Single Responsibility Principle (SRP) in MuleSoft: Structuring Flows with Focused Responsibilities
What is SRP? The Single Responsibility Principle (SRP) states that a component should have only one reason to change. In practical terms, this means that each part of a system should be dedicated to a single task or specific function. When applied to MuleSoft, SRP helps us design specialized flows and subflows, each focused on a well-defined responsibility. This results in systems that are more organized, easier to maintain, and scalable. Why is SRP Crucial in Integrations? Imagine an order system with a monolithic flow performing the following tasks: Validating order data (e.g., checking if orderId exists). Calculating the total order value based on items and quantities. Sending data to an external ERP system. Notifying the customer via SMS or email after processing. This flow violates SRP because it mixes multiple responsibilities in one place, making it difficult to understand, test, and modify. Any change in one of these responsibilities can impact the entire flow. By applying SRP, we can divide these responsibilities into dedicated subflows, making the system more modular and manageable. How to Apply SRP in the Order System? 1. Divide Flows into Specialized Subflows In MuleSoft, responsibilities can be divided into dedicated subflows: One subflow exclusively for validating order data. Another for calculating the total order value. A third for sending data to the ERP system. Practical Example – Before (violating SRP): Practical Example – After (applying SRP): 2. Organize Files in a Structured Way In addition to logically dividing responsibilities, it is important to organize files physically to facilitate maintenance and scalability. Recommended File Structure Main File: order-processing-api.xml Contains the main flow orchestrating the subflows. Subflows File: order-subflows.xml Groups all subflows related to orders. Utility File: common-utils.xml Contains reusable generic subflows such as error handling or logging. Example Structure src/ |-- main/ | |-- mule/ | |-- order-processing-api.xml | |-- order-subflows.xml | |-- common-utils.xml 3. Reuse Subflows with Shared Libraries Specialized subflows can be reused across different APIs or projects using Shared Libraries in MuleSoft. Benefits of Shared Libraries Avoid code duplication by allowing reuse of generic subflows (e.g., validation or error handling). Facilitate centralized updates without impacting multiple projects. How to Create Shared Libraries Publish generic subflows in the Anypoint Exchange as reusable modules. Import these modules into other projects as needed. For more details, see MuleSoft's official documentation on Shared Libraries. Benefits of SRP in the Order System Readability: Smaller flows are easier to understand and document. Reusability: Specialized subflows can be used across multiple APIs or future projects (e.g., validation can be reused in stock-related APIs). Simplified Maintenance: Changes in one subflow do not impact other components. Checklist for Applying SRP in MuleSoft [ ] Does each flow/subflow perform only one specific task? [ ] Are reusable subflows created for common tasks? [ ] Is the project structure conducive to maintenance and scalability? Next Steps Now that we’ve covered SRP, let’s move on to the next principle in the series: the Open/Closed Principle (OCP) — designing APIs and flows that evolve without breaking existing functionalities. ← Introduction | → Next Principle: OCP SOLID in MuleSoft – The Art of Designing Evolutionary Integrations Complete Series: Introduction Single Responsibility Principle (SRP) Open/Closed Principle (OCP) Liskov Substitution Principle (LSP) Interface Segregation Principle (ISP) Dependency Inversion Principle (DIP)
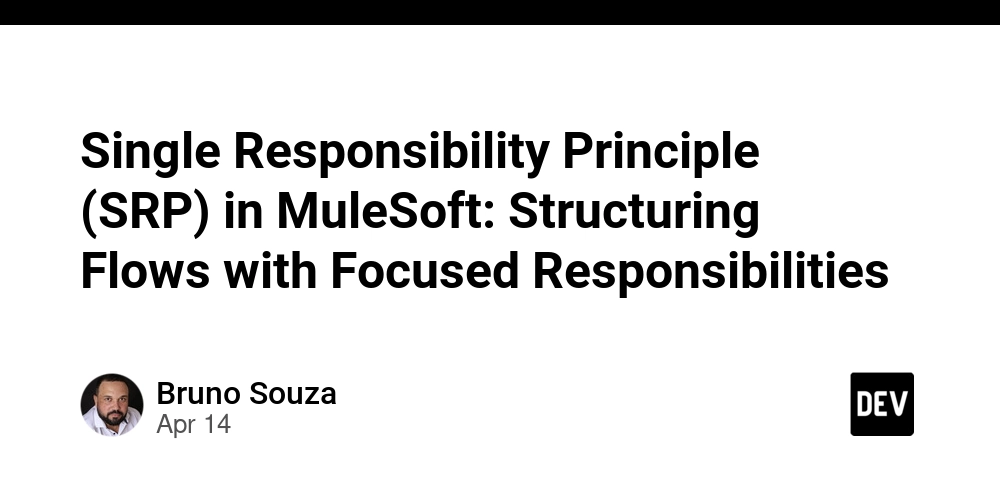
What is SRP?
The Single Responsibility Principle (SRP) states that a component should have only one reason to change. In practical terms, this means that each part of a system should be dedicated to a single task or specific function.
When applied to MuleSoft, SRP helps us design specialized flows and subflows, each focused on a well-defined responsibility. This results in systems that are more organized, easier to maintain, and scalable.
Why is SRP Crucial in Integrations?
Imagine an order system with a monolithic flow performing the following tasks:
- Validating order data (e.g., checking if
orderId
exists). - Calculating the total order value based on items and quantities.
- Sending data to an external ERP system.
- Notifying the customer via SMS or email after processing.
This flow violates SRP because it mixes multiple responsibilities in one place, making it difficult to understand, test, and modify. Any change in one of these responsibilities can impact the entire flow.
By applying SRP, we can divide these responsibilities into dedicated subflows, making the system more modular and manageable.
How to Apply SRP in the Order System?
1. Divide Flows into Specialized Subflows
In MuleSoft, responsibilities can be divided into dedicated subflows:
- One subflow exclusively for validating order data.
- Another for calculating the total order value.
- A third for sending data to the ERP system.
Practical Example – Before (violating SRP):
name="processOrder">
path="/orders"/>
expression="#[payload.orderId == null]">
value="Order ID is missing"/>
doc:name="Calculate Total">
method="POST" url="https://erp.example.com/api/orders"/>
Practical Example – After (applying SRP):
name="processOrder">
path="/orders"/>
name="validateOrder"/>
name="calculateTotal"/>
name="sendToERP"/>
name="validateOrder">
expression="#[payload.orderId == null]">
value="Order ID is missing"/>
name="calculateTotal">
doc:name="Calculate Total">
name="sendToERP">
method="POST" url="https://erp.example.com/api/orders"/>
2. Organize Files in a Structured Way
In addition to logically dividing responsibilities, it is important to organize files physically to facilitate maintenance and scalability.
Recommended File Structure
-
Main File:
order-processing-api.xml
- Contains the main flow orchestrating the subflows.
-
Subflows File:
order-subflows.xml
- Groups all subflows related to orders.
-
Utility File:
common-utils.xml
- Contains reusable generic subflows such as error handling or logging.
Example Structure
src/
|-- main/
| |-- mule/
| |-- order-processing-api.xml
| |-- order-subflows.xml
| |-- common-utils.xml
3. Reuse Subflows with Shared Libraries
Specialized subflows can be reused across different APIs or projects using Shared Libraries in MuleSoft.
Benefits of Shared Libraries
- Avoid code duplication by allowing reuse of generic subflows (e.g., validation or error handling).
- Facilitate centralized updates without impacting multiple projects.
How to Create Shared Libraries
- Publish generic subflows in the Anypoint Exchange as reusable modules.
- Import these modules into other projects as needed.
For more details, see MuleSoft's official documentation on Shared Libraries.
Benefits of SRP in the Order System
- Readability: Smaller flows are easier to understand and document.
- Reusability: Specialized subflows can be used across multiple APIs or future projects (e.g., validation can be reused in stock-related APIs).
- Simplified Maintenance: Changes in one subflow do not impact other components.
Checklist for Applying SRP in MuleSoft
- [ ] Does each flow/subflow perform only one specific task?
- [ ] Are reusable subflows created for common tasks?
- [ ] Is the project structure conducive to maintenance and scalability?
Next Steps
Now that we’ve covered SRP, let’s move on to the next principle in the series: the Open/Closed Principle (OCP) — designing APIs and flows that evolve without breaking existing functionalities.
← Introduction | → Next Principle: OCP