Technical assessments for junior software developers
typically cover fundamental programming concepts, problem-solving skills, and basic software development practices. Here are 20 common types of assessments: Programming Basics Writing simple programs in languages like Python, Java, or JavaScript Understanding syntax, variables, loops, and conditionals Data Structures & Algorithms Arrays, Linked Lists, Hash Tables, Stacks, and Queues Sorting algorithms (Bubble Sort, Merge Sort, Quick Sort) Searching algorithms (Binary Search, Linear Search) Object-Oriented Programming (OOP) Classes and Objects Inheritance, Encapsulation, and Polymorphism Design principles like SOLID Database Basics Writing SQL queries (SELECT, INSERT, UPDATE, DELETE) Understanding database normalization and relationships Basic knowledge of NoSQL (MongoDB, Firebase) Web Development Fundamentals HTML, CSS, JavaScript basics RESTful APIs and HTTP methods Understanding JSON and XML Version Control (Git/GitHub) Basic Git commands (clone, commit, push, pull, branch) Understanding merge conflicts Debugging & Code Analysis Finding and fixing syntax and logical errors Using debugging tools in IDEs like VS Code, IntelliJ, or PyCharm Unit Testing Writing test cases using Jest, JUnit, or PyTest Understanding test-driven development (TDD) Problem-Solving Challenges Solving coding challenges on platforms like LeetCode, HackerRank, or CodeSignal API Integration Working with third-party APIs (Google Maps API, Stripe, etc.) Handling API authentication (OAuth, API keys) Frontend Frameworks (Basics) Understanding React, Vue.js, or Angular fundamentals State management concepts Backend Development (Basics) Introduction to Node.js, Express, or Django Writing simple server-side logic Software Development Lifecycle (SDLC) Understanding Agile and Scrum methodologies Writing basic documentation Code Optimization Reducing time complexity in algorithms Avoiding unnecessary memory usage Security Basics Understanding common vulnerabilities (SQL Injection, XSS, CSRF) Basic knowledge of authentication and authorization Operating Systems & Command Line Basic Linux/Unix commands (ls, cd, mkdir, grep) Understanding process management and permissions Cloud Computing Fundamentals Basics of AWS, Azure, or Google Cloud Deploying simple applications Containerization (Intro to Docker) Understanding Docker and containerized applications Writing simple Dockerfiles Continuous Integration/Continuous Deployment (CI/CD) Understanding Jenkins, GitHub Actions, or Travis CI Setting up simple automated pipelines Soft Skills Assessment Code readability and maintainability Communication and teamwork skills
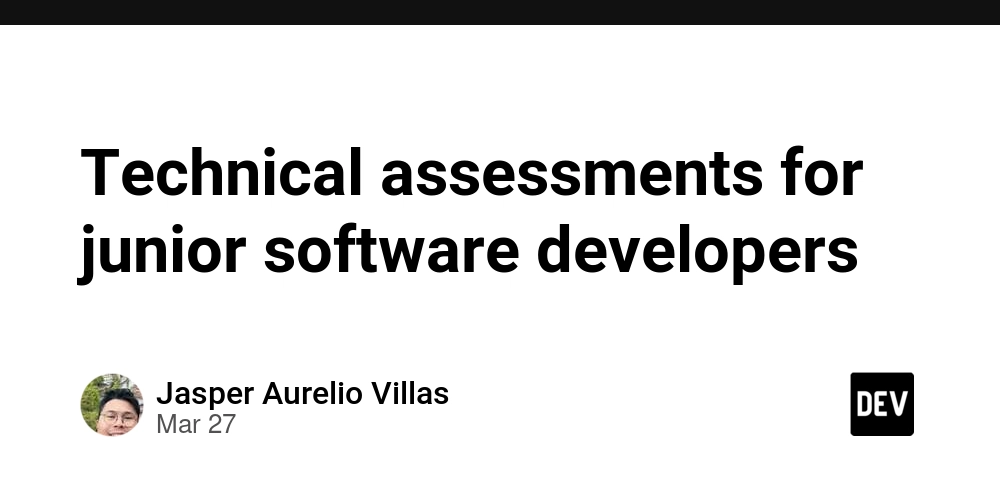
typically cover fundamental programming concepts, problem-solving skills, and basic software development practices.
Here are 20 common types of assessments:
- Programming Basics Writing simple programs in languages like Python, Java, or JavaScript
Understanding syntax, variables, loops, and conditionals
- Data Structures & Algorithms Arrays, Linked Lists, Hash Tables, Stacks, and Queues
Sorting algorithms (Bubble Sort, Merge Sort, Quick Sort)
Searching algorithms (Binary Search, Linear Search)
- Object-Oriented Programming (OOP) Classes and Objects
Inheritance, Encapsulation, and Polymorphism
Design principles like SOLID
- Database Basics Writing SQL queries (SELECT, INSERT, UPDATE, DELETE)
Understanding database normalization and relationships
Basic knowledge of NoSQL (MongoDB, Firebase)
- Web Development Fundamentals HTML, CSS, JavaScript basics
RESTful APIs and HTTP methods
Understanding JSON and XML
- Version Control (Git/GitHub) Basic Git commands (clone, commit, push, pull, branch)
Understanding merge conflicts
- Debugging & Code Analysis Finding and fixing syntax and logical errors
Using debugging tools in IDEs like VS Code, IntelliJ, or PyCharm
- Unit Testing Writing test cases using Jest, JUnit, or PyTest
Understanding test-driven development (TDD)
Problem-Solving Challenges
Solving coding challenges on platforms like LeetCode, HackerRank, or CodeSignalAPI Integration
Working with third-party APIs (Google Maps API, Stripe, etc.)
Handling API authentication (OAuth, API keys)
- Frontend Frameworks (Basics) Understanding React, Vue.js, or Angular fundamentals
State management concepts
- Backend Development (Basics) Introduction to Node.js, Express, or Django
Writing simple server-side logic
- Software Development Lifecycle (SDLC) Understanding Agile and Scrum methodologies
Writing basic documentation
- Code Optimization Reducing time complexity in algorithms
Avoiding unnecessary memory usage
- Security Basics Understanding common vulnerabilities (SQL Injection, XSS, CSRF)
Basic knowledge of authentication and authorization
- Operating Systems & Command Line Basic Linux/Unix commands (ls, cd, mkdir, grep)
Understanding process management and permissions
- Cloud Computing Fundamentals Basics of AWS, Azure, or Google Cloud
Deploying simple applications
- Containerization (Intro to Docker) Understanding Docker and containerized applications
Writing simple Dockerfiles
- Continuous Integration/Continuous Deployment (CI/CD) Understanding Jenkins, GitHub Actions, or Travis CI
Setting up simple automated pipelines
- Soft Skills Assessment Code readability and maintainability
Communication and teamwork skills