Setting Up ESLint and Prettier in Your React Project with Webpack and Babel (Continued)
In our previous post, we set up a React project from scratch using Webpack and Babel. Now, let's enhance our project by adding ESLint for code linting and Prettier for code formatting. These tools will ensure that our codebase remains consistent, readable, and error-free as it grows. What is ESLint? ESLint is a static code analysis tool that helps JavaScript developers find and fix problems in their code. It works by parsing your code using an Abstract Syntax Tree (AST). It uses the ESTree format for the AST structure, which is similar to Babel's AST. For example, the AST representation of a simple code like 1 + 2 would look like this: { "type": "ExpressionStatement", "expression": { "type": "BinaryExpression", "left": { "type": "Literal", "value": 1, "raw": "1" }, "operator": "+", "right": { "type": "Literal", "value": 2, "raw": "2" } } } With this AST structure, tools like ESLint can easily detect violations in your code. How to Install ESLint in Your React Project Install ESLint as a dev dependency: npm install -D eslint Initialize ESLint: npx eslint --init This will prompt you with a series of questions to generate the eslint.config.js file. For a React project, ensure you select React as your framework. Sample ESLint Config File (for React) Once you've run npx eslint --init, your eslint.config.js will look something like this: `import { defineConfig } from "eslint/config"; import js from "@eslint/js"; import globals from "globals"; import pluginReact from "eslint-plugin-react"; export default defineConfig([ { files: ["*/.{js,jsx}"], languageOptions: { globals: globals.browser }, plugins: { js }, extends: ["js/recommended"] }, pluginReact.configs.flat.recommended, ]); ` Let's break down the configuration: files: Specifies which files this config will apply to. Here it’s set to apply to all .js and .jsx files. languageOptions: Contains JavaScript-specific options, such as setting the global variables (globals.browser in this case). plugins: Specifies additional plugins to use for linting, such as the js plugin for general JavaScript linting. extends: This property tells ESLint to extend rules from eslint:recommended, a set of base rules that ESLint provides. Now that we have the basic setup for ESLint, let’s configure some custom rules to match our project’s requirements. Adding Custom ESLint Rules You may want to customize ESLint’s behavior for your specific use case. For example, we can disable the prop-types rule and turn off the need to import React in every JSX file for React 17+. Here's how to do it: export default defineConfig([ { files: ["**/*.{js,jsx}"], languageOptions: { globals: globals.browser }, plugins: { js }, extends: ["js/recommended"] }, { ...pluginReact.configs.flat.recommended, rules: { ...pluginReact.configs.flat.recommended.rules, "react/prop-types": "off", // Disable prop-types rule "react/react-in-jsx-scope": "off" // No need to import React in every file (React 17+) }, }, ]); Setting Up ESLint in VS Code To make sure ESLint works seamlessly in your editor, we’ll need to install the ESLint extension for VSCode. Install the ESLint Extension: Search for ESLint in the Extensions tab of VSCode and install it. Configure VSCode: Create a .vscode/settings.json file and add the following configuration: { "eslint.experimental.useFlatConfig": true, "eslint.validate": ["javascript", "javascriptreact"], "editor.codeActionsOnSave": { "source.fixAll.eslint": "explicit" } } This configuration ensures ESLint runs automatically every time you save your file. Ignoring Files with ESLint In ESLint, if you want to ignore certain files (like webpack.config.js), you should do it via the ignores property in your eslint.config.js instead of using .eslintignore (which is deprecated for flat config): ignores: ["webpack.config.js"] Setting Up Prettier Prettier is an opinionated code formatter that ensures consistency in your code. It integrates seamlessly with ESLint to format your code while also catching linting violations. Install Prettier: Install the necessary packages: npm install --save-dev prettier eslint-plugin-prettier eslint-config-prettier Create a .prettierrc File: This file will define your formatting rules. Here’s an example: { "singleQuote": true, "trailingComma": "all", "printWidth": 100, "semi": true } Integrate Prettier with ESLint: To ensure that Prettier’s rules are respected in ESLint, update your eslint.config.js file: `import { defineConfig } from 'eslint/config'; import js from '@eslint/js'; import globals from 'globals'; import pluginReact from 'eslint-plugin-react'; import eslintPluginPrettierRecommended from 'eslint-plugin-prettier/recommended'; import prettierConfig from 'eslint-config-prettier'; export default defineConfig([
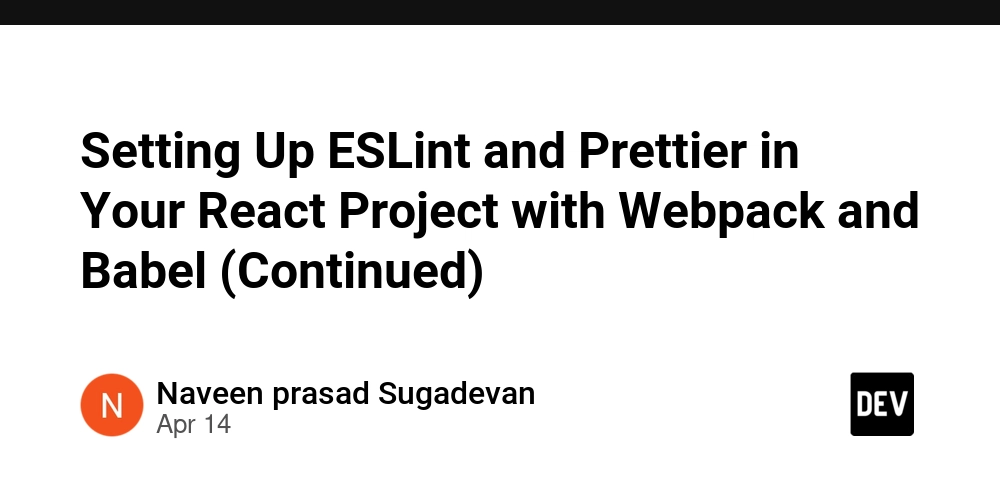
In our previous post, we set up a React project from scratch using Webpack and Babel. Now, let's enhance our project by adding ESLint for code linting and Prettier for code formatting. These tools will ensure that our codebase remains consistent, readable, and error-free as it grows.
What is ESLint?
ESLint is a static code analysis tool that helps JavaScript developers find and fix problems in their code. It works by parsing your code using an Abstract Syntax Tree (AST). It uses the ESTree format for the AST structure, which is similar to Babel's AST.
For example, the AST representation of a simple code like 1 + 2
would look like this:
{
"type": "ExpressionStatement",
"expression": {
"type": "BinaryExpression",
"left": {
"type": "Literal",
"value": 1,
"raw": "1"
},
"operator": "+",
"right": {
"type": "Literal",
"value": 2,
"raw": "2"
}
}
}
With this AST structure, tools like ESLint can easily detect violations in your code.
How to Install ESLint in Your React Project
- Install ESLint as a dev dependency:
npm install -D eslint
- Initialize ESLint:
npx eslint --init
This will prompt you with a series of questions to generate the eslint.config.js file. For a React project, ensure you select React as your framework.
Sample ESLint Config File (for React)
Once you've run npx eslint --init, your eslint.config.js will look something like this:
`import { defineConfig } from "eslint/config";
import js from "@eslint/js";
import globals from "globals";
import pluginReact from "eslint-plugin-react";
export default defineConfig([
{ files: ["*/.{js,jsx}"], languageOptions: { globals: globals.browser }, plugins: { js }, extends: ["js/recommended"] },
pluginReact.configs.flat.recommended,
]);
`
Let's break down the configuration:
files: Specifies which files this config will apply to. Here it’s set to apply to all .js and .jsx files.
languageOptions: Contains JavaScript-specific options, such as setting the global variables (globals.browser in this case).
plugins: Specifies additional plugins to use for linting, such as the js plugin for general JavaScript linting.
extends: This property tells ESLint to extend rules from eslint:recommended, a set of base rules that ESLint provides.
Now that we have the basic setup for ESLint, let’s configure some custom rules to match our project’s requirements.
Adding Custom ESLint Rules
You may want to customize ESLint’s behavior for your specific use case. For example, we can disable the prop-types rule and turn off the need to import React in every JSX file for React 17+.
Here's how to do it:
export default defineConfig([
{
files: ["**/*.{js,jsx}"],
languageOptions: { globals: globals.browser },
plugins: { js },
extends: ["js/recommended"]
},
{
...pluginReact.configs.flat.recommended,
rules: {
...pluginReact.configs.flat.recommended.rules,
"react/prop-types": "off", // Disable prop-types rule
"react/react-in-jsx-scope": "off" // No need to import React in every file (React 17+)
},
},
]);
Setting Up ESLint in VS Code
To make sure ESLint works seamlessly in your editor, we’ll need to install the ESLint extension for VSCode.
- Install the ESLint Extension: Search for ESLint in the Extensions tab of VSCode and install it.
- Configure VSCode:
Create a .vscode/settings.json file and add the following
configuration:
{ "eslint.experimental.useFlatConfig": true, "eslint.validate": ["javascript", "javascriptreact"], "editor.codeActionsOnSave": { "source.fixAll.eslint": "explicit" } }
This configuration ensures ESLint runs automatically every time you save your file.
Ignoring Files with ESLint
In ESLint, if you want to ignore certain files (like webpack.config.js), you should do it via the ignores property in your eslint.config.js instead of using .eslintignore (which is deprecated for flat config):
ignores: ["webpack.config.js"]
Setting Up Prettier
Prettier is an opinionated code formatter that ensures consistency in your code. It integrates seamlessly with ESLint to format your code while also catching linting violations.
Install Prettier:
Install the necessary packages:
npm install --save-dev prettier eslint-plugin-prettier eslint-config-prettier
Create a .prettierrc File:
This file will define your formatting rules. Here’s an example:
{
"singleQuote": true,
"trailingComma": "all",
"printWidth": 100,
"semi": true
}
Integrate Prettier with ESLint:
To ensure that Prettier’s rules are respected in ESLint, update your eslint.config.js file:
`import { defineConfig } from 'eslint/config';
import js from '@eslint/js';
import globals from 'globals';
import pluginReact from 'eslint-plugin-react';
import eslintPluginPrettierRecommended from 'eslint-plugin-prettier/recommended';
import prettierConfig from 'eslint-config-prettier';
export default defineConfig([
{
files: ['*/.{js,jsx}'],
ignores: ['webpack.config.js'],
languageOptions: { globals: globals.browser },
plugins: { js },
extends: ['js/recommended'],
},
{
...pluginReact.configs.flat.recommended,
rules: {
...pluginReact.configs.flat.recommended.rules,
'react/prop-types': 'off', // Turn off prop-types rule
'react/react-in-jsx-scope': 'off', // React import no longer required
'react/jsx-key': 'error', // Enforce keys in lists
},
},
eslintPluginPrettierRecommended,
prettierConfig,
]);
`
Configure VSCode for Prettier
To format code automatically with Prettier in VSCode, you need to install the Prettier extension:
Install the Prettier Extension:
Search for Prettier - Code formatter in the VSCode Extensions marketplace and install it.
Set Prettier as the Default Formatter:
Add the following to your .vscode/settings.json:
{
"editor.defaultFormatter": "esbenp.prettier-vscode"
}
Adding Lint and Format Scripts to package.json
Now that we have everything set up, let’s add some useful scripts to our package.json to make it easier to run ESLint and Prettier checks:
"scripts": {
"lint": "eslint .",
"lint:fix": "eslint . --fix",
}
Conclusion
In this post, we’ve walked through how to set up ESLint and Prettier in a React project created without CRA (using Webpack and Babel). We also covered how to:
- Install and configure ESLint for React.
- Add custom ESLint rules.
- Integrate Prettier with ESLint to auto-format code.
- Set up VSCode to work seamlessly with both ESLint and Prettier.
- Add useful scripts to package.json for easy linting and formatting.