shallowCopy & deepCopy in JavaScript
ShallowCopy When we copy an object to a variable and then change the value using that variable, the original object's values also change. This happens because we're not copying the actual data—we're just copying the object's reference (the memory address). So, both the original object and the new variable point to the same data in memory. To avoid this, we use a shallow copy, which creates a new object with the same values, so changes to the new object don’t affect the original one Problem statement let obj = { name: "saurabh" }; let user = obj; name.name = "sachin"; console.log(obj); //output is sachin Solution 1. Solution let obj = { name: "saurabh" } let user = {...obj} user.name = "sachin" console.log(obj) //output will be saurabh 2. Solution let obj = { name: "saurabh" } let user = object.assign({},obj); user.name="sachin" console.log(obj) //output will be saurabh Deep Copy A shallow copy won't be enough if an object has nested objects (objects inside objects). That’s because a shallow copy only copies the top-level properties. The nested objects are still copied by reference. So, if we change a value inside a nested object in the copied version, it will still affect the original object. To avoid this, we use a deep copy, which copies everything, including all nested objects. This way, the copied object is completely separate from the original, and changes won’t affect each other. Problem statement let obj = { name: "Saurabh", address: { city: "Pune", state: "MH" } }; let user = {...obj} user.address.city = "Mumbai" console.log (obj) //output will be obj, city will be changed to Mumbai Solution let obj = { name: "Saurabh" address: { city: "Pune" State: "MH" } } let user = JSON.parse(JSON.Stringfy(obj)); user.address.city = "Mumbai" console.log(obj) // output remains the same as the obj will not change
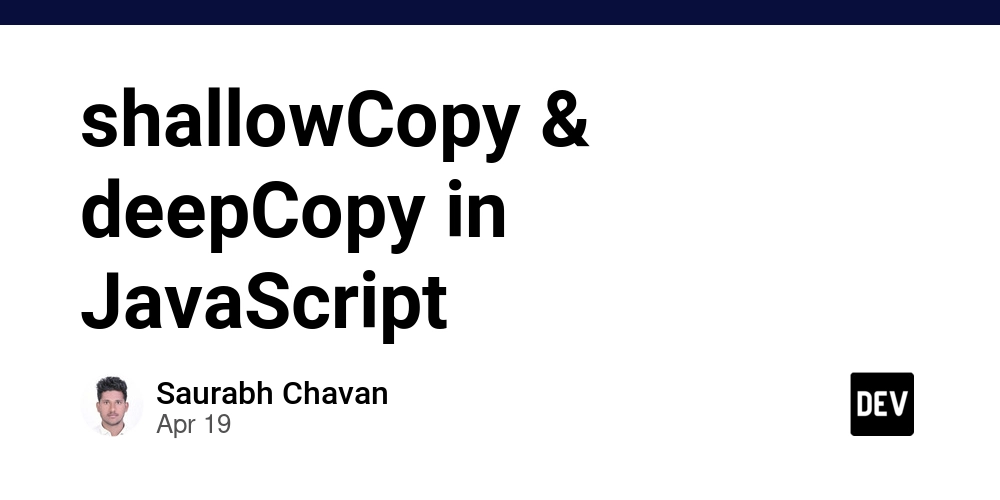
ShallowCopy
When we copy an object to a variable and then change the value using that variable, the original object's values also change. This happens because we're not copying the actual data—we're just copying the object's reference (the memory address). So, both the original object and the new variable point to the same data in memory.
To avoid this, we use a shallow copy, which creates a new object with the same values, so changes to the new object don’t affect the original one
Problem statement
let obj = {
name: "saurabh"
};
let user = obj;
name.name = "sachin";
console.log(obj); //output is sachin
Solution
1. Solution
let obj = {
name: "saurabh"
}
let user = {...obj}
user.name = "sachin"
console.log(obj) //output will be saurabh
2. Solution
let obj = {
name: "saurabh"
}
let user = object.assign({},obj);
user.name="sachin"
console.log(obj) //output will be saurabh
Deep Copy
A shallow copy won't be enough if an object has nested objects (objects inside objects). That’s because a shallow copy only copies the top-level properties. The nested objects are still copied by reference.
So, if we change a value inside a nested object in the copied version, it will still affect the original object.
To avoid this, we use a deep copy, which copies everything, including all nested objects. This way, the copied object is completely separate from the original, and changes won’t affect each other.
Problem statement
let obj = {
name: "Saurabh",
address: {
city: "Pune",
state: "MH"
}
};
let user = {...obj}
user.address.city = "Mumbai"
console.log (obj) //output will be obj, city will be changed to Mumbai
Solution
let obj = {
name: "Saurabh"
address: {
city: "Pune"
State: "MH"
}
}
let user = JSON.parse(JSON.Stringfy(obj));
user.address.city = "Mumbai"
console.log(obj) // output remains the same as the obj will not change