Understanding Angular Rendering, Re-rendering, and Change Detection for Optimal Performance
Introduction If you’re transitioning from React to Angular or starting with Angular as first step, understanding Angular’s rendering process, change detection mechanism, and re-rendering strategy is crucial for writing efficient and high-performance applications. Unlike React, where the virtual DOM helps optimize the re-render process, Angular’s change detection mechanism checks every component to ensure the UI reflects the latest data. This article will break down the concepts of rendering, change detection, and performance optimization in Angular, answering the questions you might have along the way. Understanding Angular Rendering and Re-rendering How Does Angular Render and Re-render Components? Angular, unlike React, uses a real DOM and checks for changes during change detection. Let's break down the process: Initial Rendering: When a component is created for the first time, Angular processes the component’s template and binds the data from the component to the template. Change Detection: Once the component is initialized, Angular listens for changes in the data. When any data-bound property changes, Angular triggers the change detection process, checking the whole component tree from the root. Re-rendering: When data in a component changes, Angular goes through the change detection cycle again. Unlike React, which uses a virtual DOM, Angular re-renders the DOM directly. This brings up an interesting question: If change detection checks the whole component tree, does Angular re-render everything even if only a small part of the data changes? Do Angular Re-renders Everything or Just the Changed Part? Angular does indeed go through all bindings in the component, even if only a single property changes. This is less efficient compared to React’s virtual DOM diffing mechanism, where only the changed elements are re-rendered. However, Angular uses optimizations such as OnPush change detection strategy and TrackBy in *ngFor to reduce unnecessary checks. Let's dive deeper into these strategies. Exploring Angular's Change Detection Mechanism How Does Change Detection Work in Angular? Change detection in Angular follows a process where Angular traverses the component tree and checks each binding to see if it has changed. If any change is detected, Angular updates the DOM. The default strategy is CheckAlways, which checks all components in the component tree. But Angular allows you to optimize this using OnPush. What is OnPush? When using OnPush change detection, Angular only checks a component for changes when: One of its Input properties changes. An event is triggered (e.g., button click). An observable emits a new value. This can significantly improve performance in large applications by avoiding unnecessary checks on components that haven’t changed. @Component({ selector: 'app-my-component', changeDetection: ChangeDetectionStrategy.OnPush, templateUrl: './my-component.component.html', }) export class MyComponent { @Input() data: any; } By using OnPush, you restrict the component to be checked only when a relevant change occurs, rather than checking it on every cycle. Why Does Angular Check All Components for Changes? You might wonder, Why does Angular check the entire component tree? Can it just check the component where the change happened? Angular’s default change detection works by traversing the whole component tree to check for any data changes. This design ensures that all bindings are up to date, but can become inefficient, especially in large applications. Unlike React’s virtual DOM, Angular doesn’t maintain a previous DOM snapshot, and so it doesn't have an efficient diffing mechanism that would allow it to detect changes at a granular level. The Challenge with Functions in Templates Angular has a two-way binding mechanism, which helps with reactivity. But one performance pitfall is calling functions directly in templates. Functions in templates are re-evaluated on every change detection cycle, which can hurt performance if not used properly. For example, calling a function in a template like this: {{ computeValue() }} Could cause the function to run repeatedly, making it inefficient. To avoid this, use getters or Signals (in Angular 16.2+) to track state more efficiently. get computedValue(): number { return this.data * 10; } This way, the value is calculated once, and Angular can optimize re-renders. Optimizing Angular for Better Performance To address performance concerns, let’s talk about strategies to optimize rendering and change detection in Angular. Use OnPush Change Detection As mentioned earlier, the OnPush strategy significantly reduces unnecessary re-renders. It tells Angular to check the component only when its inputs or observables change, or when an event is triggered. Use trackBy with *ngFor When using *ngFor, A
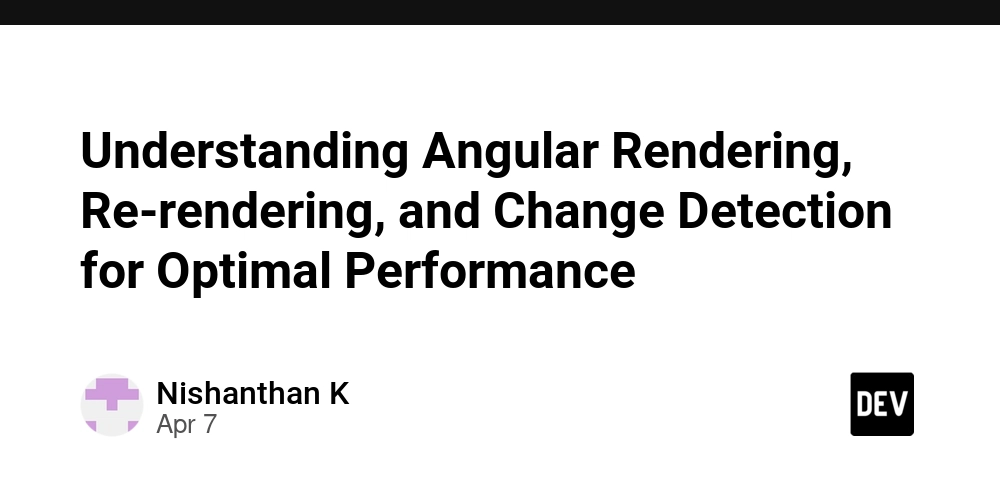
Introduction
If you’re transitioning from React to Angular or starting with Angular as first step, understanding Angular’s rendering process, change detection mechanism, and re-rendering strategy is crucial for writing efficient and high-performance applications. Unlike React, where the virtual DOM helps optimize the re-render process, Angular’s change detection mechanism checks every component to ensure the UI reflects the latest data. This article will break down the concepts of rendering, change detection, and performance optimization in Angular, answering the questions you might have along the way.
Understanding Angular Rendering and Re-rendering
How Does Angular Render and Re-render Components?
Angular, unlike React, uses a real DOM and checks for changes during change detection. Let's break down the process:
Initial Rendering: When a component is created for the first time, Angular processes the component’s template and binds the data from the component to the template.
Change Detection: Once the component is initialized, Angular listens for changes in the data. When any data-bound property changes, Angular triggers the change detection process, checking the whole component tree from the root.
Re-rendering: When data in a component changes, Angular goes through the change detection cycle again. Unlike React, which uses a virtual DOM, Angular re-renders the DOM directly.
This brings up an interesting question: If change detection checks the whole component tree, does Angular re-render everything even if only a small part of the data changes?
Do Angular Re-renders Everything or Just the Changed Part?
Angular does indeed go through all bindings in the component, even if only a single property changes. This is less efficient compared to React’s virtual DOM diffing mechanism, where only the changed elements are re-rendered.
However, Angular uses optimizations such as OnPush change detection strategy and TrackBy in *ngFor to reduce unnecessary checks. Let's dive deeper into these strategies.
Exploring Angular's Change Detection Mechanism
How Does Change Detection Work in Angular?
Change detection in Angular follows a process where Angular traverses the component tree and checks each binding to see if it has changed. If any change is detected, Angular updates the DOM.
The default strategy is CheckAlways, which checks all components in the component tree. But Angular allows you to optimize this using OnPush.
What is OnPush?
When using OnPush change detection, Angular only checks a component for changes when:
One of its Input properties changes.
An event is triggered (e.g., button click).
An observable emits a new value.
This can significantly improve performance in large applications by avoiding unnecessary checks on components that haven’t changed.
@Component({
selector: 'app-my-component',
changeDetection: ChangeDetectionStrategy.OnPush,
templateUrl: './my-component.component.html',
})
export class MyComponent {
@Input() data: any;
}
By using OnPush, you restrict the component to be checked only when a relevant change occurs, rather than checking it on every cycle.
Why Does Angular Check All Components for Changes?
You might wonder, Why does Angular check the entire component tree? Can it just check the component where the change happened?
Angular’s default change detection works by traversing the whole component tree to check for any data changes. This design ensures that all bindings are up to date, but can become inefficient, especially in large applications.
Unlike React’s virtual DOM, Angular doesn’t maintain a previous DOM snapshot, and so it doesn't have an efficient diffing mechanism that would allow it to detect changes at a granular level.
The Challenge with Functions in Templates
Angular has a two-way binding mechanism, which helps with reactivity. But one performance pitfall is calling functions directly in templates. Functions in templates are re-evaluated on every change detection cycle, which can hurt performance if not used properly.
For example, calling a function in a template like this:
{{ computeValue() }}
Could cause the function to run repeatedly, making it inefficient. To avoid this, use getters or Signals (in Angular 16.2+) to track state more efficiently.
get computedValue(): number {
return this.data * 10;
}
This way, the value is calculated once, and Angular can optimize re-renders.
Optimizing Angular for Better Performance
To address performance concerns, let’s talk about strategies to optimize rendering and change detection in Angular.
- Use OnPush Change Detection
As mentioned earlier, the OnPush strategy significantly reduces unnecessary re-renders. It tells Angular to check the component only when its inputs or observables change, or when an event is triggered.
- Use trackBy with *ngFor
When using *ngFor, Angular creates new DOM elements for every item in the list. If the list is large, this can become inefficient. You can optimize the rendering of lists by using trackBy to track which items have changed, rather than recreating the entire DOM for the list every time.
{{ item.name }}
trackById(index: number, item: any): number {
return item.id;
}
- Avoid Functions in Templates
Instead of calling functions directly in the template, use getters or Signals (in Angular 16.2+) to keep state changes efficient and avoid recomputing values on every change detection cycle.
- Signals (Angular 16.2+):
Signals in Angular provide a more efficient way to manage state and trigger updates. With signals, Angular tracks the state and updates the DOM only when the value of the signal changes.
isA = signal(true);
isB = signal(false);
- Use ngOnChanges and Input Setters For efficient handling of input changes, Angular provides Input Setters and ngOnChanges. Instead of checking changes manually, use these lifecycle hooks to optimize reactivity.
@Input() set data(value: any) {
this._data = value;
}
You can use this instead of ngOnChanges to efficiently handle changes in input properties.
Conclusion
Understanding Angular’s rendering, re-rendering, and change detection mechanisms is key to building efficient applications. By using techniques like OnPush change detection, trackBy in *ngFor, avoiding functions in templates, and leveraging Signals (in Angular 16.2+), you can optimize your Angular applications for better performance.
Remember, while Angular doesn’t use a virtual DOM like React, it offers a powerful change detection system that you can fine-tune for optimal performance. Implement these strategies and take control of your app’s reactivity—leading to faster, smoother, and more efficient user experiences.