Understanding Dynamic Programming
Dynamic Programming (DP) is a technique used to solve problems by breaking them into smaller subproblems and storing the results to avoid redundant work. One of the most basic examples is calculating the Fibonacci numbers. Java Code Example: Fibonacci Using Memoization java // Memoization (Top-down approach) import java.util.Arrays; class Fibonacci { static int fib(int n, int[] memo) { if (n
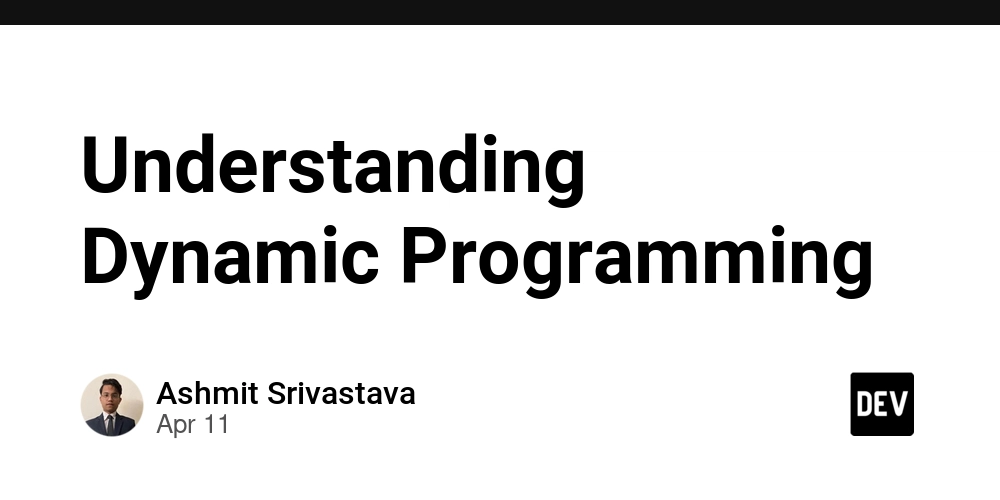
Dynamic Programming (DP) is a technique used to solve problems by breaking them into smaller subproblems and storing the results to avoid redundant work.
One of the most basic examples is calculating the Fibonacci numbers.
Java Code Example: Fibonacci Using Memoization
java
// Memoization (Top-down approach)
import java.util.Arrays;
class Fibonacci {
static int fib(int n, int[] memo) {
if (n <= 1) return n;
if (memo[n] != -1) return memo[n];
memo[n] = fib(n - 1, memo) + fib(n - 2, memo);
return memo[n];
}
public static void main(String[] args) {
int n = 10;
int[] memo = new int[n + 1];
Arrays.fill(memo, -1);
System.out.println("Fibonacci of " + n + " is " + fib(n, memo));
}
}