Recursion
check palindrome: def is_palindrome(s): if len(s)
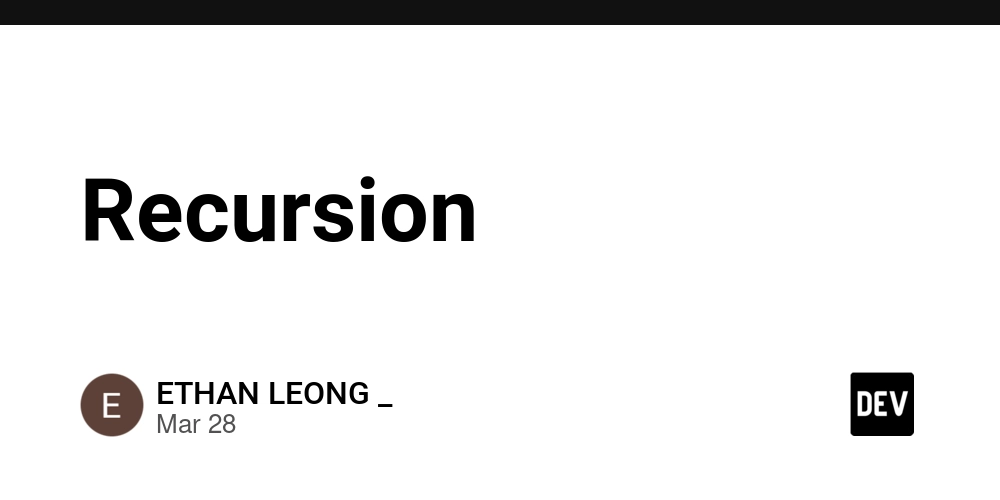
check palindrome:
def is_palindrome(s):
if len(s) <= 1:
return True
if s[0] != s[-1]:
return False
return is_palindrome(s[1:-1])
permutations of string
def permute(s, answer=''):
if len(s) == 0:
print(answer)
return
for i in range(len(s)):
ch = s[i]
left = s[:i]
right = s[i+1:]
permute(left + right, answer + ch)
subsets
def subsets(s, current=""):
if len(s) == 0:
print(current)
return
subsets(s[1:], current) # Exclude
subsets(s[1:], current + s[0]) # Include
tower of hanoi
def hanoi(n, source, target, auxiliary):
if n == 0:
return
hanoi(n - 1, source, auxiliary, target)
print(f"Move disk {n} from {source} to {target}")
hanoi(n - 1, auxiliary, target, source)
nth stair
def count_ways(n):
if n <= 1:
return 1
return count_ways(n - 1) + count_ways(n - 2)