Understanding the AngularJS Application Creation Workflow
AngularJS, once the go-to framework for building dynamic single-page applications (SPAs), still holds significance in legacy projects and systems today. While newer frameworks have taken the spotlight, AngularJS remains relevant for developers maintaining or upgrading older applications. If you're diving into AngularJS or managing an existing project, it’s essential to understand the AngularJS coding process and how its application creation workflow functions from start to finish. What Is AngularJS? AngularJS is a structural JavaScript framework maintained by Google. It lets developers use HTML as the template language and extend HTML’s syntax to express application components clearly and succinctly. AngularJS’s data binding and dependency injection eliminate much of the code you would otherwise write. The AngularJS Coding Process: Step-by-Step Workflow Building an AngularJS application involves more than just writing JavaScript. It requires a clear understanding of how the different components interact, and how to structure your code for scalability and performance. Below is a typical workflow developers follow during the AngularJS application creation process. 1. Planning the Application Structure Before writing any code, developers outline the application's purpose, required features, and user experience flow. This includes: Defining the module structure Identifying necessary components (controllers, services, directives) Mapping data models and APIs Planning is crucial to ensuring maintainability, especially when working on enterprise-level applications. 2. Setting Up the Environment The next step involves preparing the development environment: Install Node.js and npm (if needed for tooling) Set up a project directory structure Include AngularJS via CDN or by downloading it locally Create core folders like /app, /controllers, /services, and /views A solid structure makes the AngularJS coding process more organized and less error-prone. 3. Creating the Main Module Every AngularJS application starts with a root module. This is done using the angular.module() method: javascript Copy Edit var app = angular.module('myApp', []); This module acts as a container for various parts of the app like controllers, services, filters, and directives. 4. Building Controllers and Views Controllers in AngularJS are JavaScript functions bound to a particular scope. They control the data and logic behind the views: javascript Copy Edit app.controller('MainCtrl', function($scope) { $scope.message = "Welcome to AngularJS!"; }); Then, bind this controller to an HTML view: html Copy Edit {{ message }} This combination of controller and view is the foundation of the MVC (Model-View-Controller) pattern in AngularJS. 5. Implementing Services and Factories Services are reusable components that handle business logic and data communication, such as API calls: javascript Copy Edit app.service('DataService', function($http) { this.getData = function() { return $http.get('/api/data'); }; }); Using services helps separate logic from the controller, keeping the codebase clean and modular. 6. Using Directives for Custom Behavior Directives in AngularJS let you invent new HTML syntax specific to your application. For example: javascript Copy Edit app.directive('customGreeting', function() { return { template: 'Hello, this is a custom directive!' }; }); Custom directives enhance reusability and encapsulate functionality within a component-like structure. 7. Routing and Navigation To create a seamless single-page application, AngularJS uses $routeProvider for routing: javascript Copy Edit app.config(function($routeProvider) { $routeProvider .when('/', { templateUrl: 'home.html', controller: 'HomeCtrl' }) .when('/about', { templateUrl: 'about.html', controller: 'AboutCtrl' }); }); Routing helps manage different views without reloading the page, offering a smooth user experience. 8. Form Handling and Validation AngularJS simplifies form handling through directives like ng-model, ng-submit, and ng-required. Real-time validation provides instant feedback to users, enhancing UX and reducing server load. html Copy Edit Name is required. 9. Testing and Debugging AngularJS supports unit testing through tools like Jasmine and Karma. Using dependency injection, components can be easily tested in isolation. Debugging is also straightforward with browser dev tools and the ng-inspector extension. 10. Build and Deployment Process Although AngularJS apps are written in plain JavaScript, they often go through a build process before deployment. This may include: Minifying and bundling files with tools like Gulp or Grunt Running tests Compressing images Deploying to production servers or platforms Modern deployment can integrate CI/CD pipelines to automate these steps, ensuring a smooth release cycle. Final Thoughts Understanding the AngularJS
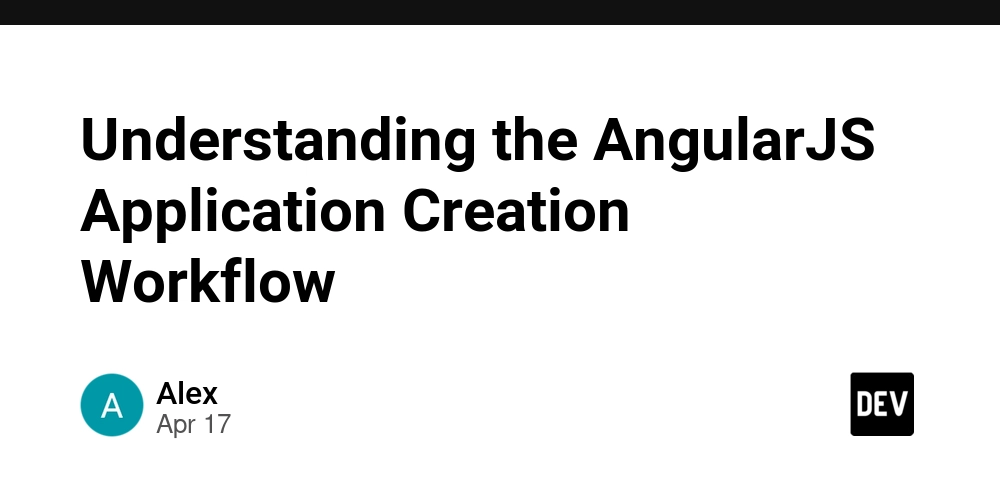
AngularJS, once the go-to framework for building dynamic single-page applications (SPAs), still holds significance in legacy projects and systems today. While newer frameworks have taken the spotlight, AngularJS remains relevant for developers maintaining or upgrading older applications. If you're diving into AngularJS or managing an existing project, it’s essential to understand the AngularJS coding process and how its application creation workflow functions from start to finish.
What Is AngularJS?
AngularJS is a structural JavaScript framework maintained by Google. It lets developers use HTML as the template language and extend HTML’s syntax to express application components clearly and succinctly. AngularJS’s data binding and dependency injection eliminate much of the code you would otherwise write.
The AngularJS Coding Process: Step-by-Step Workflow
Building an AngularJS application involves more than just writing JavaScript. It requires a clear understanding of how the different components interact, and how to structure your code for scalability and performance. Below is a typical workflow developers follow during the AngularJS application creation process.
1. Planning the Application Structure
Before writing any code, developers outline the application's purpose, required features, and user experience flow. This includes:
Defining the module structure
Identifying necessary components (controllers, services, directives)
Mapping data models and APIs
Planning is crucial to ensuring maintainability, especially when working on enterprise-level applications.
2. Setting Up the Environment
The next step involves preparing the development environment:
Install Node.js and npm (if needed for tooling)
Set up a project directory structure
Include AngularJS via CDN or by downloading it locally
Create core folders like /app, /controllers, /services, and /views
A solid structure makes the AngularJS coding process more organized and less error-prone.
3. Creating the Main Module
Every AngularJS application starts with a root module. This is done using the angular.module() method:
javascript
Copy
Edit
var app = angular.module('myApp', []);
This module acts as a container for various parts of the app like controllers, services, filters, and directives.
4. Building Controllers and Views
Controllers in AngularJS are JavaScript functions bound to a particular scope. They control the data and logic behind the views:
javascript
Copy
Edit
app.controller('MainCtrl', function($scope) {
$scope.message = "Welcome to AngularJS!";
});
Then, bind this controller to an HTML view:
html
Copy
Edit
{{ message }}
This combination of controller and view is the foundation of the MVC (Model-View-Controller) pattern in AngularJS.
5. Implementing Services and Factories
Services are reusable components that handle business logic and data communication, such as API calls:
javascript
Copy
Edit
app.service('DataService', function($http) {
this.getData = function() {
return $http.get('/api/data');
};
});
Using services helps separate logic from the controller, keeping the codebase clean and modular.
6. Using Directives for Custom Behavior
Directives in AngularJS let you invent new HTML syntax specific to your application. For example:
javascript
Copy
Edit
app.directive('customGreeting', function() {
return {
template: 'Hello, this is a custom directive!'
};
});
Custom directives enhance reusability and encapsulate functionality within a component-like structure.
7. Routing and Navigation
To create a seamless single-page application, AngularJS uses $routeProvider for routing:
javascript
Copy
Edit
app.config(function($routeProvider) {
$routeProvider
.when('/', {
templateUrl: 'home.html',
controller: 'HomeCtrl'
})
.when('/about', {
templateUrl: 'about.html',
controller: 'AboutCtrl'
});
});
Routing helps manage different views without reloading the page, offering a smooth user experience.
8. Form Handling and Validation
AngularJS simplifies form handling through directives like ng-model, ng-submit, and ng-required. Real-time validation provides instant feedback to users, enhancing UX and reducing server load.
html
Copy
Edit
Name is required.
9. Testing and Debugging
AngularJS supports unit testing through tools like Jasmine and Karma. Using dependency injection, components can be easily tested in isolation. Debugging is also straightforward with browser dev tools and the ng-inspector extension.
10. Build and Deployment Process
Although AngularJS apps are written in plain JavaScript, they often go through a build process before deployment. This may include:
Minifying and bundling files with tools like Gulp or Grunt
Running tests
Compressing images
Deploying to production servers or platforms
Modern deployment can integrate CI/CD pipelines to automate these steps, ensuring a smooth release cycle.
Final Thoughts
Understanding the AngularJS coding process is key to building functional, maintainable web applications. While AngularJS might no longer be the latest and greatest, many businesses continue to rely on it. Mastering the AngularJS application creation workflow—from planning and coding to building and deployment—ensures that developers can maintain existing apps efficiently or even build new ones where appropriate.
If you're managing a legacy AngularJS project, keeping these workflow steps in mind can make your job easier and your application more robust.