The Ultimate Guide to Web Accessibility Testing: From Screen Readers to LighthouseCI
Accessibility Testing Accessibility testing is one of the most underrated yet critical aspects of web development. As someone who’s both a dark-mode addict and a self-proclaimed "bug magnet," I’ve come to realize that accessibility isn’t just about supporting disabled users—it’s about creating a seamless, inclusive experience for everyone. What is Accessibility Testing? Accessibility testing ensures that web applications are usable by everyone, including individuals with visual, auditory, motor, and cognitive impairments. This process follows the Web Content Accessibility Guidelines (WCAG) to ensure web content is: Perceivable — Content must be presented in a way that users can experience (e.g., alternative text for images, captions for videos). Operable — Users should be able to navigate and interact seamlessly (e.g., keyboard accessibility, no time-based restrictions). Understandable — Content must be clear and predictable (e.g., user-friendly error messages, readable text). Robust — Web content should be compatible with assistive technologies like screen readers and voice navigation. Understanding WCAG The Web Content Accessibility Guidelines (WCAG), developed by the W3C (Yes! like Selenium), are international standards designed to make the web more inclusive. WCAG Compliance Levels: A (Basic) — Covers fundamental accessibility features (e.g., alternative text for images). AA (Recommended) — Addresses major barriers (e.g., proper color contrast, keyboard focus). AAA (Advanced) — Ensures the highest level of accessibility (e.g., sign language interpretation). Manual Accessibility Testing Tools While automated tools detect many issues, manual testing is crucial to ensure a truly accessible user experience. Screen Readers: NVDA (NonVisual Desktop Access) — Free, open-source screen reader for Windows. JAWS (Job Access With Speech) — Paid, widely used in corporate environments. VoiceOver (Mac & iOS) — Built-in screen reader for Apple devices. Browser Extensions: WAVE (Web Accessibility Evaluation Tool) — Highlights accessibility errors visually. Axe DevTools — Detects WCAG violations and suggests code fixes. Accessibility Insights (Microsoft) — Assists in testing keyboard navigation, ARIA roles, and screen reader compatibility. Accessibility FastPass Report Keyboard Navigation Testing: Test your website using Tab, Shift+Tab, Enter, Space, Esc, and Arrow keys. Ensure focus indicators are clearly visible using :focus in CSS. Prevent keyboard traps (users should be able to exit modals using the Esc key). Automated Accessibility Testing Tools Speed up your accessibility testing by integrating automated tools into your workflow! Google Lighthouse (Built into Chrome DevTools) Checks accessibility, performance, and SEO in one go. Run via Chrome DevTools → Lighthouse Tab. Pa11y (CLI Tool for Automated Testing) Executes accessibility tests via the command line. Manual testing is possible with just a couple of commands. Easily integrates into CI/CD pipelines. Example Pa11y Run with Custom Options from CLI: #bash pa11y https://example.com \ --runner axe \ --reporter html > report.html \ --standard WCAG2AA \ --timeout 30000 \ --hide-elements '#ad-banner, .popup' axe-core + Selenium/WebDriver Automates accessibility tests within Selenium-based test suites. Ensures elements have correct labels, alt text, and keyboard focus. Dependencies: org.seleniumhq.selenium selenium-java 4.29.0 com.deque.html.axe-core selenium 4.10.1 Selenium Example: import static com.velespit.utilities.Driver.*; import com.deque.html.axecore.results.Results; import com.deque.html.axecore.results.Rule; import com.deque.html.axecore.selenium.AxeBuilder; import org.junit.Test; import org.openqa.selenium.JavascriptExecutor; import java.net.URL; import java.util.List; @Test public void accessTest() { try { getDriver().get("https://yusufasik.com"); URL axeScriptUrl = AccessibilityTest.class.getResource("/axe.min.js"); JavascriptExecutor js = (JavascriptExecutor) getDriver(); js.executeScript("var script = document.createElement('script');" + "script.src = arguments[0];" + "document.head.appendChild(script);", axeScriptUrl.toString()); AxeBuilder axeBuilder = new AxeBuilder(); Results results = axeBuilder.analyze(getDriver()); List violations = results.getViolations(); if (violations.isEmpty()) { System.out.println("No accessibility issues found."); } else { System.out.println("Accessibility Issues Found:"); for (Rule violation : violations) { System.out.println("Violation: "
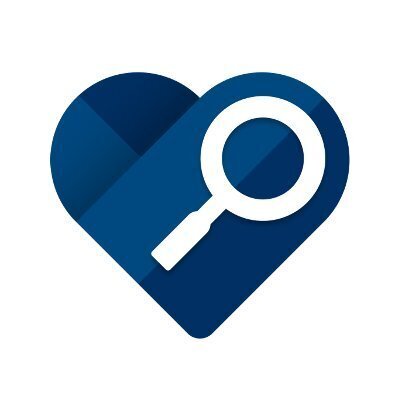
Accessibility Testing
Accessibility testing is one of the most underrated yet critical aspects of web development. As someone who’s both a dark-mode addict and a self-proclaimed "bug magnet," I’ve come to realize that accessibility isn’t just about supporting disabled users—it’s about creating a seamless, inclusive experience for everyone.
What is Accessibility Testing?
Accessibility testing ensures that web applications are usable by everyone, including individuals with visual, auditory, motor, and cognitive impairments. This process follows the Web Content Accessibility Guidelines (WCAG) to ensure web content is:
- Perceivable — Content must be presented in a way that users can experience (e.g., alternative text for images, captions for videos).
- Operable — Users should be able to navigate and interact seamlessly (e.g., keyboard accessibility, no time-based restrictions).
- Understandable — Content must be clear and predictable (e.g., user-friendly error messages, readable text).
- Robust — Web content should be compatible with assistive technologies like screen readers and voice navigation.
Understanding WCAG
The Web Content Accessibility Guidelines (WCAG), developed by the W3C (Yes! like Selenium), are international standards designed to make the web more inclusive.
WCAG Compliance Levels:
- A (Basic) — Covers fundamental accessibility features (e.g., alternative text for images).
- AA (Recommended) — Addresses major barriers (e.g., proper color contrast, keyboard focus).
- AAA (Advanced) — Ensures the highest level of accessibility (e.g., sign language interpretation).
Manual Accessibility Testing Tools
While automated tools detect many issues, manual testing is crucial to ensure a truly accessible user experience.
Screen Readers:
- NVDA (NonVisual Desktop Access) — Free, open-source screen reader for Windows.
- JAWS (Job Access With Speech) — Paid, widely used in corporate environments.
- VoiceOver (Mac & iOS) — Built-in screen reader for Apple devices.
Browser Extensions:
- WAVE (Web Accessibility Evaluation Tool) — Highlights accessibility errors visually.
- Axe DevTools — Detects WCAG violations and suggests code fixes.
- Accessibility Insights (Microsoft) — Assists in testing keyboard navigation, ARIA roles, and screen reader compatibility.
Keyboard Navigation Testing:
- Test your website using
Tab
,Shift+Tab
,Enter
,Space
,Esc
, andArrow
keys. - Ensure focus indicators are clearly visible using
:focus
in CSS. - Prevent keyboard traps (users should be able to exit modals using the
Esc
key).
Automated Accessibility Testing Tools
Speed up your accessibility testing by integrating automated tools into your workflow!
Google Lighthouse (Built into Chrome DevTools)
- Checks accessibility, performance, and SEO in one go.
- Run via Chrome DevTools → Lighthouse Tab.
Pa11y (CLI Tool for Automated Testing)
- Executes accessibility tests via the command line.
- Manual testing is possible with just a couple of commands.
- Easily integrates into CI/CD pipelines.
Example Pa11y Run with Custom Options from CLI:
#bash
pa11y https://example.com \
--runner axe \
--reporter html > report.html \
--standard WCAG2AA \
--timeout 30000 \
--hide-elements '#ad-banner, .popup'
axe-core + Selenium/WebDriver
- Automates accessibility tests within Selenium-based test suites.
- Ensures elements have correct labels, alt text, and keyboard focus.
Dependencies:
org.seleniumhq.selenium
selenium-java
4.29.0
com.deque.html.axe-core
selenium
4.10.1
Selenium Example:
import static com.velespit.utilities.Driver.*;
import com.deque.html.axecore.results.Results;
import com.deque.html.axecore.results.Rule;
import com.deque.html.axecore.selenium.AxeBuilder;
import org.junit.Test;
import org.openqa.selenium.JavascriptExecutor;
import java.net.URL;
import java.util.List;
@Test
public void accessTest() {
try {
getDriver().get("https://yusufasik.com");
URL axeScriptUrl = AccessibilityTest.class.getResource("/axe.min.js");
JavascriptExecutor js = (JavascriptExecutor) getDriver();
js.executeScript("var script = document.createElement('script');" +
"script.src = arguments[0];" +
"document.head.appendChild(script);", axeScriptUrl.toString());
AxeBuilder axeBuilder = new AxeBuilder();
Results results = axeBuilder.analyze(getDriver());
List<Rule> violations = results.getViolations();
if (violations.isEmpty()) {
System.out.println("No accessibility issues found.");
} else {
System.out.println("Accessibility Issues Found:");
for (Rule violation : violations) {
System.out.println("Violation: " + violation.getId());
System.out.println("Description: " + violation.getDescription());
System.out.println("Help: " + violation.getHelp());
System.out.println("Help URL: " + violation.getHelpUrl());
System.out.println("Impact: " + violation.getImpact());
System.out.println("Tags: " + violation.getTags());
System.out.println("Nodes: " + violation.getNodes().size());
System.out.println("--------------------");
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
closeDriver();
}
}
Integrating Accessibility Testing into CI/CD Pipelines
Want to ensure accessibility compliance continuously? Automate it in your CI/CD workflow:
Lighthouse CI (Jenkins)
machine-setup.sh
#!/bin/bash
set -euxo pipefail
# Add Chrome's apt-key
echo "deb [arch=amd64] http://dl.google.com/linux/chrome/deb/ stable main" | sudo tee -a /etc/apt/sources.list.d/google.list
wget -q -O - https://dl.google.com/linux/linux_signing_key.pub | sudo apt-key add -
# Add Node's apt-key
curl -sL https://deb.nodesource.com/setup_20.x | sudo -E bash -
# Install NodeJS and Google Chrome
sudo apt update
sudo apt install -y nodejs google-chrome-stable
job.sh
#!/bin/bash
set -euxo pipefail
npm install
npm run build
export CHROME_PATH=$(which google-chrome-stable)
export LHCI_BUILD_CONTEXT__EXTERNAL_BUILD_URL="$BUILD_URL"
npm install -g @lhci/cli@0.14.x
lhci autorun
Make sure to check full guide in my repo:
Pa11y CI
- Integrates accessibility checks into CI/CD pipelines.
Axe CLI + Jest
- Adds automated accessibility tests to unit testing.
When Should Accessibility Testing Be Performed?
- During Development — Use linters and static analysis tools to catch accessibility issues early.
- UI Testing (System & Integration Testing) — Use tools like axe, WAVE, Lighthouse.
- Before UAT (Performance & Functional Testing) — Ensure accessibility features do not impact performance.
- During UAT (Final Validation Before Release) — Validate real-world usability and WCAG compliance.
Key Takeaways
- Accessibility is a journey, not a checkbox.
- Manual tools (axe, NVDA) catch UX gaps; automation (Lighthouse, pa11y) scales compliance.
- Test early and often — integrate tools into your workflow with CI/CD pipelines for long-term compliance.
Further Reading:
Make accessibility a priority because the web should be for everyone!