Simplifying API Promise Handling in Next.js 15
In modern Next.js 15 applications, API routes often deal with asynchronous operations like database queries, authentication, and data fetching. Handling these properly requires structured error management to avoid unexpected crashes. In our previous blogs, we built: A structured API response helper → serverResponse Read here A request body parser → getJsonBodyData Read here Now, we’ll take it one step further by creating a universal API promise resolver to eliminate try-catch repetition across all API routes. Why Use a Promise Resolver for API Routes? ❌ Without it, every API function needs a try-catch block, leading to redundant code. ❌ Error handling becomes inconsistent, making debugging harder. ❌ Missed edge cases might cause APIs to crash unexpectedly. ✅ With a reusable promise resolver, we centralize error handling, reduce boilerplate, and ensure clean API code. Building the serverAsyncResolve Helper in Next.js 15 Step 1: Creating the Promise Resolver Function Let’s build a universal try-catch wrapper for Next.js 15 API routes: import type { NextResponse } from "next/server"; import serverResponse from "@/helpers/server-helper/serverResponse"; import type { ServerResponseType } from "@/types"; export default async function serverAsyncResolve( asyncCallback: () => Promise, ): Promise { try { return await asyncCallback(); } catch (err) { if (err instanceof Error) { switch (err.name) { default: return serverResponse({ success: false, error: err.message, status: 500, }); } } return serverResponse({ success: false, error: "Something went wrong", status: 500, }); } }
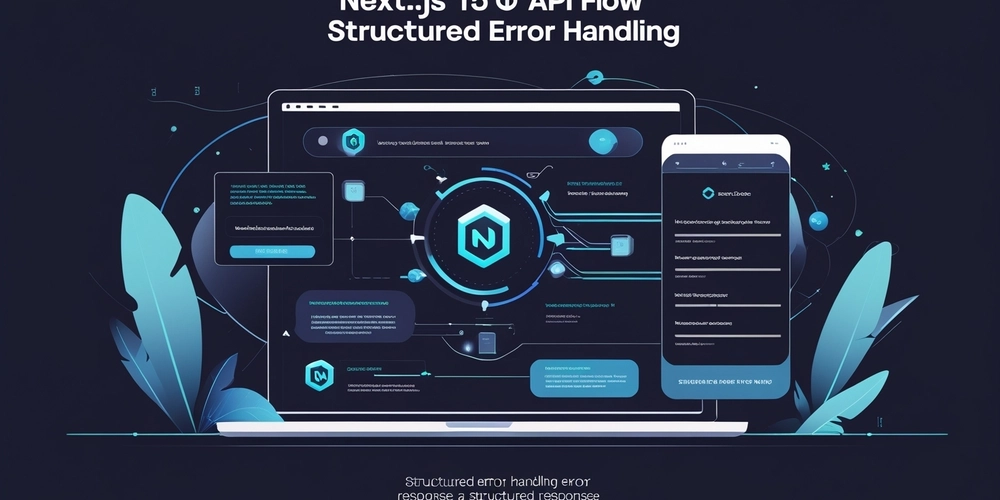
In modern Next.js 15 applications, API routes often deal with asynchronous operations like database queries, authentication, and data fetching. Handling these properly requires structured error management to avoid unexpected crashes.
In our previous blogs, we built:
- A structured API response helper →
serverResponse
Read here - A request body parser →
getJsonBodyData
Read here
Now, we’ll take it one step further by creating a universal API promise resolver to eliminate try-catch repetition across all API routes.
Why Use a Promise Resolver for API Routes?
❌ Without it, every API function needs a try-catch block, leading to redundant code.
❌ Error handling becomes inconsistent, making debugging harder.
❌ Missed edge cases might cause APIs to crash unexpectedly.
✅ With a reusable promise resolver, we centralize error handling, reduce boilerplate, and ensure clean API code.
Building the serverAsyncResolve
Helper in Next.js 15
Step 1: Creating the Promise Resolver Function
Let’s build a universal try-catch wrapper for Next.js 15 API routes:
import type { NextResponse } from "next/server";
import serverResponse from "@/helpers/server-helper/serverResponse";
import type { ServerResponseType } from "@/types";
export default async function serverAsyncResolve<T>(
asyncCallback: () => Promise<NextResponse<ServerResponseType<T>>>,
): Promise<NextResponse<ServerResponseType<T>>> {
try {
return await asyncCallback();
} catch (err) {
if (err instanceof Error) {
switch (err.name) {
default:
return serverResponse({
success: false,
error: err.message,
status: 500,
});
}
}
return serverResponse({
success: false,
error: "Something went wrong",
status: 500,
});
}
}