STEP-BY-STEP GUIDE: Lambda + CRR + EC2 Control + SNS (Email)
Create an SNS Topic for Email Alerts Go to AWS Console > SNS > Topics Click Create topic Type: Standard Name: AlertTopic Click Create Under Subscriptions, click Create subscription Protocol: Email Endpoint: your email address Check your email inbox and confirm the subscription IAM Role for Lambda You’ll need a role with permissions to control EC2, publish to SNS, and read from S3. Go to IAM > Roles > Create Role Select AWS Service > Lambda Attach policies: AmazonEC2FullAccess AmazonSNSFullAccess AmazonS3ReadOnlyAccess Name the role: LambdaAutomationRole Enable Cross-Region Replication (CRR) Go to S3 > Create two buckets: Source: my-source-bucket-123 Destination: my-destination-bucket-123 Must be in different AWS regions Enable versioning on both buckets. In the source bucket, go to Management > Replication rules Add a rule: Source: Entire bucket Destination: Your destination bucket IAM Role: Create one or use an existing S3 replication role Save the rule — CRR is now enabled. Create Lambda for S3 CRR Monitoring Go to Lambda > Create Function Name: MonitorCRR Runtime: Python 3.10 Permissions: Choose Use existing role > Select LambdaAutomationRole Paste the code: import boto3 import json def lambda_handler(event, context): s3 = boto3.client('s3') sns = boto3.client('sns') for record in event['Records']: bucket = record['s3']['bucket']['name'] key = record['s3']['object']['key'] message = f"New object '{key}' added to bucket '{bucket}'. Check replication status." sns.publish( TopicArn='arn:aws:sns:your-region:your-account-id:AlertTopic', Message=message, Subject='[CRR Monitor] New S3 Upload' ) return { 'statusCode': 200, 'body': json.dumps('CRR notification sent.') } Click Deploy Connect S3 to Trigger Lambda Go to S3 > Your source bucket > Properties Scroll to Event Notifications Click Create Event Notification Name: NewUploadTrigger Event type: All object create events Destination: Lambda function > Choose MonitorCRR Create Lambda to Start/Stop EC2 with SNS Go to Lambda > Create Function Name: EC2ControlNotify Runtime: Python 3.10 Role: Use LambdaAutomationRole Paste this code: import boto3 ec2 = boto3.client('ec2') sns = boto3.client('sns') def lambda_handler(event, context): instance_id = 'i-xxxxxxxxxxxxxxxxx' # Replace with your EC2 instance ID action = 'start' # or 'stop' if action == 'start': ec2.start_instances(InstanceIds=[instance_id]) message = f"Started EC2 instance {instance_id}" else: ec2.stop_instances(InstanceIds=[instance_id]) message = f"Stopped EC2 instance {instance_id}" sns.publish( TopicArn='arn:aws:sns:your-region:your-account-id:AlertTopic', Message=message, Subject=f'[EC2 Control] {action.upper()} action performed' ) return {'status': 'success', 'message': message} Click Deploy (Optional) Trigger EC2 Lambda Automatically If you want to start/stop EC2 on schedule: Go to CloudWatch > Rules > Create rule Event Source: Schedule Example: cron(0 18 * * ? *) for 6 PM UTC daily Target: Lambda function > Choose EC2ControlNotify Test Everything Upload an object to the source S3 bucket → Check your email for CRR alert. Run EC2 Lambda manually → Instance should start/stop and email should arrive. Check CloudWatch Logs for debugging. Bonus Tip: Secure Your Setup Use environment variables in Lambda to avoid hardcoding instance IDs or SNS ARNs. Add logging and error handling. Use CloudTrail to audit changes.
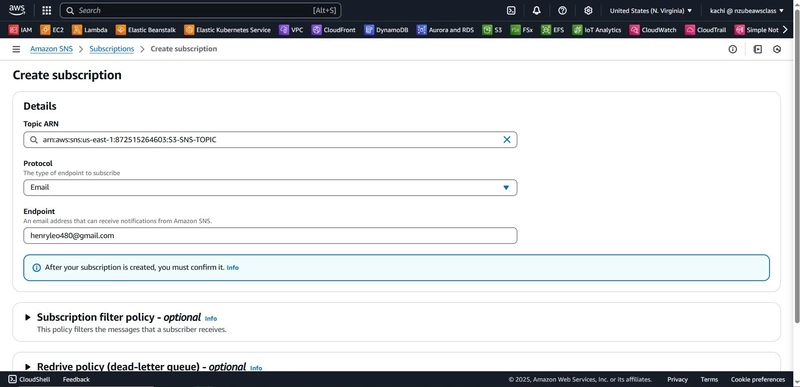
Create an SNS Topic for Email Alerts
- Go to AWS Console > SNS > Topics
- Click Create topic
- Type: Standard
- Name:
AlertTopic
- Click Create
- Under Subscriptions, click Create subscription
- Protocol:
Email
- Endpoint: your email address
- Protocol:
- Check your email inbox and confirm the subscription
IAM Role for Lambda
You’ll need a role with permissions to control EC2, publish to SNS, and read from S3.
- Go to IAM > Roles > Create Role
- Select AWS Service > Lambda
- Attach policies:
AmazonEC2FullAccess
AmazonSNSFullAccess
AmazonS3ReadOnlyAccess
- Name the role:
LambdaAutomationRole
Enable Cross-Region Replication (CRR)
- Go to S3 > Create two buckets:
- Source:
my-source-bucket-123
- Destination:
my-destination-bucket-123
- Must be in different AWS regions
- Source:
- Enable versioning on both buckets.
- In the source bucket, go to Management > Replication rules
- Add a rule:
- Source: Entire bucket
- Destination: Your destination bucket
- IAM Role: Create one or use an existing S3 replication role
- Add a rule:
- Save the rule — CRR is now enabled.
Create Lambda for S3 CRR Monitoring
- Go to Lambda > Create Function
- Name:
MonitorCRR
- Runtime: Python 3.10
- Permissions: Choose
Use existing role
> SelectLambdaAutomationRole
- Name:
- Paste the code:
import boto3
import json
def lambda_handler(event, context):
s3 = boto3.client('s3')
sns = boto3.client('sns')
for record in event['Records']:
bucket = record['s3']['bucket']['name']
key = record['s3']['object']['key']
message = f"New object '{key}' added to bucket '{bucket}'. Check replication status."
sns.publish(
TopicArn='arn:aws:sns:your-region:your-account-id:AlertTopic',
Message=message,
Subject='[CRR Monitor] New S3 Upload'
)
return {
'statusCode': 200,
'body': json.dumps('CRR notification sent.')
}
Click Deploy
Connect S3 to Trigger Lambda
- Go to S3 > Your source bucket > Properties
- Scroll to Event Notifications
- Click Create Event Notification
- Name:
NewUploadTrigger
- Event type:
All object create events
- Destination: Lambda function > Choose
MonitorCRR
- Name:
Create Lambda to Start/Stop EC2 with SNS
-
Go to Lambda > Create Function
- Name:
EC2ControlNotify
- Runtime: Python 3.10
- Role: Use
LambdaAutomationRole
- Name:
Paste this code:
import boto3
ec2 = boto3.client('ec2')
sns = boto3.client('sns')
def lambda_handler(event, context):
instance_id = 'i-xxxxxxxxxxxxxxxxx' # Replace with your EC2 instance ID
action = 'start' # or 'stop'
if action == 'start':
ec2.start_instances(InstanceIds=[instance_id])
message = f"Started EC2 instance {instance_id}"
else:
ec2.stop_instances(InstanceIds=[instance_id])
message = f"Stopped EC2 instance {instance_id}"
sns.publish(
TopicArn='arn:aws:sns:your-region:your-account-id:AlertTopic',
Message=message,
Subject=f'[EC2 Control] {action.upper()} action performed'
)
return {'status': 'success', 'message': message}
Click Deploy
(Optional) Trigger EC2 Lambda Automatically
If you want to start/stop EC2 on schedule:
- Go to CloudWatch > Rules > Create rule
- Event Source:
Schedule
- Example:
cron(0 18 * * ? *)
for 6 PM UTC daily
- Example:
- Target: Lambda function > Choose
EC2ControlNotify
Test Everything
- Upload an object to the source S3 bucket → Check your email for CRR alert.
- Run EC2 Lambda manually → Instance should start/stop and email should arrive.
- Check CloudWatch Logs for debugging.
Bonus Tip: Secure Your Setup
- Use environment variables in Lambda to avoid hardcoding instance IDs or SNS ARNs.
- Add logging and error handling.
- Use CloudTrail to audit changes.