Unlocking boto3 Events Subscriptions: Undocumented DynamoDB Extensions
Introduction If you’ve ever wished boto3 did just a bit more out of the box for DynamoDB, you’re in luck. In this post, we’ll explore boto3 event subscriptions, a little-known extension point that lets you inject custom behavior into DynamoDB calls. You’ll learn how to tap into the request/response lifecycle without forking or monkey-patching. Plus, we’ll point you to the library which makes life easier. What Is boto3 Events Subscriptions? At its core, boto3’s event system “allows users to register a function to a specific event” in the AWS API call lifecycle.¹ Instead of subclassing or forking the SDK, you simply hook into named stages like provide-client-params, before-call, or after-call. Handlers run in registration order, can tweak parameters or short-circuit requests, and—yes—they work just as well for DynamoDB as they do for S3. Why It Matters Custom defaults: Need a capacity setting or default serialization? Inject it automatically. Logging & metrics: Time your scans or log conditional checks—no external wrapper needed. Serialization tweaks: Encode/decode attributes in a bespoke format (think custom date strings). Non-invasive: You’re not monkey-patching or maintaining a separate client—handlers live alongside your code. Real Example: Injecting a Default TableName # Minimal subscription for DynamoDB Scan from boto3 import client def default_table(params, **kwargs): params.setdefault('TableName', 'MyDefaultTable') dynamo = client('dynamodb') dynamo.meta.events.register( 'provide-client-params.dynamodb.Scan', default_table ) # Later... dynamo.scan() # Uses 'MyDefaultTable' automatically Why it matters: This 3-line tweak saves you from repeating TableName= everywhere, and still fails fast if you really mean a different table. Pros and Cons of Each Approach Approach: **Event Subscriptions **Pros: Zero-fork, aligns with boto3 Hierarchical & wildcard support Cons: Underdocumented Signature needs **kwargs Approach: Custom Wrapper Library Pros: Abstracts complexity Shareable code Cons: Extra dependency May lag behind boto3 updates Approach: Monkey-patching Methods Pros: Full control Cons: Brittle, breaks easily Best Practices Use unique IDs when registering so you can unregister later. Scope narrowly: register to provide-client-params.dynamodb.GetItem, not the entire service, unless you really mean “every operation.” Keep handlers idempotent: they may run multiple times in complex call flows. Test in isolation: stub DynamoDB with botocore’s stubber or local DynamoDB. Document your hooks: future you (or your team) will thank you. Common Mistakes to Avoid Misusing wildcards: 'provide-client-params.dynamodb.*Item' won’t match GetItem Ignoring isolation: each client/resource has its own event system—registering on one won’t affect another Introducing botowrap Rather than reinventing the wheel, check out botowrap on PyPI. It bundles common DynamoDB event subscriptions—automatic serialization, pagination helpers, timestamps, and more—into a clean, extensible framework. Install with: pip install botowrap Then set up your enhanced boto3 client in just a few lines: import boto3 from botowrap.core import ExtensionManager from botowrap.extensions.dynamodb import DynamoDBExtension, DynamoDBConfig # Configure and register the DynamoDB extension mgr = ExtensionManager() ddb_config = DynamoDBConfig( max_retries=5, # Auto-retry on throttling log_consumed=True, # Log capacity consumption add_pagination=True, # Add scan_all and query_all helpers add_timestamps=True # Auto-add CreatedAt/UpdatedAt timestamps ) mgr.register(DynamoDBExtension(ddb_config)) mgr.bootstrap() # Now create clients as usual - they're automatically enhanced ddb = boto3.client('dynamodb') # Use with native Python types - no more TypeSerializer! ddb.put_item( TableName='Users', Item={ 'UserId': 'user123', 'Name': 'Alice', 'Age': 30, 'Active': True, 'Tags': ['developer', 'admin'] } ) # Get results as Python objects - automatic deserialization response = ddb.get_item(TableName='Users', Key={'UserId': 'user123'}) print(response['Item']) # A Python dict with native types # Use pagination helpers to get all results in one call all_users = ddb.scan_all(TableName='Users') print(f"Found {len(all_users['Items'])} users") Conclusion boto3 event subscriptions are a powerful, if underdocumented, way to extend DynamoDB clients without hacks. Whether you need default behaviors, custom serialization, or fine-grained logging, events have you covered. Next up: deep-dive into advanced serializers—stay tuned! Further Reading Boto3 Events Guide: https://boto3.amazonaws.com/v1/documentation/api/latest/guide/events.html Botocore Events: https://botocore.amazonaws.com/v1/documentation/api/latest/reference/events.html bo
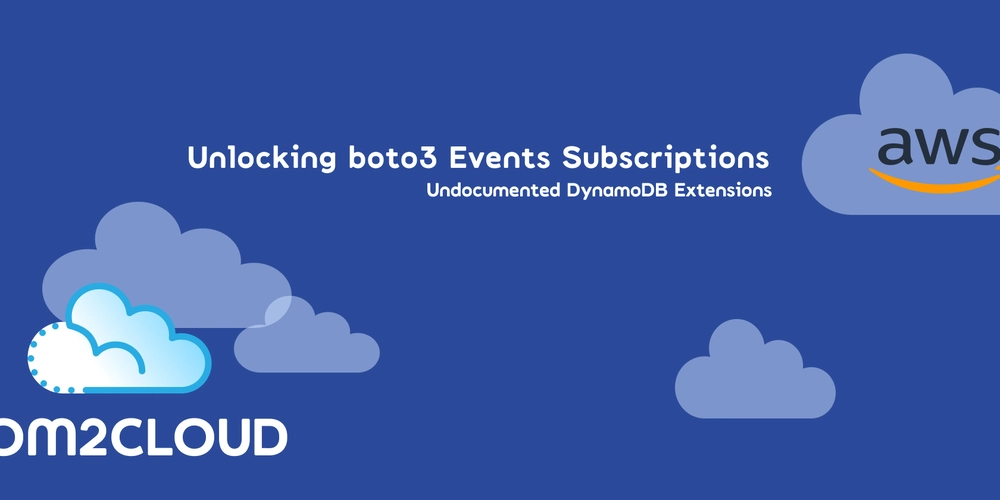
Introduction
If you’ve ever wished boto3 did just a bit more out of the box for DynamoDB, you’re in luck. In this post, we’ll explore boto3 event subscriptions, a little-known extension point that lets you inject custom behavior into DynamoDB calls. You’ll learn how to tap into the request/response lifecycle without forking or monkey-patching. Plus, we’ll point you to the library which makes life easier.
What Is boto3 Events Subscriptions?
At its core, boto3’s event system “allows users to register a function to a specific event” in the AWS API call lifecycle.¹ Instead of subclassing or forking the SDK, you simply hook into named stages like provide-client-params, before-call, or after-call. Handlers run in registration order, can tweak parameters or short-circuit requests, and—yes—they work just as well for DynamoDB as they do for S3.
Why It Matters
- Custom defaults: Need a capacity setting or default serialization? Inject it automatically.
- Logging & metrics: Time your scans or log conditional checks—no external wrapper needed.
- Serialization tweaks: Encode/decode attributes in a bespoke format (think custom date strings).
- Non-invasive: You’re not monkey-patching or maintaining a separate client—handlers live alongside your code.
Real Example: Injecting a Default TableName
# Minimal subscription for DynamoDB Scan
from boto3 import client
def default_table(params, **kwargs):
params.setdefault('TableName', 'MyDefaultTable')
dynamo = client('dynamodb')
dynamo.meta.events.register(
'provide-client-params.dynamodb.Scan',
default_table
)
# Later...
dynamo.scan() # Uses 'MyDefaultTable' automatically
Why it matters: This 3-line tweak saves you from repeating TableName= everywhere, and still fails fast if you really mean a different table.
Pros and Cons of Each Approach
Approach: **Event Subscriptions
**Pros:
- Zero-fork, aligns with boto3
- Hierarchical & wildcard support
Cons:
- Underdocumented
- Signature needs **kwargs
Approach: Custom Wrapper Library
Pros:
- Abstracts complexity
- Shareable code
Cons:
- Extra dependency
- May lag behind boto3 updates
Approach: Monkey-patching Methods
Pros:
- Full control
Cons:
- Brittle, breaks easily
Best Practices
- Use unique IDs when registering so you can unregister later.
- Scope narrowly: register to
provide-client-params.dynamodb.GetItem
, not the entire service, unless you really mean “every operation.” - Keep handlers idempotent: they may run multiple times in complex call flows.
- Test in isolation: stub DynamoDB with botocore’s stubber or local DynamoDB.
- Document your hooks: future you (or your team) will thank you.
Common Mistakes to Avoid
- Misusing wildcards:
'provide-client-params.dynamodb.*Item'
won’t match GetItem - Ignoring isolation: each client/resource has its own event system—registering on one won’t affect another
Introducing botowrap
Rather than reinventing the wheel, check out botowrap on PyPI. It bundles common DynamoDB event subscriptions—automatic serialization, pagination helpers, timestamps, and more—into a clean, extensible framework. Install with:
pip install botowrap
Then set up your enhanced boto3 client in just a few lines:
import boto3
from botowrap.core import ExtensionManager
from botowrap.extensions.dynamodb import DynamoDBExtension, DynamoDBConfig
# Configure and register the DynamoDB extension
mgr = ExtensionManager()
ddb_config = DynamoDBConfig(
max_retries=5, # Auto-retry on throttling
log_consumed=True, # Log capacity consumption
add_pagination=True, # Add scan_all and query_all helpers
add_timestamps=True # Auto-add CreatedAt/UpdatedAt timestamps
)
mgr.register(DynamoDBExtension(ddb_config))
mgr.bootstrap()
# Now create clients as usual - they're automatically enhanced
ddb = boto3.client('dynamodb')
# Use with native Python types - no more TypeSerializer!
ddb.put_item(
TableName='Users',
Item={
'UserId': 'user123',
'Name': 'Alice',
'Age': 30,
'Active': True,
'Tags': ['developer', 'admin']
}
)
# Get results as Python objects - automatic deserialization
response = ddb.get_item(TableName='Users', Key={'UserId': 'user123'})
print(response['Item']) # A Python dict with native types
# Use pagination helpers to get all results in one call
all_users = ddb.scan_all(TableName='Users')
print(f"Found {len(all_users['Items'])} users")
Conclusion
boto3 event subscriptions are a powerful, if underdocumented, way to extend DynamoDB clients without hacks. Whether you need default behaviors, custom serialization, or fine-grained logging, events have you covered. Next up: deep-dive into advanced serializers—stay tuned!
Further Reading
- Boto3 Events Guide: https://boto3.amazonaws.com/v1/documentation/api/latest/guide/events.html
- Botocore Events: https://botocore.amazonaws.com/v1/documentation/api/latest/reference/events.html
- botowrap on PyPI: https://pypi.org/project/com2cloud-botowrap/