ToDtype in PyTorch (2)
Buy Me a Coffee☕ *Memos: My post explains ToDtype() about scale=True. My post explains how to convert and scale a PIL Image to an Image in PyTorch. My post explains Compose(). My post explains ToImage(). My post explains ToTensor(). My post explains PILToTensor(). My post explains ToPILImage(). My post explains OxfordIIITPet(). ToDtype() can set a dtype to an Image, Video or tensor and scale its values as shown below. *It's about scale=False: from torchvision.datasets import OxfordIIITPet from torchvision.transforms.v2 import ToImage, ToDtype import torch import numpy as np PILImage_data = OxfordIIITPet( root="data", transform=None ) Image_data = OxfordIIITPet( root="data", transform=ToImage() ) td = ToDtype(dtype=torch.float32, scale=False) td(PILImage_data[0]) # (, 0) list(td(PILImage_data[0][0]).getdata()) # [(37, 20, 12), # (35, 18, 10), # (36, 19, 11), # (36, 19, 11), # (37, 18, 11), # ...] td(Image_data[0]) # (Image([[[37., 35., 36., ..., 247., 249., 249.], # [35., 35., 37., ..., 246., 248., 249.], # ..., # [28., 28., 27., ..., 59., 65., 76.]], # [[20., 18., 19., ..., 248., 248., 248.], # [18., 18., 20., ..., 247., 247., 248.], # ..., # [27., 27., 27., ..., 94., 106., 117.]], # [[12., 10., 11., ..., 253., 253., 253.], # [10., 10., 12., ..., 251., 252., 253.], # ..., # [35., 35., 35., ..., 214., 232., 223.]]],), 0) td(Image_data[0][0]) # Image([[[37, 35, 36, ..., 247, 249, 249], # [35, 35, 37, ..., 246, 248, 249], # ..., # [28, 28, 27, ..., 59, 65, 76]], # [[20, 18, 19, ..., 248, 248, 248], # [18, 18, 20, ..., 247, 247, 248], # ..., # [27, 27, 27, ..., 94, 106, 117]], # [[12, 10, 11, ..., 253, 253, 253], # [10, 10, 12, ..., 251, 252, 253], # ..., # [35, 35, 35, ..., 214, 232, 223]]],) td((torch.tensor(3), 0)) # int64 td((torch.tensor(3, dtype=torch.int64), 0)) # (tensor(3.), 0) td(torch.tensor(3)) # tensor(3.) td((torch.tensor([0, 1, 2, 3]), 0)) # (tensor([0., 1., 2., 3.]), 0) td(torch.tensor([0, 1, 2, 3])) # tensor([0., 1., 2., 3.]) td((torch.tensor([[0, 1, 2, 3]]), 0)) # (tensor([[0., 1., 2., 3.]]), 0) td(torch.tensor([[0, 1, 2, 3]])) # tensor([[0., 1., 2., 3.]]) td((torch.tensor([[[0, 1, 2, 3]]]), 0)) # (tensor([[[0., 1., 2., 3.]]]), 0) td(torch.tensor([[[0, 1, 2, 3]]])) # tensor([[[0., 1., 2., 3.]]]) td((torch.tensor([[[[0, 1, 2, 3]]]]), 0)) # (tensor([[[[0., 1., 2., 3.]]]]), 0) td(torch.tensor([[[[0, 1, 2, 3]]]])) # tensor([[[[0., 1., 2., 3.]]]]) td((torch.tensor([[[[[0, 1, 2, 3]]]]]), 0)) # (tensor([[[[[0., 1., 2., 3.]]]]]), 0) td(torch.tensor([[[[[0, 1, 2, 3]]]]])) # tensor([[[[[0., 1., 2., 3.]]]]]) td((torch.tensor([[0, 1, 2, 3]], dtype=torch.int32), 0)) td((torch.tensor([[0., 1., 2., 3.]]), 0)) # float32 td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float32), 0)) td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float64), 0)) td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex64 td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=torch.complex64), 0)) td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=torch.complex32), 0)) # (tensor([[0., 1., 2., 3.]]), 0) td((torch.tensor([[True, False, True, False]]), 0)) # bool td((torch.tensor([[True, False, True, False]], dtype=torch.bool), 0)) # (tensor([[1., 0., 1., 0.]]), 0) td((np.array(3), 0)) # int32 td((np.array(3, dtype=np.int32), 0)) # (array(3), 0) td(np.array(3)) # array(3) td((np.array([0, 1, 2, 3]), 0)) # (array([0, 1, 2, 3]), 0) td(np.array([0, 1, 2, 3])) # array([0, 1, 2, 3]) td((np.array([[0, 1, 2, 3]]), 0)) # (array([[0, 1, 2, 3]]), 0) td(np.array([[0, 1, 2, 3]])) # array([[0, 1, 2, 3]]) td((np.array([[[0, 1, 2, 3]]]), 0)) # (array([[[0, 1, 2, 3]]]), 0) td(np.array([[[0, 1, 2, 3]]])) # array([[[0, 1, 2, 3]]]) td((np.array([[[[0, 1, 2, 3]]]]), 0)) # (array([[[[0, 1, 2, 3]]]]), 0) td(np.array([[[[0, 1, 2, 3]]]])) # array([[[[0, 1, 2, 3]]]]) td((np.array([[[[[0, 1, 2, 3]]]]]), 0)) # (array([[[[[0, 1, 2, 3]]]]]), 0) td(np.array([[[[[0, 1, 2, 3]]]]])) # array([[[[[0, 1, 2, 3]]]]]) td((np.array([[0, 1, 2, 3]], dtype=np.int64), 0)) # (array([[0, 1, 2, 3]], dtype=int64), 0) td((np.array([[0., 1., 2., 3.]]), 0)) # float64 td((np.array([[0., 1., 2., 3.]], dtype=np.float64), 0)) # (array([[0., 1., 2., 3.]]), 0) td((np.array([[0., 1., 2., 3.]], dtype=np.float32), 0)) # (array([[0., 1., 2., 3.]], dtype=float32), 0) td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex128 td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex128), 0)) # (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0) td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex64), 0)) # (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=complex64), 0) td((np.array([[True, False, True, False]]), 0)) # bool td((np.array([[True, False, True, False]], dtype=bool), 0))
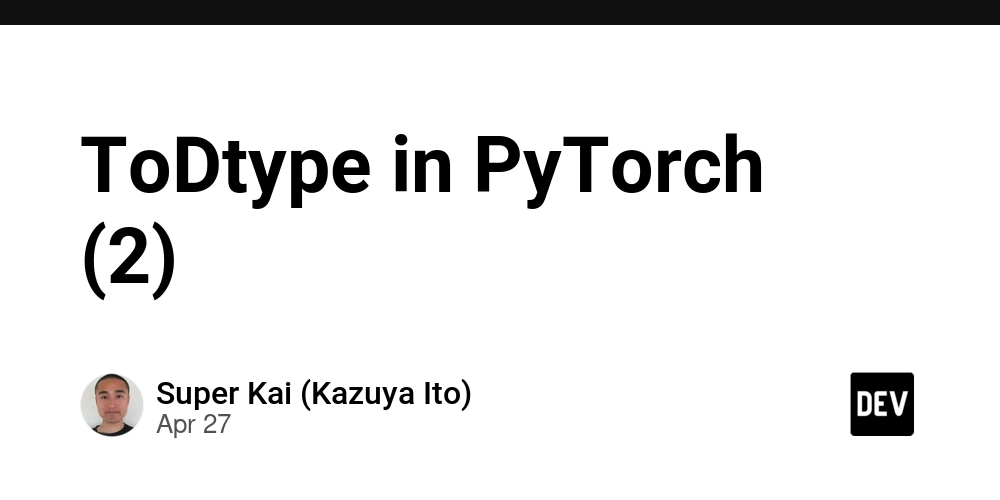
*Memos:
-
My post explains ToDtype() about
scale=True
. - My post explains how to convert and scale a PIL Image to an Image in PyTorch.
- My post explains Compose().
- My post explains ToImage().
- My post explains ToTensor().
- My post explains PILToTensor().
- My post explains ToPILImage().
- My post explains OxfordIIITPet().
ToDtype() can set a dtype to an Image, Video or tensor and scale its values as shown below. *It's about scale=False
:
from torchvision.datasets import OxfordIIITPet
from torchvision.transforms.v2 import ToImage, ToDtype
import torch
import numpy as np
PILImage_data = OxfordIIITPet(
root="data",
transform=None
)
Image_data = OxfordIIITPet(
root="data",
transform=ToImage()
)
td = ToDtype(dtype=torch.float32, scale=False)
td(PILImage_data[0])
# (, 0)
list(td(PILImage_data[0][0]).getdata())
# [(37, 20, 12),
# (35, 18, 10),
# (36, 19, 11),
# (36, 19, 11),
# (37, 18, 11),
# ...]
td(Image_data[0])
# (Image([[[37., 35., 36., ..., 247., 249., 249.],
# [35., 35., 37., ..., 246., 248., 249.],
# ...,
# [28., 28., 27., ..., 59., 65., 76.]],
# [[20., 18., 19., ..., 248., 248., 248.],
# [18., 18., 20., ..., 247., 247., 248.],
# ...,
# [27., 27., 27., ..., 94., 106., 117.]],
# [[12., 10., 11., ..., 253., 253., 253.],
# [10., 10., 12., ..., 251., 252., 253.],
# ...,
# [35., 35., 35., ..., 214., 232., 223.]]],), 0)
td(Image_data[0][0])
# Image([[[37, 35, 36, ..., 247, 249, 249],
# [35, 35, 37, ..., 246, 248, 249],
# ...,
# [28, 28, 27, ..., 59, 65, 76]],
# [[20, 18, 19, ..., 248, 248, 248],
# [18, 18, 20, ..., 247, 247, 248],
# ...,
# [27, 27, 27, ..., 94, 106, 117]],
# [[12, 10, 11, ..., 253, 253, 253],
# [10, 10, 12, ..., 251, 252, 253],
# ...,
# [35, 35, 35, ..., 214, 232, 223]]],)
td((torch.tensor(3), 0)) # int64
td((torch.tensor(3, dtype=torch.int64), 0))
# (tensor(3.), 0)
td(torch.tensor(3))
# tensor(3.)
td((torch.tensor([0, 1, 2, 3]), 0))
# (tensor([0., 1., 2., 3.]), 0)
td(torch.tensor([0, 1, 2, 3]))
# tensor([0., 1., 2., 3.])
td((torch.tensor([[0, 1, 2, 3]]), 0))
# (tensor([[0., 1., 2., 3.]]), 0)
td(torch.tensor([[0, 1, 2, 3]]))
# tensor([[0., 1., 2., 3.]])
td((torch.tensor([[[0, 1, 2, 3]]]), 0))
# (tensor([[[0., 1., 2., 3.]]]), 0)
td(torch.tensor([[[0, 1, 2, 3]]]))
# tensor([[[0., 1., 2., 3.]]])
td((torch.tensor([[[[0, 1, 2, 3]]]]), 0))
# (tensor([[[[0., 1., 2., 3.]]]]), 0)
td(torch.tensor([[[[0, 1, 2, 3]]]]))
# tensor([[[[0., 1., 2., 3.]]]])
td((torch.tensor([[[[[0, 1, 2, 3]]]]]), 0))
# (tensor([[[[[0., 1., 2., 3.]]]]]), 0)
td(torch.tensor([[[[[0, 1, 2, 3]]]]]))
# tensor([[[[[0., 1., 2., 3.]]]]])
td((torch.tensor([[0, 1, 2, 3]], dtype=torch.int32), 0))
td((torch.tensor([[0., 1., 2., 3.]]), 0)) # float32
td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float32), 0))
td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float64), 0))
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex64
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]],
dtype=torch.complex64), 0))
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]],
dtype=torch.complex32), 0))
# (tensor([[0., 1., 2., 3.]]), 0)
td((torch.tensor([[True, False, True, False]]), 0)) # bool
td((torch.tensor([[True, False, True, False]], dtype=torch.bool), 0))
# (tensor([[1., 0., 1., 0.]]), 0)
td((np.array(3), 0)) # int32
td((np.array(3, dtype=np.int32), 0))
# (array(3), 0)
td(np.array(3))
# array(3)
td((np.array([0, 1, 2, 3]), 0))
# (array([0, 1, 2, 3]), 0)
td(np.array([0, 1, 2, 3]))
# array([0, 1, 2, 3])
td((np.array([[0, 1, 2, 3]]), 0))
# (array([[0, 1, 2, 3]]), 0)
td(np.array([[0, 1, 2, 3]]))
# array([[0, 1, 2, 3]])
td((np.array([[[0, 1, 2, 3]]]), 0))
# (array([[[0, 1, 2, 3]]]), 0)
td(np.array([[[0, 1, 2, 3]]]))
# array([[[0, 1, 2, 3]]])
td((np.array([[[[0, 1, 2, 3]]]]), 0))
# (array([[[[0, 1, 2, 3]]]]), 0)
td(np.array([[[[0, 1, 2, 3]]]]))
# array([[[[0, 1, 2, 3]]]])
td((np.array([[[[[0, 1, 2, 3]]]]]), 0))
# (array([[[[[0, 1, 2, 3]]]]]), 0)
td(np.array([[[[[0, 1, 2, 3]]]]]))
# array([[[[[0, 1, 2, 3]]]]])
td((np.array([[0, 1, 2, 3]], dtype=np.int64), 0))
# (array([[0, 1, 2, 3]], dtype=int64), 0)
td((np.array([[0., 1., 2., 3.]]), 0)) # float64
td((np.array([[0., 1., 2., 3.]], dtype=np.float64), 0))
# (array([[0., 1., 2., 3.]]), 0)
td((np.array([[0., 1., 2., 3.]], dtype=np.float32), 0))
# (array([[0., 1., 2., 3.]], dtype=float32), 0)
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex128
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex128), 0))
# (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex64), 0))
# (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=complex64), 0)
td((np.array([[True, False, True, False]]), 0)) # bool
td((np.array([[True, False, True, False]], dtype=bool), 0))
# (array([[True, False, True, False]]), 0)
td = ToDtype(dtype=torch.complex64, scale=False)
td(PILImage_data[0])
# (, 0)
list(td(PILImage_data[0][0]).getdata())
# [(37, 20, 12),
# (35, 18, 10),
# (36, 19, 11),
# (36, 19, 11),
# (37, 18, 11),
# ...]
td(Image_data[0])
# (Image([[[37.+0.j, 35.+0.j, 36.+0.j, ..., 247.+0.j, 249.+0.j, 249.+0.j],
# [35.+0.j, 35.+0.j, 37.+0.j, ..., 246.+0.j, 248.+0.j, 249.+0.j],
# ...,
# [28.+0.j, 28.+0.j, 27.+0.j, ..., 59.+0.j, 65.+0.j, 76.+0.j]],
# [[20.+0.j, 18.+0.j, 19.+0.j, ..., 248.+0.j, 248.+0.j, 248.+0.j],
# [18.+0.j, 18.+0.j, 20.+0.j, ..., 247.+0.j, 247.+0.j, 248.+0.j],
# ...,
# [27.+0.j, 27.+0.j, 27.+0.j, ..., 94.+0.j, 106.+0.j, 117.+0.j]],
# [[12.+0.j, 10.+0.j, 11.+0.j, ..., 253.+0.j, 253.+0.j, 253.+0.j],
# [10.+0.j, 10.+0.j, 12.+0.j, ..., 251.+0.j, 252.+0.j, 253.+0.j],
# ...,
# [35.+0.j, 35.+0.j, 35.+0.j, ..., 214.+0.j, 232.+0.j, 223.+0.j]]],),
# 0)
td(Image_data[0][0])
# Image([[[37.+0.j, 35.+0.j, 36.+0.j, ..., 247.+0.j, 249.+0.j, 249.+0.j],
# [35.+0.j, 35.+0.j, 37.+0.j, ..., 246.+0.j, 248.+0.j, 249.+0.j],
# ...,
# [28.+0.j, 28.+0.j, 27.+0.j, ..., 59.+0.j, 65.+0.j, 76.+0.j]],
# [[20.+0.j, 18.+0.j, 19.+0.j, ..., 248.+0.j, 248.+0.j, 248.+0.j],
# [18.+0.j, 18.+0.j, 20.+0.j, ..., 247.+0.j, 247.+0.j, 248.+0.j],
# ...,
# [27.+0.j, 27.+0.j, 27.+0.j, ..., 94.+0.j, 106.+0.j, 117.+0.j]],
# [[12.+0.j, 10.+0.j, 11.+0.j, ..., 253.+0.j, 253.+0.j, 253.+0.j],
# [10.+0.j, 10.+0.j, 12.+0.j, ..., 251.+0.j, 252.+0.j, 253.+0.j],
# ...,
# [35.+0.j, 35.+0.j, 35.+0.j, ..., 214.+0.j, 232.+0.j, 223.+0.j]]],)
td((torch.tensor(3), 0)) # int64
td((torch.tensor(3, dtype=torch.int64), 0))
# (tensor(3.+0.j), 0)
td(torch.tensor(3))
# tensor(3.+0.j)
td((torch.tensor([0, 1, 2, 3]), 0))
# (tensor([0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]), 0)
td(torch.tensor([0, 1, 2, 3]))
# tensor([0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j])
td((torch.tensor([[0, 1, 2, 3]]), 0))
# (tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)
td(torch.tensor([[0, 1, 2, 3]]))
# tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]])
td((torch.tensor([[[0, 1, 2, 3]]]), 0))
# (tensor([[[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]]), 0)
td(torch.tensor([[[0, 1, 2, 3]]]))
# tensor([[[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]])
td((torch.tensor([[[[0, 1, 2, 3]]]]), 0))
# (tensor([[[[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]]]), 0)
td(torch.tensor([[[[0, 1, 2, 3]]]]))
# tensor([[[[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]]])
td((torch.tensor([[[[[0, 1, 2, 3]]]]]), 0))
# (tensor([[[[[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]]]]), 0)
td(torch.tensor([[[[[0, 1, 2, 3]]]]]))
# tensor([[[[[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]]]])
td((torch.tensor([[0, 1, 2, 3]], dtype=torch.int32), 0))
# (tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)
td((torch.tensor([[0., 1., 2., 3.]]), 0)) # float32
td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float32), 0))
td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float64), 0))
# (tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex64
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]],
dtype=torch.complex64), 0))
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]],
dtype=torch.complex32), 0))
# (tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)
td((torch.tensor([[True, False, True, False]]), 0)) # bool
td((torch.tensor([[True, False, True, False]], dtype=torch.bool), 0))
# (tensor([[1.+0.j, 0.+0.j, 1.+0.j, 0.+0.j]]), 0)
td((np.array(3), 0)) # int32
td((np.array(3, dtype=np.int32), 0))
# (array(3), 0)
td(np.array(3))
# array(3)
td((np.array([0, 1, 2, 3]), 0))
# (array([0, 1, 2, 3]), 0)
td(np.array([0, 1, 2, 3]))
# array([0, 1, 2, 3])
td((np.array([[0, 1, 2, 3]]), 0))
# (array([[0, 1, 2, 3]]), 0)
td(np.array([[0, 1, 2, 3]]))
# array([[0, 1, 2, 3]])
td((np.array([[[0, 1, 2, 3]]]), 0))
# (array([[[0, 1, 2, 3]]]), 0)
td(np.array([[[0, 1, 2, 3]]]))
# array([[[0, 1, 2, 3]]])
td((np.array([[0, 1, 2, 3]], dtype=np.int64), 0))
# (array([[0, 1, 2, 3]], dtype=int64), 0)
td((np.array([[0., 1., 2., 3.]]), 0)) # float64
td((np.array([[0., 1., 2., 3.]], dtype=np.float64), 0))
# (array([[0., 1., 2., 3.]]), 0)
td((np.array([[0., 1., 2., 3.]], dtype=np.float32), 0))
# (array([[0., 1., 2., 3.]], dtype=float32), 0)
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex128
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex128), 0))
# (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex64), 0))
# (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=complex64), 0)
td((np.array([[True, False, True, False]]), 0)) # bool
td((np.array([[True, False, True, False]], dtype=bool), 0))
# (array([[True, False, True, False]]), 0)
td = ToDtype(dtype=torch.bool, scale=False)
td(PILImage_data[0])
# (, 0)
list(td(PILImage_data[0][0]).getdata())
# [(37, 20, 12),
# (35, 18, 10),
# (36, 19, 11),
# (36, 19, 11),
# (37, 18, 11),
# ...]
td(Image_data[0])
# (Image([[[True, True, True, ..., True, True, True],
# [True, True, True, ..., True, True, True],
# ...,
# [True, True, True, ..., True, True, True]],
# [[True, True, True, ..., True, True, True],
# [True, True, True, ..., True, True, True],
# ...,
# [True, True, True, ..., True, True, True]],
# [[True, True, True, ..., True, True, True],
# [True, True, True, ..., True, True, True],
# ...,
# [True, True, True, ..., True, True, True]]],), 0)
td(Image_data[0][0])
# Image([[[True, True, True, ..., True, True, True],
# [True, True, True, ..., True, True, True],
# ...,
# [True, True, True, ..., True, True, True]],
# [[True, True, True, ..., True, True, True],
# [True, True, True, ..., True, True, True],
# ...,
# [True, True, True, ..., True, True, True]],
# [[True, True, True, ..., True, True, True],
# [True, True, True, ..., True, True, True],
# ...,
# [True, True, True, ..., True, True, True]]],)
td((torch.tensor(3), 0)) # int64
td((torch.tensor(3, dtype=torch.int64), 0))
# (tensor(True), 0)
td(torch.tensor(3))
# tensor(True)
td((torch.tensor([0, 1, 2, 3]), 0))
# (tensor([False, True, True, True]), 0)
td(torch.tensor([0, 1, 2, 3]))
# tensor([False, True, True, True])
td((torch.tensor([[0, 1, 2, 3]]), 0))
# (tensor([[False, True, True, True]]), 0)
td(torch.tensor([[0, 1, 2, 3]]))
# tensor([[False, True, True, True]])
td((torch.tensor([[[0, 1, 2, 3]]]), 0))
# (tensor([[[False, True, True, True]]]), 0)
td(torch.tensor([[[0, 1, 2, 3]]]))
# tensor([[[False, True, True, True]]])
td((torch.tensor([[[[0, 1, 2, 3]]]]), 0))
# (tensor([[[[False, True, True, True]]]]), 0)
td(torch.tensor([[[[0, 1, 2, 3]]]]))
# tensor([[[[False, True, True, True]]]])
td((torch.tensor([[[[[0, 1, 2, 3]]]]]), 0))
# (tensor([[[[[False, True, True, True]]]]]), 0)
td(torch.tensor([[[[[0, 1, 2, 3]]]]]))
# tensor([[[[[False, True, True, True]]]]])
td((torch.tensor([[0, 1, 2, 3]], dtype=torch.int32), 0))
# (tensor([[False, True, True, True]]), 0)
td((torch.tensor([[0., 1., 2., 3.]]), 0)) # float32
td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float32), 0))
td((torch.tensor([[0., 1., 2., 3.]], dtype=torch.float64), 0))
# (tensor([[False, True, True, True]]), 0)
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex64
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]],
dtype=torch.complex64), 0))
td((torch.tensor([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]],
dtype=torch.complex32), 0))
# (tensor([[False, True, True, True]]), 0)
td((torch.tensor([[True, False, True, False]]), 0)) # bool
td((torch.tensor([[True, False, True, False]], dtype=torch.bool), 0))
# (tensor([[True, False, True, False]]), 0)
td((np.array(3), 0)) # int32
td((np.array(3, dtype=np.int32), 0))
# (array(3), 0)
td(np.array(3))
# array(3)
td((np.array([0, 1, 2, 3]), 0))
# (array([0, 1, 2, 3]), 0)
td(np.array([0, 1, 2, 3]))
# array([0, 1, 2, 3])
td((np.array([[0, 1, 2, 3]]), 0))
# (array([[0, 1, 2, 3]]), 0)
td(np.array([[0, 1, 2, 3]]))
# array([[0, 1, 2, 3]])
td((np.array([[[0, 1, 2, 3]]]), 0))
# (array([[[0, 1, 2, 3]]]), 0)
td(np.array([[[0, 1, 2, 3]]]))
# array([[[0, 1, 2, 3]]])
td((np.array([[0, 1, 2, 3]], dtype=np.int64), 0))
# (array([[0, 1, 2, 3]], dtype=int64), 0)
td((np.array([[0., 1., 2., 3.]]), 0)) # float64
td((np.array([[0., 1., 2., 3.]], dtype=np.float64), 0))
# (array([[0., 1., 2., 3.]]), 0)
td((np.array([[0., 1., 2., 3.]], dtype=np.float32), 0))
# (array([[0., 1., 2., 3.]], dtype=float32), 0)
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)) # complex128
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex128), 0))
# (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]]), 0)
td((np.array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=np.complex64), 0))
# (array([[0.+0.j, 1.+0.j, 2.+0.j, 3.+0.j]], dtype=complex64), 0)
td((np.array([[True, False, True, False]]), 0)) # bool
td((np.array([[True, False, True, False]], dtype=bool), 0))
# (array([[True, False, True, False]]), 0)