Spring boot Ecommerce project frontend using ReactJS
1.Entity: package com.example.demo.entity; import jakarta.persistence.Entity; import jakarta.persistence.GeneratedValue; import jakarta.persistence.GenerationType; import jakarta.persistence.Id; import jakarta.persistence.Table; import lombok.AllArgsConstructor; import lombok.Getter; import lombok.NoArgsConstructor; @Entity @NoArgsConstructor @AllArgsConstructor @Getter @Table(name="product") public class Product { @Id @GeneratedValue(strategy=GenerationType.IDENTITY) private Long id; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } public String getImg() { return img; } public void setImg(String img) { this.img = img; } public Integer getRating() { return rating; } public void setRating(Integer rating) { this.rating = rating; } public Integer getStock() { return stock; } public void setStock(Integer stock) { this.stock = stock; } public Long getPrice() { return price; } public void setPrice(Long price) { this.price = price; } private String name, description, img; private Integer rating, stock; private Long price; } 2.repository: package com.example.demo.repository; import org.springframework.data.jpa.repository.JpaRepository; import org.springframework.stereotype.Repository; import com.example.demo.entity.Product; @Repository public interface ProductRepository extends JpaRepository{ } 3.Service: package com.example.demo.service; import java.util.List; import org.springframework.stereotype.Service; import com.example.demo.entity.Product; import com.example.demo.repository.ProductRepository; @Service public class ProductService { private final ProductRepository repository; public ProductService(ProductRepository repository) { this.repository = repository; } public List getAllProducts() { return repository.findAll(); } public Product addProduct(Product product) { return repository.save(product); } } 4.Controller: package com.example.demo.controller; import java.util.List; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.example.demo.entity.Product; import com.example.demo.service.ProductService; @RestController @RequestMapping("/api/products") public class ProductController { private final ProductService service; public ProductController(ProductService service) { this.service = service; } @GetMapping public List showAllProducts() { return service.getAllProducts(); } @PostMapping public Product addProduct(@RequestBody Product product) { return service.addProduct(product); } } neelakandan@neelakandan-HP-Laptop-15s-eq2xxx:~$ sudo -i -u postgres [sudo] password for neelakandan: postgres@neelakandan-HP-Laptop-15s-eq2xxx:~$ psql psql (16.8 (Ubuntu 16.8-0ubuntu0.24.04.1)) Type "help" for help. postgres=# \l postgres=# \c ecommerce You are now connected to database "ecommerce" as user "postgres". ecommerce=# \d Did not find any relations. ecommerce=# GRANT ALL PRIVILEGES ON SCHEMA public TO ecom3; GRANT ecommerce=# \d List of relations Schema | Name | Type | Owner --------+----------------+----------+------- public | cart | table | ecom3 public | cart_id_seq | sequence | ecom3 public | payment | table | ecom3 public | payment_id_seq | sequence | ecom3 public | product | table | ecom3 public | product_id_seq | sequence | ecom3 (6 rows) ecommerce=# insert into product(name, description,url, rating, price, stock) values('prod-1', 'Good prod', 'link-1',4, 10000,10); ERROR: column "url" of relation "product" does not exist LINE 1: insert into product(name, description,url, rating, price, st... ^ ecommerce=# ^C ecommerce=# ^C ecommerce=# insert into product(name, description, url, rating, price, stock) values('prod-1', 'Good prod', 'link-1', 4, 10000, 10); ERROR: column "url" of relation "product" does not exist LINE 1: insert into product(name, description, url, rating, price, s... ^ ecommerce=# \d product ecommerce=# ^[[200~ALTER TABLE product ADD COLUMN url TEXT; ERROR: syntax error at or near " ecommerce=# ALTER TABLE product ADD COLU
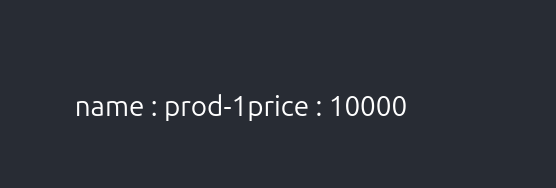
1.Entity:
package com.example.demo.entity;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.Table;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
@Entity
@NoArgsConstructor
@AllArgsConstructor
@Getter
@Table(name="product")
public class Product {
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private Long id;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getImg() {
return img;
}
public void setImg(String img) {
this.img = img;
}
public Integer getRating() {
return rating;
}
public void setRating(Integer rating) {
this.rating = rating;
}
public Integer getStock() {
return stock;
}
public void setStock(Integer stock) {
this.stock = stock;
}
public Long getPrice() {
return price;
}
public void setPrice(Long price) {
this.price = price;
}
private String name, description, img;
private Integer rating, stock;
private Long price;
}
2.repository:
package com.example.demo.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.example.demo.entity.Product;
@Repository
public interface ProductRepository extends
JpaRepository{
}
3.Service:
package com.example.demo.service;
import java.util.List;
import org.springframework.stereotype.Service;
import com.example.demo.entity.Product;
import com.example.demo.repository.ProductRepository;
@Service
public class ProductService {
private final ProductRepository repository;
public ProductService(ProductRepository repository)
{
this.repository = repository;
}
public List getAllProducts()
{
return repository.findAll();
}
public Product addProduct(Product product)
{
return repository.save(product);
}
}
4.Controller:
package com.example.demo.controller;
import java.util.List;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.example.demo.entity.Product;
import com.example.demo.service.ProductService;
@RestController
@RequestMapping("/api/products")
public class ProductController {
private final ProductService service;
public ProductController(ProductService service)
{
this.service = service;
}
@GetMapping
public List showAllProducts()
{
return service.getAllProducts();
}
@PostMapping
public Product addProduct(@RequestBody Product product)
{
return service.addProduct(product);
}
}
neelakandan@neelakandan-HP-Laptop-15s-eq2xxx:~$ sudo -i -u postgres
[sudo] password for neelakandan:
postgres@neelakandan-HP-Laptop-15s-eq2xxx:~$ psql
psql (16.8 (Ubuntu 16.8-0ubuntu0.24.04.1))
Type "help" for help.
postgres=# \l
postgres=# \c ecommerce
You are now connected to database "ecommerce" as user "postgres".
ecommerce=# \d
Did not find any relations.
ecommerce=# GRANT ALL PRIVILEGES ON SCHEMA public TO ecom3;
GRANT
ecommerce=# \d
List of relations
Schema | Name | Type | Owner
--------+----------------+----------+-------
public | cart | table | ecom3
public | cart_id_seq | sequence | ecom3
public | payment | table | ecom3
public | payment_id_seq | sequence | ecom3
public | product | table | ecom3
public | product_id_seq | sequence | ecom3
(6 rows)
ecommerce=# insert into product(name, description,url, rating, price, stock)
values('prod-1', 'Good prod', 'link-1',4, 10000,10);
ERROR: column "url" of relation "product" does not exist
LINE 1: insert into product(name, description,url, rating, price, st...
^
ecommerce=# ^C
ecommerce=# ^C
ecommerce=# insert into product(name, description, url, rating, price, stock)
values('prod-1', 'Good prod', 'link-1', 4, 10000, 10);
ERROR: column "url" of relation "product" does not exist
LINE 1: insert into product(name, description, url, rating, price, s...
^
ecommerce=# \d product
ecommerce=# ^[[200~ALTER TABLE product ADD COLUMN url TEXT;
ERROR: syntax error at or near "
ecommerce=# ALTER TABLE product ADD COLUMN url TEXT;//alter table
ALTER TABLE
ecommerce=# insert into product(name, description, url, rating, price, stock)
values('prod-1', 'Good prod', 'link-1', 4, 10000, 10);
INSERT 0 1
ecommerce=# \d//table
public | cart | table | ecom3
public | cart_id_seq | sequence | ecom3
public | payment | table | ecom3
public | payment_id_seq | sequence | ecom3
public | product | table | ecom3
public | product_id_seq | sequence | ecom3
ecommerce=# \c//conect database
You are now connected to database "ecommerce" as user "postgres".
ecommerce=# \l
ecommerce=# SELECT * FROM product;//inside table
1 | Good prod | | prod-1 | 10000 | 4 | 10 | link-1
ecommerce=# \c product_cad ;
You are now connected to database "product_cad" as user "postgres".
**product_cad=# drop database ecommerce**;
ERROR: database "ecommerce" is being accessed by other users
DETAIL: There are 10 other sessions using the database.
**API Endpoint URL** :localhost:8084/api/products
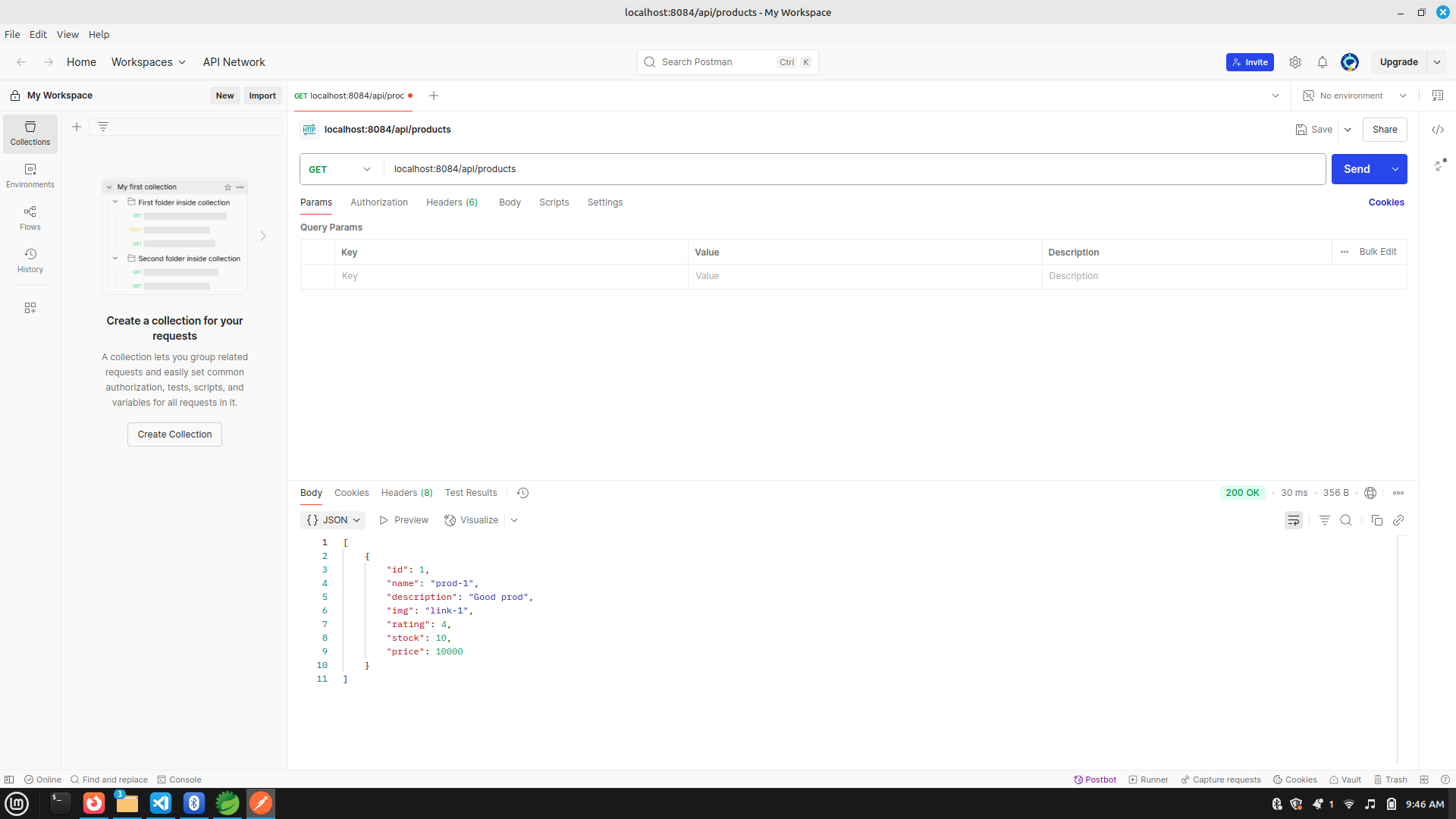
In a React application, you can send data to a backend server (like a Spring Boot API) using a POST request. To do this, we use the fetch() method or a library like axios. In the example above, we created a simple form that accepts a product's name and price. When the form is submitted, the handleSubmit function is called. Inside handleSubmit, we send a POST request to http://localhost:8084/api/products, with the form data converted into JSON format. The server is expected to accept this data, save it (e.g., into a database), and return a response. To allow this, the Spring Boot backend must have a controller method with @PostMapping("/api/products") and accept the incoming JSON using @RequestBody. This setup allows the React frontend and Spring Boot backend to communicate, making it possible to add new products dynamically from the user interface.
**Create file called API.js and base below code **
import axios from "axios";
export const getProducts = ()=> axios.get("http://localhost:8084/api/products");
*Create file called APP.js and base below code *
import logo from './logo.svg';
import './App.css';
import ProductCard from './components/ProductCard';
import { getProducts } from './api';
import { useEffect, useState } from 'react';
function App() {
const [productList,setProductList] = useState([]);
useEffect(()=>{
getProducts()
.then((res)=>setProductList(res.data))
.catch((err)=>console.log(err));
},[])
return (
{
productList.map((prod)=>)
//
}
);
}
export default App;
Create file component file and create file called name.jsx
function ProductCard({items})
{
return(
name : {items.name}
price : {items.price}
)
}
export default ProductCard;