Understanding JavaScript Prototypes and Classes
Table of Contents Introduction What are Prototypes in JavaScript? What are Classes in JavaScript? Differences Between Prototypes and Classes What are JavaScript Prototypes? JavaScript objects have a hidden internal property called [[Prototype]], which is a reference to another object. This is known as the prototype chain, and it allows objects to inherit properties and methods from other objects. javascript function Person(name) { this.name = name; } Person.prototype.greet = function() { console.log(`Hello, my name is ${this.name}`); }; const john = new Person('John'); john.greet(); // "Hello, my name is John"
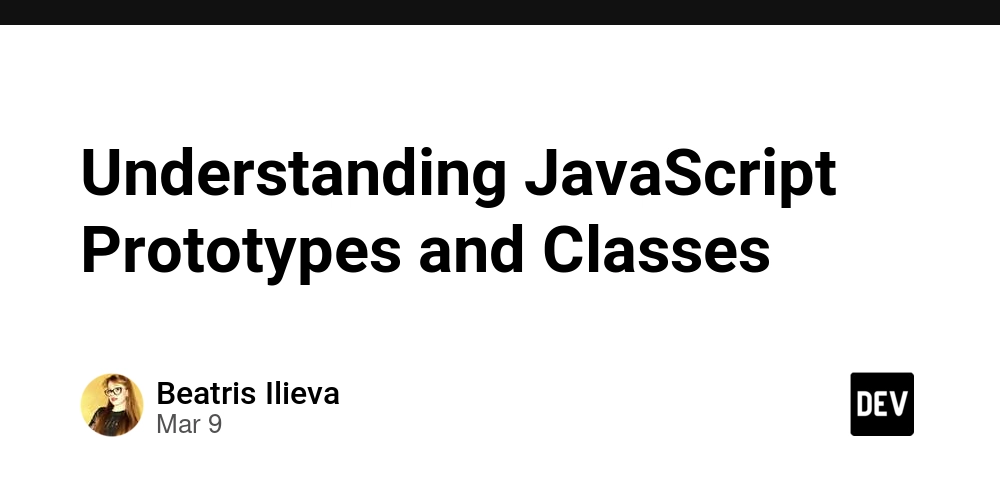
Table of Contents
- Introduction
- What are Prototypes in JavaScript?
- What are Classes in JavaScript?
- Differences Between Prototypes and Classes
What are JavaScript Prototypes?
JavaScript objects have a hidden internal property called [[Prototype]]
, which is a reference to another object. This is known as the prototype chain, and it allows objects to inherit properties and methods from other objects.
javascript
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}`);
};
const john = new Person('John');
john.greet(); // "Hello, my name is John"