TS1378: Top-level 'await' expressions are only allowed when the
TS1378: Top-level 'await' expressions are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher. TypeScript is a programming language that builds on JavaScript by adding static type definitions. This means you can define the types of variables, function parameters, and object properties. Types are a way to define what kind of value a variable can hold, providing a structured approach to coding that can help catch errors early in the development process. For instance, here is a simple type definition for a variable: let greeting: string = "Hello, world!"; In this example, greeting is defined with a type of string, which means it can only hold string values. If you're eager to expand your knowledge in TypeScript or want to explore AI tools to assist your learning, consider subscribing to my blog or joining gpteach for coding insights! What is a Superset Language? A superset language is a programming language that extends another language by adding new features while maintaining compatibility with the original language. TypeScript is a superset of JavaScript, meaning every valid JavaScript code is also valid TypeScript code. TypeScript adds features like type definitions, interfaces, and enums, allowing developers to write more robust and maintainable code. The Error: TS1378 Explained Now let's turn our focus to the error message TS1378: Top-level 'await' expressions are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher. This error occurs when you try to use the await keyword at the top level of your code without the appropriate TypeScript configuration. Using await at the top level is a feature that allows you to pause execution until a Promise is resolved or rejected, simplifying asynchronous programming. Here is an example that will trigger this error: // top-level await example const data = await fetch('https://api.example.com/data'); console.log(data); Important to Know! To be able to use top-level await, you'll need to ensure your TypeScript configuration (typically in the tsconfig.json file) specifies that you are using a module format that supports top-level await: { "compilerOptions": { "module": "es2022", "target": "es2017" } } If your tsconfig.json does not include the correct settings, you will encounter TS1378. How to Fix TS1378 To fix the TS1378 error, follow these steps: Check the module option: Ensure it's set to one of the following: es2022, esnext, system, node16, or nodenext. Check the target option: Make sure it's set to es2017 or higher. Here’s how you can modify your tsconfig.json file: { "compilerOptions": { "module": "es2022", "target": "es2018" } } With this configuration, your top-level await should work without any issues! FAQs Q1: What is a module in TypeScript? A: A module is a file that contains code that is executed in strict mode. It can export and import variables, functions, and classes from other modules. Q2: Why do I need to set the target option? A: The target option specifies the version of JavaScript you want your TypeScript code to compile to. Using a newer version allows you to leverage newer JavaScript features, including top-level await. Q3: Can I use top-level await in older JavaScript versions? A: No, top-level await is not supported in older JavaScript versions. You must use at least ES2017 as the target. Important Things to Know Ensure your TypeScript version is up-to-date to support top-level await. Using top-level await can improve readability and flow of asynchronous code. You may need to adjust your build tools (like Babel or Webpack) if they are transforming your code. In conclusion, the TS1378 error highlights specific requirements in TypeScript regarding module and target settings for utilizing top-level await expressions. Ensure your configuration aligns with the requirements mentioned: TS1378: Top-level 'await' expressions are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher. By following the guidelines outlined in this article, you should be able to effectively resolve any related issues.
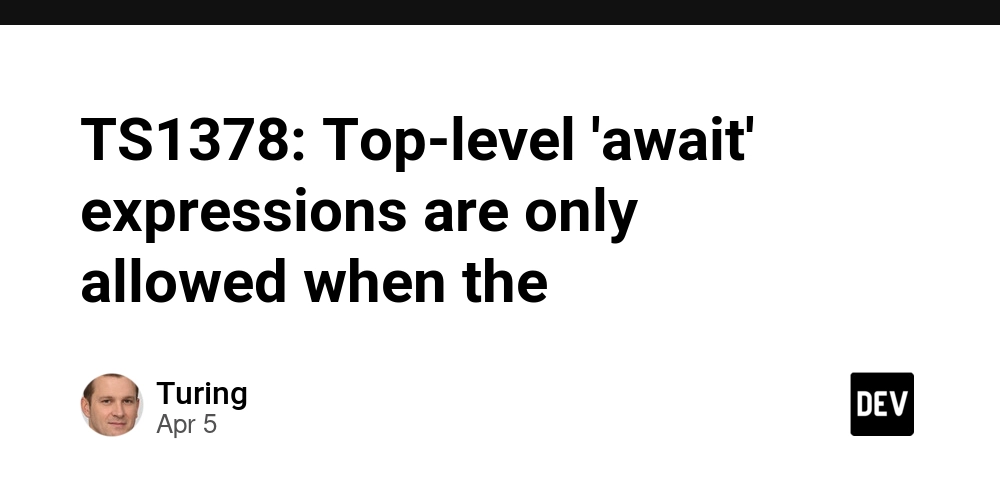
TS1378: Top-level 'await' expressions are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher.
TypeScript is a programming language that builds on JavaScript by adding static type definitions. This means you can define the types of variables, function parameters, and object properties. Types are a way to define what kind of value a variable can hold, providing a structured approach to coding that can help catch errors early in the development process.
For instance, here is a simple type definition for a variable:
let greeting: string = "Hello, world!";
In this example, greeting
is defined with a type of string
, which means it can only hold string values.
If you're eager to expand your knowledge in TypeScript or want to explore AI tools to assist your learning, consider subscribing to my blog or joining gpteach for coding insights!
What is a Superset Language?
A superset language is a programming language that extends another language by adding new features while maintaining compatibility with the original language. TypeScript is a superset of JavaScript, meaning every valid JavaScript code is also valid TypeScript code. TypeScript adds features like type definitions, interfaces, and enums, allowing developers to write more robust and maintainable code.
The Error: TS1378 Explained
Now let's turn our focus to the error message TS1378: Top-level 'await' expressions are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher.
This error occurs when you try to use the await
keyword at the top level of your code without the appropriate TypeScript configuration. Using await
at the top level is a feature that allows you to pause execution until a Promise is resolved or rejected, simplifying asynchronous programming.
Here is an example that will trigger this error:
// top-level await example
const data = await fetch('https://api.example.com/data');
console.log(data);
Important to Know!
To be able to use top-level await
, you'll need to ensure your TypeScript configuration (typically in the tsconfig.json
file) specifies that you are using a module format that supports top-level await:
{
"compilerOptions": {
"module": "es2022",
"target": "es2017"
}
}
If your tsconfig.json
does not include the correct settings, you will encounter TS1378.
How to Fix TS1378
To fix the TS1378 error, follow these steps:
-
Check the module option: Ensure it's set to one of the following:
es2022
,esnext
,system
,node16
, ornodenext
. -
Check the target option: Make sure it's set to
es2017
or higher.
Here’s how you can modify your tsconfig.json
file:
{
"compilerOptions": {
"module": "es2022",
"target": "es2018"
}
}
With this configuration, your top-level await should work without any issues!
FAQs
Q1: What is a module in TypeScript?
A: A module is a file that contains code that is executed in strict mode. It can export and import variables, functions, and classes from other modules.
Q2: Why do I need to set the target option?
A: The target option specifies the version of JavaScript you want your TypeScript code to compile to. Using a newer version allows you to leverage newer JavaScript features, including top-level await.
Q3: Can I use top-level await in older JavaScript versions?
A: No, top-level await is not supported in older JavaScript versions. You must use at least ES2017 as the target.
Important Things to Know
- Ensure your TypeScript version is up-to-date to support top-level await.
- Using top-level await can improve readability and flow of asynchronous code.
- You may need to adjust your build tools (like Babel or Webpack) if they are transforming your code.
In conclusion, the TS1378 error highlights specific requirements in TypeScript regarding module and target settings for utilizing top-level await expressions. Ensure your configuration aligns with the requirements mentioned: TS1378: Top-level 'await' expressions are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher. By following the guidelines outlined in this article, you should be able to effectively resolve any related issues.