Stop Storing Plain Text Passwords! Use Bcrypt for Security
When building an application that requires user authentication, one of the worst mistakes you can make is storing passwords in plain text. If your database gets hacked, attackers can see all user credentials instantly. That’s a huge security risk! Instead, passwords should always be stored in a hashed format. Hashing transforms a password into an unreadable string, making it nearly impossible to reverse-engineer. But simple hashing isn’t enough, adding salting and key stretching makes passwords even more secure. What Are Hashing, Salting, and Key Stretching? Hashing Hashing is a one-way function that converts a password into a fixed-length, irreversible string. This ensures that even if someone gains access to the database, they cannot retrieve the original password. If we hash the password password123 EF92B778BAF9C3D1C6CFE1C4A7316C83E4F2E9F288D5A68B84BC7E0549A9AFA3 Salting Salting adds a unique random value to each password before hashing. This ensures that even if two users have the same password, their hashes will be different. Password: password123 Salt: X7!a9@ Hashed Output: Hash(password123 + X7!a9@) Each time a password is stored, a different salt is generated, making attacks significantly harder. Key Stretching Key stretching is a technique that increases the computational time required to hash a password, making brute-force attacks more difficult. This is achieved by repeatedly applying the hashing algorithm multiple times. Instead of hashing once: Hash(password123) We hash multiple times: Hash(Hash(Hash(password123))) This increases the time required for attackers to compute password hashes, thereby enhancing security. Why Use Bcrypt? Bcrypt is one of the best tools for hashing passwords, because it automatically includes salting and key stretching. How Bcrypt coverts plain text into hash before storing in Database Generate a salt (a random value). Combine the password with the salt. Apply multiple rounds of hashing (key stretching) to slow down attacks. Store the final hashed password in the database. const hashedPassword = await bcrypt.hash(password, 10); How Bcrypt Compares Passwords When a user logs in, we need to check if their password matches the stored hash. Retrieve the stored hashed password from the database. Extract the salt from the stored hash. Hash the user-entered password using the extracted salt. Compare the newly generated hash with the stored hash. If they match, grant access; otherwise, deny access. const isMatch = await bcrypt.compare(enteredPassword, storedHash); Summing It Up Security should never be an afterthought, especially when dealing with user passwords. Bcrypt makes it easy to implement strong password hashing with salting, key stretching, and adaptability. By using bcrypt, you can protect user data and significantly reduce the risk of password-related breaches.
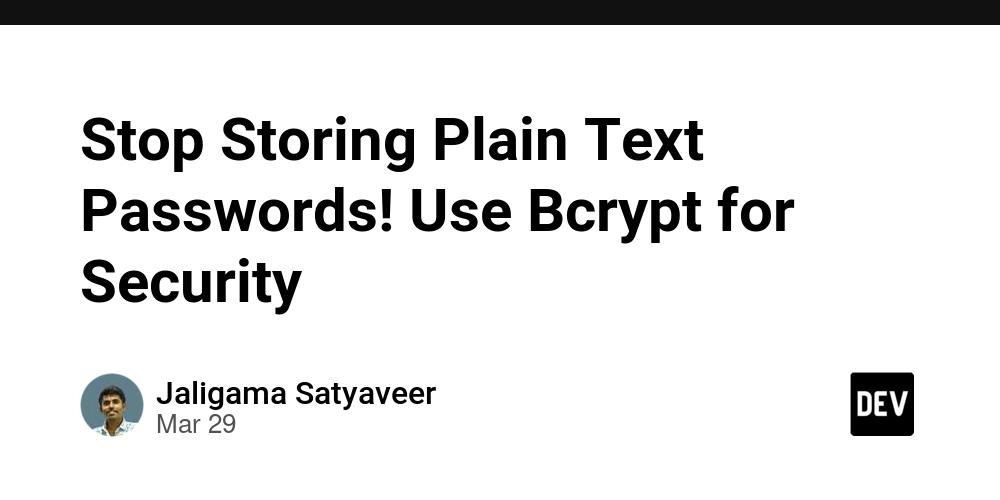
When building an application that requires user authentication, one of the worst mistakes you can make is storing passwords in plain text. If your database gets hacked, attackers can see all user credentials instantly. That’s a huge security risk!
Instead, passwords should always be stored in a hashed format. Hashing transforms a password into an unreadable string, making it nearly impossible to reverse-engineer. But simple hashing isn’t enough, adding salting and key stretching makes passwords even more secure.
What Are Hashing, Salting, and Key Stretching?
Hashing
Hashing is a one-way function that converts a password into a fixed-length, irreversible string. This ensures that even if someone gains access to the database, they cannot retrieve the original password.
If we hash the password password123
EF92B778BAF9C3D1C6CFE1C4A7316C83E4F2E9F288D5A68B84BC7E0549A9AFA3
Salting
Salting adds a unique random value to each password before hashing. This ensures that even if two users have the same password, their hashes will be different.
Password: password123
Salt: X7!a9@
Hashed Output: Hash(password123 + X7!a9@)
Each time a password is stored, a different salt is generated, making attacks significantly harder.
Key Stretching
Key stretching is a technique that increases the computational time required to hash a password, making brute-force attacks more difficult. This is achieved by repeatedly applying the hashing algorithm multiple times.
Instead of hashing once:
Hash(password123)
We hash multiple times:
Hash(Hash(Hash(password123)))
This increases the time required for attackers to compute password hashes, thereby enhancing security.
Why Use Bcrypt?
Bcrypt is one of the best tools for hashing passwords, because it automatically includes salting and key stretching.
How Bcrypt coverts plain text into hash before storing in Database
- Generate a salt (a random value).
- Combine the password with the salt.
- Apply multiple rounds of hashing (key stretching) to slow down attacks.
- Store the final hashed password in the database.
const hashedPassword = await bcrypt.hash(password, 10);
How Bcrypt Compares Passwords
When a user logs in, we need to check if their password matches the stored hash.
- Retrieve the stored hashed password from the database.
- Extract the salt from the stored hash.
- Hash the user-entered password using the extracted salt.
- Compare the newly generated hash with the stored hash.
- If they match, grant access; otherwise, deny access.
const isMatch = await bcrypt.compare(enteredPassword, storedHash);
Summing It Up
Security should never be an afterthought, especially when dealing with user passwords. Bcrypt makes it easy to implement strong password hashing with salting, key stretching, and adaptability. By using bcrypt, you can protect user data and significantly reduce the risk of password-related breaches.