000s with the advancement of asynchronous programming paradigms. While the traditional imperative programming model operates on static data streams, reactive programming focuses on data as flows or streams that can be observed and reacted to.
Early Concepts
The roots of reactive programming can be traced back to functional programming languages such as Haskell and Scala, where the notions of immutability and higher-order functions are foundational. Libraries and frameworks that emerged in the late 2000s and early 2010s, such as CommonJS's async
and later promises introduced in ECMAScript 6 (ES6), made asynchronous programming more manageable but lacked built-in support for complex data flows and event handling.
Emergence of RxJS
In 2010, Microsoft introduced RxJS as part of the Reactive Extensions project. RxJS encapsulated the observer pattern to manage asynchronous data streams by providing a consistent API for composing asynchronous and event-based programs. The term "Observable" was born here, introducing a powerful abstraction for working with streams of data.
The Observable Pattern
Definition
An Observable is an object that represents a collection of future values or events. It defines a subscription model where an observer subscribes to an observable to receive notifications of new data, errors, or completion.
Core Components
- Observable: A representation of a stream of values over time.
-
Observer: An object that defines callback functions to handle emissions from the observable (
next
,error
,complete
). - Subscription: The connection between an observer and an observable which allows the observer to receive data.
- Operators: Functions that enable you to transform, filter, and compose observables.
Basic Observable Example
import { Observable } from 'rxjs';
const observable = new Observable(subscriber => {
subscriber.next('Hello');
subscriber.next('World');
subscriber.complete();
});
observable.subscribe({
next(x) { console.log(x); },
error(err) { console.error('Something went wrong: ' + err); },
complete() { console.log('Completed'); }
});
In this example, the observable emits two strings and then completes.
Advanced Code Examples
Creating Custom Observables
Advanced use cases often require the creation of more complex observables. Below is an example of a custom observable loader that simulates fetching data from an API.
import { Observable } from 'rxjs';
function fetchData(url) {
return new Observable(subscriber => {
const xhr = new XMLHttpRequest();
xhr.open('GET', url, true);
xhr.onload = () => {
if (xhr.status === 200) {
subscriber.next(JSON.parse(xhr.responseText));
subscriber.complete();
} else {
subscriber.error(`Error: ${xhr.status}`);
}
};
xhr.onerror = () => subscriber.error('Error fetching the data');
xhr.send();
// Cleanup if the subscription is closed
return () => xhr.abort();
});
}
fetchData('https://api.example.com/data')
.subscribe({
next(data) {
console.log('Data received:', data);
},
error(err) {
console.error(err);
},
complete() {
console.log('Data fetching complete');
}
});
Composing Multiple Observables
One of the greatest strengths of Observables is composing them using operators. The following example shows how to combine multiple observables using merge
and forkJoin
.
import { of, merge, forkJoin } from 'rxjs';
import { delay } from 'rxjs/operators';
const obs1 = of('Hello').pipe(delay(1000));
const obs2 = of('World').pipe(delay(2000));
const mergedObservable = merge(obs1, obs2);
mergedObservable.subscribe(value => console.log(value));
const forkedObservable = forkJoin([obs1, obs2]);
forkedObservable.subscribe(values => console.log('Forked:', values));
Error Handling with Observables
Error handling can be managed using the catchError
operator. Here’s an example that includes error handling.
import { of, throwError } from 'rxjs';
import { catchError } from 'rxjs/operators';
const failObservable = throwError('Something went wrong!');
failObservable.pipe(
catchError(err => {
console.error(err);
return of('Fallback data');
})
).subscribe(value => console.log(value));
Edge Cases and Advanced Implementation Techniques
Handling Unsubscription
Memory leaks can lead to performance bottlenecks. It’s essential to unsubscribe from observables when they are no longer needed. This can be accomplished using the takeUntil
operator:
import { Subject, interval } from 'rxjs';
import { takeUntil } from 'rxjs/operators';
const unsubscribeSignal = new Subject();
const obs = interval(1000).pipe(takeUntil(unsubscribeSignal));
const subscription = obs.subscribe(value => console.log(value));
// Trigger unsubscribe after 5 seconds
setTimeout(() => {
unsubscribeSignal.next();
unsubscribeSignal.complete();
}, 5000);
Creating a Debounce Mechanism
Debouncing is an essential technique to prevent high-frequency events from firing and is particularly useful in scenarios like search input handling:
import { fromEvent } from 'rxjs';
import { debounceTime } from 'rxjs/operators';
const inputElement = document.getElementById('search');
fromEvent(inputElement, 'input').pipe(
debounceTime(300) // wait for 300ms pause in events
).subscribe(event => {
console.log(event.target.value);
});
Comparison with Alternative Approaches
While Observables provide a powerful paradigm for managing async behavior, other patterns and libraries exist:
- Promises: A promise represents a single immanent future value, making it less suitable for modeling continuous data streams.
- Callbacks: Traditional callbacks can lead to “callback hell” and convoluted code. Observables maintain a cleaner, more declarative syntax.
- async/await: A syntactic sugar for promises that improves readability but still hinges on the single-value resolution model.
Trade-offs
- Observables offer complex event handling through operators but come with a learning curve.
- Promises simplify the chain of asynchronous operations but lack the granularity to manage streams of events.
Real-World Use Cases
User Interface Interaction
In modern UIs, reactive programming shines in handling user interactions, such as button clicks, form inputs, and animations. Frameworks like Angular extensively utilize RxJS for managing state and event streams, enabling developers to encapsulate complex interactions into manageable code.
Data Fetching and State Management
Observables are powerful when integrating with APIs. Tools such as Redux-Observable combine Redux and RxJS to manage asynchronous actions and side effects in a scalable way.
Complex Event Streams
Observable patterns can efficiently manage events in applications like live chat or real-time notifications by providing a way to compose multiple asynchronous sources of data.
Performance Considerations and Optimization Strategies
Performance Bottlenecks
- Avoid Tight Coupling: Keeping observables modular enhances testability and performance.
- Unsubscribe Properly: Always ensure observables are unsubscribed properly to avoid memory leaks.
-
Use Operators Wisely: Some operators can be more CPU-intensive than others (e.g.,
combineLatest
). Review performance implications.
Production Optimization
- Cold vs. Hot Observables: Cold observables create their own execution context for each subscription, while hot observables share the execution context. Choose based on your data flow requirements.
-
Batching and Throttling: Utilize
batch
andthrottleTime
to manage the frequency of emitted values.
Debugging Techniques
Advanced Debugging
Leveraging the power of RxJS, the tap
operator can be instrumental for debugging:
import { tap } from 'rxjs/operators';
const observableWithDebugging = observable.pipe(
tap(value => console.log('Emitted Value: ', value))
);
Error Catching
Errors can propagate through the chain of observables. Using comprehensive error handling with catchError
and logging can ensure that errors are manageable and trackable.
Dev Tools
Libraries like the Redux DevTools enable tracking and monitoring of observables and their state changes, providing insight into how state flows within an application.
Conclusion
Observables represent a profound shift in how JavaScript applications can manage asynchronous events and data streams. They form the backbone of reactive programming in the JavaScript ecosystem, allowing developers to build scalable, maintainable, and performative applications. As with any powerful tool, understanding the strengths and weaknesses of observables will empower developers to tackle complex problems adeptly.
Further Reading and Resources
- RxJS Official Documentation
- Reactive Extensions
- Building Applications with RxJS
- Functional Programming in JavaScript
By comprehensively understanding observables, senior developers can enhance their skillset and design better systems centered around reactive programming principles.