Recursion in JavaScript
Recursion is a powerful programming concept and when we fully understand, it can greatly enhance our problem solving skills. Though it can seem intimidating at first, especially for beginners, recursion is a concept that is way simpler than it looks. In this post, let's explore what recursion is, why it's useful, and how we can start implementing it in JavaScript. What is Recursion? Recursion is a technique where a function calls itself to solve a problem. A method of breaking down a larger task into smaller sub tasks. When a function calls itself, it’s usually solving a smaller instance of the same problem until it reaches a base case, which is a condition where the recursion stops. For example, think of it like a set of Russian nesting dolls: you open one to find another smaller doll inside, and you repeat this process until you reach the smallest doll. The Basic Structure of Recursion A recursive function generally has two parts: ~Base case: This is the condition that ends the recursion. Without a base case, a recursive function will call itself indefinitely and cause a stack overflow which is a program crash. ~Recursive case: This is the part where the function calls itself with adjusted arguments, that gradually move towards the base case. function factorial(n) { // Base case if (n === 0) { return 1; } // Recursive case return n * factorial(n - 1); } console.log(factorial(5)); // Output: 120 In this function: *The base case is n === 0 where the factorial of 0 is defined as 1. *The recursive case is the function calling itself with n - 1, reducing the problem until it hits the base case. Why is Recursion Useful? Recursion is a refined way of solving problems that have a repetitive structure. For example: Recursion is great for problems like calculating factorials, Fibonacci sequences , as well as traversing mathematical functions that will repeat themselves in smaller units. Let’s take a look at a classic example: calculating the Fibonacci sequence. The Fibonacci sequence is defined as: F(0) = 0 F(1) = 1 F(n) = F(n-1) + F(n-2) for n > 1 *In JavaScript we can execute this as: function fibonacci(n) { // Base cases if (n === 0) return 0; if (n === 1) return 1; // Recursive case return fibonacci(n - 1) + fibonacci(n - 2); } console.log(fibonacci(6)); // Output: 8 *Here every call to fibonacci calculates smaller & smaller versions of the problem until it reaches the base cases (n === 0 or n === 1). Imagine you are standing in front of two mirrors facing each other. You see an endless series of reflections within reflections. Essentially this is what recursion does: each function call reflects itself into a smaller problem until a base case is reached, where the process stops. This analogy helps explain the sometimes “infinite” nature of recursion, as long as there is a condition, the base case, to fully stop the process. ~What Can Go Wrong?~ Even though recursion is a powerful tool, there are potential danger issues: ~Stack Overflow: If the base case is not correctly defined, or the function does not reduce the problem size sufficiently in every recursive call, the recursion could go on indefinitely and cause a stack overflow. Which is a situation where the call stack exceeds its limit and crashes the program. ~Efficiency: Recursive solutions are often less efficient than iterative solutions because every recursive call adds a layer to the stack. With problems like the Fibonacci sequence, a poorly structured recursive solution can repeat calculations many times, leading to unnecessary overhead. Here's an example of this inefficiency: function fibonacci(n) { if (n === 0) return 0; if (n === 1) return 1; return fibonacci(n - 1) + fibonacci(n - 2); // Inefficient for large n } console.log(fibonacci(50)); // This takes a long time *In cases like this, using memoization approach drastically improves performance. Memoization stores the results of expensive function calls and will return the cached result when the same inputs occur again. ~Improving Efficiency with Memoization~ Memoization involves storing the result of a function call so that if the function is called again with the same arguments, the cached result is returned, instead of recalculating it. Here’s how we can apply memoization to the Fibonacci function: function fibonacci(n, memo = {}) { if (n in memo) return memo[n]; // Return cached result if (n === 0) return 0; if (n === 1) return 1; // Store the result in the memo object memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo); return memo[n]; } console.log(fibonacci(50)); // Now it's much faster *By using memoization, we store the Fibonacci numbers as they are calculated, and the next time we need them, we can simply look them up in the memo object. ~Embrace the Power of Recursion~ If you're just starting with recursion, don’t worry if it feels strange at first. With pract
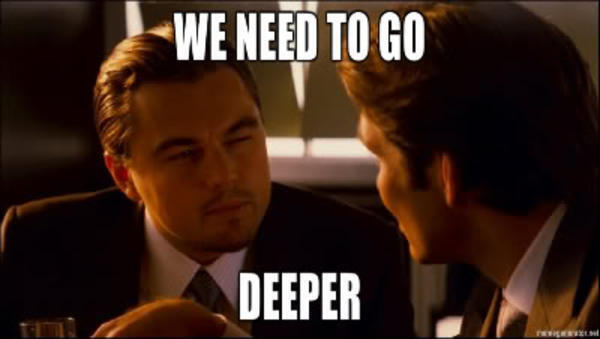
Recursion is a powerful programming concept and when we fully understand, it can greatly enhance our problem solving skills. Though it can seem intimidating at first, especially for beginners, recursion is a concept that is way simpler than it looks. In this post, let's explore what recursion is, why it's useful, and how we can start implementing it in JavaScript.
What is Recursion?
Recursion is a technique where a function calls itself to solve a problem. A method of breaking down a larger task into smaller sub tasks. When a function calls itself, it’s usually solving a smaller instance of the same problem until it reaches a base case, which is a condition where the recursion stops.
For example, think of it like a set of Russian nesting dolls: you open one to find another smaller doll inside, and you repeat this process until you reach the smallest doll.
The Basic Structure of Recursion
A recursive function generally has two parts:
~Base case: This is the condition that ends the recursion. Without a base case, a recursive function will call itself indefinitely and cause a stack overflow which is a program crash.
~Recursive case: This is the part where the function calls itself with adjusted arguments, that gradually move towards the base case.
function factorial(n) {
// Base case
if (n === 0) {
return 1;
}
// Recursive case
return n * factorial(n - 1);
}
console.log(factorial(5)); // Output: 120
In this function:
*The base case is n === 0
where the factorial of 0 is defined as 1.
*The recursive case is the function calling itself with n - 1
, reducing the problem until it hits the base case.
Why is Recursion Useful?
Recursion is a refined way of solving problems that have a repetitive structure. For example:
Recursion is great for problems like calculating factorials, Fibonacci sequences , as well as traversing mathematical functions that will repeat themselves in smaller units.
Let’s take a look at a classic example: calculating the Fibonacci sequence. The Fibonacci sequence is defined as:
F(0) = 0
F(1) = 1
F(n) = F(n-1) + F(n-2) for n > 1
*In JavaScript we can execute this as:
function fibonacci(n) {
// Base cases
if (n === 0) return 0;
if (n === 1) return 1;
// Recursive case
return fibonacci(n - 1) + fibonacci(n - 2);
}
console.log(fibonacci(6)); // Output: 8
*Here every call to fibonacci calculates smaller & smaller versions of the problem until it reaches the base cases
(n === 0 or n === 1)
.
Imagine you are standing in front of two mirrors facing each other. You see an endless series of reflections within reflections. Essentially this is what recursion does: each function call reflects itself into a smaller problem until a base case is reached, where the process stops. This analogy helps explain the sometimes “infinite” nature of recursion, as long as there is a condition, the base case, to fully stop the process.
~What Can Go Wrong?~
Even though recursion is a powerful tool, there are potential danger issues:
~Stack Overflow: If the base case is not correctly defined, or the function does not reduce the problem size sufficiently in every recursive call, the recursion could go on indefinitely and cause a stack overflow. Which is a situation where the call stack exceeds its limit and crashes the program.
~Efficiency: Recursive solutions are often less efficient than iterative solutions because every recursive call adds a layer to the stack. With problems like the Fibonacci sequence, a poorly structured recursive solution can repeat calculations many times, leading to unnecessary overhead. Here's an example of this inefficiency:
function fibonacci(n) {
if (n === 0) return 0;
if (n === 1) return 1;
return fibonacci(n - 1) + fibonacci(n - 2); // Inefficient for large n
}
console.log(fibonacci(50)); // This takes a long time
*In cases like this, using memoization approach drastically improves performance. Memoization stores the results of expensive function calls and will return the cached result when the same inputs occur again.
~Improving Efficiency with Memoization~
Memoization involves storing the result of a function call so that if the function is called again with the same arguments, the cached result is returned, instead of recalculating it. Here’s how we can apply memoization to the Fibonacci function:
function fibonacci(n, memo = {}) {
if (n in memo) return memo[n]; // Return cached result
if (n === 0) return 0;
if (n === 1) return 1;
// Store the result in the memo object
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
console.log(fibonacci(50)); // Now it's much faster
*By using memoization, we store the Fibonacci numbers as they are calculated, and the next time we need them, we can simply look them up in the memo
object.
~Embrace the Power of Recursion~
If you're just starting with recursion, don’t worry if it feels strange at first. With practice, it will start to make more sense. The key to mastering recursion is to start with simple examples and understand the flow of function calls. Then gradually increase the complexity of the problems you solve.
In conclusion, recursion is not just a tool, it’s a way of thinking about problems in a recursive manner. Next time you encounter a problem, ask yourself: "Can I break this down into smaller instances of the same problem?" And if the answer is yes, then recursion might be your perfect solution.
SOURCES
MDN Web Docs
Medium
Stack Overflow
freeCodeCamp
codedamn
YouTube