Understanding Web Real-Time Communication(WebRTC)
Introduction GitHub WebRTC Buzzwords Explained STUN (Session Traversal Utilities for NAT) TURN (Traversal Using Relays around NAT) SDP (Session Description Protocol) WebSocket Offer/Answer NAT (Network Address Translation) ICE (Interactive Connectivity Establishment) Signaling Core Concepts of WebRTC 1. Signaling Server 2. Interactive Connectivity Establishment(ICE) Candidates 3. Session Description Protocol (SDP) The WebRTC Connection Process Security Considerations Best Practices Common Challenges and Solutions Conclusion Further Reading A Deep Dive into Peer-to-Peer Communication Introduction WebRTC is a powerful technology that enables real-time communication between browsers. It allows direct peer-to-peer connections for video, audio, and data sharing without the need for intermediate servers. In this article, we'll explore the core concepts of WebRTC and see how they're implemented in a Go-based WebRTC application. GitHub The repository used for this article if you want to follow through or connect https://github.com/Doc-Scripter/WebRTC.git WebRTC Buzzwords Explained Before diving into the technical details, let's break down the common WebRTC buzzwords you'll encounter: STUN (Session Traversal Utilities for NAT) Think of STUN as a "phone book" for your computer's public address When your computer is behind a router (NAT), it needs to know its public IP address STUN servers help your computer discover its public address Like asking "What's my public phone number?" to a directory service TURN (Traversal Using Relays around NAT) TURN is like a "mail forwarding service" for your data When direct peer-to-peer connection isn't possible, TURN servers relay your data Think of it as having a friend in the middle who passes messages between you and someone else More expensive to use than direct connections, but works when nothing else does SDP (Session Description Protocol) SDP is like a "contract" that describes how the communication will work It specifies details like: What type of media we're sending (video/audio) What codecs we're using Network parameters Similar to agreeing on a common language before starting a conversation WebSocket A persistent connection between your browser and server Unlike regular HTTP requests that are one-and-done, WebSocket stays open Like having a dedicated phone line instead of sending letters back and forth Used in WebRTC for signaling (coordinating the connection setup) Offer/Answer The process of establishing a connection between peers "Offer" is like saying "Hey, let's connect this way" "Answer" is like saying "Yes, that works for me" Similar to agreeing on how to communicate before starting the actual conversation NAT (Network Address Translation) NAT is like a receptionist at a building It allows multiple devices to share one public IP address Your computer has a private address (like an internal office number) NAT translates between private and public addresses Can make direct peer-to-peer connections tricky ICE (Interactive Connectivity Establishment) ICE is like a matchmaking service for network connections It gathers all possible ways to connect (local IP, public IP, TURN relays) Tries each method until it finds one that works Similar to trying different routes to reach a destination Signaling The process of coordinating the connection setup Like exchanging phone numbers before making a call Involves sharing connection information between peers Usually done through a signaling server using WebSocket These terms work together to create a complete WebRTC connection: STUN helps discover public addresses TURN provides relay when needed SDP describes how to communicate WebSocket enables signaling Offer/Answer establishes the connection NAT traversal (via STUN/TURN) ensures connectivity ICE finds the best connection path Core Concepts of WebRTC 1. Signaling Server Signaling is the process of coordinating communication between peers. Since WebRTC is peer-to-peer, peers need a way to discover each other and exchange connection information. This is where the signaling server comes in. In our implementation, we use WebSocket for signaling: // From handlers/server.go type SignalMessage struct { Type string `json:"type"` Data any `json:"data"` From string `json:"from,omitempty"` To string `json:"to,omitempty"` } func SignalingHandler(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Println("Upgrade error:", err) return } defer conn.Close() for { var msg SignalMessage err := conn.ReadJSON(&msg) if err != nil { log.Println("Read error:", err) break } // Handle di
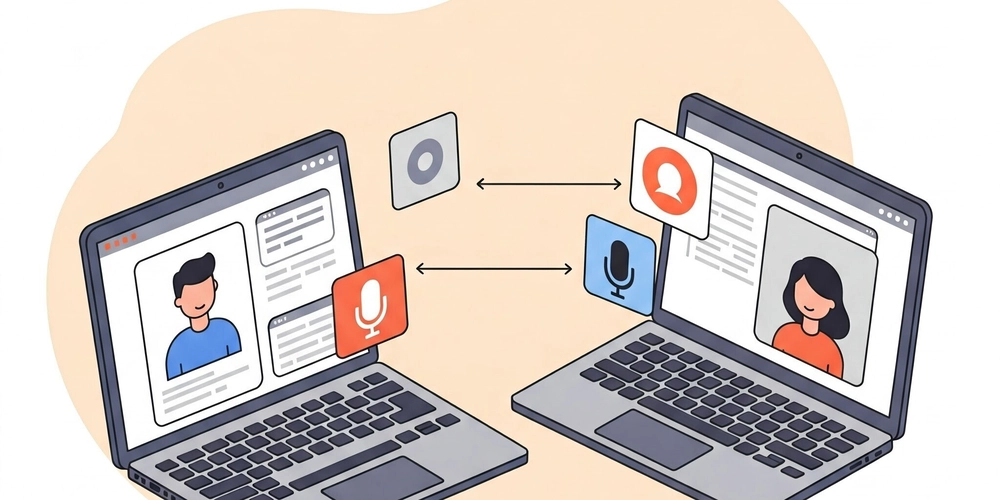
- Introduction
- GitHub
-
WebRTC Buzzwords Explained
- STUN (Session Traversal Utilities for NAT)
- TURN (Traversal Using Relays around NAT)
- SDP (Session Description Protocol)
- WebSocket
- Offer/Answer
- NAT (Network Address Translation)
- ICE (Interactive Connectivity Establishment)
- Signaling
-
Core Concepts of WebRTC
- 1. Signaling Server
- 2. Interactive Connectivity Establishment(ICE) Candidates
- 3. Session Description Protocol (SDP)
- The WebRTC Connection Process
- Security Considerations
- Best Practices
- Common Challenges and Solutions
- Conclusion
- Further Reading
A Deep Dive into Peer-to-Peer Communication
Introduction
WebRTC is a powerful technology that enables real-time communication between browsers. It allows direct peer-to-peer connections for video, audio, and data sharing without the need for intermediate servers. In this article, we'll explore the core concepts of WebRTC and see how they're implemented in a Go-based WebRTC application.
GitHub
The repository used for this article if you want to follow through or connect
WebRTC Buzzwords Explained
Before diving into the technical details, let's break down the common WebRTC buzzwords you'll encounter:
STUN (Session Traversal Utilities for NAT)
- Think of STUN as a "phone book" for your computer's public address
- When your computer is behind a router (NAT), it needs to know its public IP address
- STUN servers help your computer discover its public address
- Like asking "What's my public phone number?" to a directory service
TURN (Traversal Using Relays around NAT)
- TURN is like a "mail forwarding service" for your data
- When direct peer-to-peer connection isn't possible, TURN servers relay your data
- Think of it as having a friend in the middle who passes messages between you and someone else
- More expensive to use than direct connections, but works when nothing else does
SDP (Session Description Protocol)
- SDP is like a "contract" that describes how the communication will work
- It specifies details like:
- What type of media we're sending (video/audio)
- What codecs we're using
- Network parameters
- Similar to agreeing on a common language before starting a conversation
WebSocket
- A persistent connection between your browser and server
- Unlike regular HTTP requests that are one-and-done, WebSocket stays open
- Like having a dedicated phone line instead of sending letters back and forth
- Used in WebRTC for signaling (coordinating the connection setup)
Offer/Answer
- The process of establishing a connection between peers
- "Offer" is like saying "Hey, let's connect this way"
- "Answer" is like saying "Yes, that works for me"
- Similar to agreeing on how to communicate before starting the actual conversation
NAT (Network Address Translation)
- NAT is like a receptionist at a building
- It allows multiple devices to share one public IP address
- Your computer has a private address (like an internal office number)
- NAT translates between private and public addresses
- Can make direct peer-to-peer connections tricky
ICE (Interactive Connectivity Establishment)
- ICE is like a matchmaking service for network connections
- It gathers all possible ways to connect (local IP, public IP, TURN relays)
- Tries each method until it finds one that works
- Similar to trying different routes to reach a destination
Signaling
- The process of coordinating the connection setup
- Like exchanging phone numbers before making a call
- Involves sharing connection information between peers
- Usually done through a signaling server using WebSocket
These terms work together to create a complete WebRTC connection:
- STUN helps discover public addresses
- TURN provides relay when needed
- SDP describes how to communicate
- WebSocket enables signaling
- Offer/Answer establishes the connection
- NAT traversal (via STUN/TURN) ensures connectivity
- ICE finds the best connection path
Core Concepts of WebRTC
1. Signaling Server
Signaling is the process of coordinating communication between peers. Since WebRTC is peer-to-peer, peers need a way to discover each other and exchange connection information. This is where the signaling server comes in.
In our implementation, we use WebSocket for signaling:
// From handlers/server.go
type SignalMessage struct {
Type string `json:"type"`
Data any `json:"data"`
From string `json:"from,omitempty"`
To string `json:"to,omitempty"`
}
func SignalingHandler(w http.ResponseWriter, r *http.Request) {
conn, err := upgrader.Upgrade(w, r, nil)
if err != nil {
log.Println("Upgrade error:", err)
return
}
defer conn.Close()
for {
var msg SignalMessage
err := conn.ReadJSON(&msg)
if err != nil {
log.Println("Read error:", err)
break
}
// Handle different types of signaling messages
switch msg.Type {
case "offer":
log.Printf("Received offer: %+v", msg)
case "answer":
log.Printf("Received answer: %+v", msg)
case "ice-candidate":
log.Printf("Received ICE candidate via WebSocket: %+v", msg)
}
}
}
2. Interactive Connectivity Establishment(ICE) Candidates
ICE candidates are network addresses that can be used to establish a connection between peers. They can be local IP addresses, public IP addresses, or relayed addresses through TURN servers.
Here's how we handle ICE candidates in our implementation:
// From handlers/server.go
type ICECandidate struct {
Candidate string `json:"candidate"`
SDPMid string `json:"sdpMid"`
SDPMLineIndex int `json:"sdpMLineIndex"`
}
func HandleICECandidate(w http.ResponseWriter, r *http.Request) {
var candidate ICECandidate
if err := json.NewDecoder(r.Body).Decode(&candidate); err != nil {
http.Error(w, "Invalid ICE candidate", http.StatusBadRequest)
return
}
log.Printf("Received ICE candidate: %+v", candidate)
w.WriteHeader(http.StatusOK)
}
3. Session Description Protocol (SDP)
SDP is used to describe the parameters of the media connection between peers. It includes information about:
- Media types (audio/video)
- Codecs
- Network parameters
- Security parameters
In our implementation, SDP is exchanged through the signaling server:
// From static/scripts.js
async function createOffer() {
const peerConnection = new RTCPeerConnection(configuration);
// Add local media stream
const stream = await navigator.mediaDevices.getUserMedia({ audio: true, video: true });
stream.getTracks().forEach(track => peerConnection.addTrack(track, stream));
// Create and send offer
const offer = await peerConnection.createOffer();
await peerConnection.setLocalDescription(offer);
// Send offer through signaling server
ws.send(JSON.stringify({
type: 'offer',
data: offer
}));
}
The WebRTC Connection Process
-
Initial Setup
- Both peers connect to the signaling server via WebSocket
- Each peer creates an RTCPeerConnection object
-
Offer/Answer Exchange
- Initiator creates an offer using
createOffer()
- Offer is sent to the signaling server
- Signaling server forwards the offer to the other peer
- Receiver creates an answer using
createAnswer()
- Answer is sent back through the signaling server
- Initiator creates an offer using
-
ICE Candidate Exchange
- Both peers gather ICE candidates
- Candidates are exchanged through the signaling server
- Peers add received candidates to their RTCPeerConnection
-
Connection Establishment
- Once ICE candidates are exchanged, the connection is established
- Media streams begin flowing directly between peers
Security Considerations
WebRTC includes several security features:
- DTLS (Datagram Transport Layer Security) for data encryption
- SRTP (Secure Real-time Transport Protocol) for media encryption
- Mandatory encryption for all WebRTC components
Best Practices
- Error Handling
peerConnection.onicecandidateerror = (event) => {
console.error('ICE candidate error:', event);
};
- Connection State Monitoring
peerConnection.onconnectionstatechange = () => {
console.log('Connection state:', peerConnection.connectionState);
};
- Resource Cleanup
function cleanup() {
peerConnection.close();
ws.close();
}
Common Challenges and Solutions
-
NAT Traversal
- Use STUN/TURN servers to handle NAT traversal
- Implement ICE candidate gathering and exchange
-
Connection Stability
- Monitor connection state
- Implement reconnection logic
- Handle network changes
-
Media Quality
- Implement bandwidth adaptation
- Use appropriate codecs
- Monitor media quality metrics
Conclusion
WebRTC is a powerful technology that enables real-time communication in web browsers. Understanding its core concepts - signaling, ICE candidates, and SDP - is crucial for building robust WebRTC applications. Our Go implementation demonstrates these concepts in practice, providing a foundation for building more complex WebRTC applications.