React Server Components: The Future of React Performance
Introduction React has always been at the forefront of web development, making UI development efficient and scalable. One of the most exciting new features in React is React Server Components (RSC). This feature aims to improve performance by allowing certain components to run on the server instead of the client, reducing JavaScript bundle size and improving page load speeds. In this blog post, we’ll explore what React Server Components are, how they work, why they are beneficial, and how to integrate them into modern applications. What Are React Server Components? React Server Components (RSC) allow developers to write components that only run on the server, never being sent to the client. This means they don't increase the client-side JavaScript bundle size, leading to faster load times and improved performance. Key Problems Solved by RSCs: Large JavaScript Bundles: Traditional React apps send the entire component tree to the client, making initial loads slow. Data Fetching Inefficiencies: Fetching data in client components leads to unnecessary re-renders and poor user experience. SEO Challenges: Server-rendered content is more SEO-friendly compared to client-heavy React applications. Reduced Hydration Cost: Since server components don’t need hydration, client-side interactivity is reduced, leading to improved performance. How Do React Server Components Work? React now differentiates between three types of components: Server Components (.server.js) - Rendered on the server, never sent to the client. Client Components (.client.js) - Traditional React components that run in the browser. Shared Components - Can be used on both the server and client. Example of a Server Component // app/components/UserProfile.server.js export default async function UserProfile({ userId }) { const user = await fetch(`https://api.example.com/user/${userId}`).then(res => res.json()); return Welcome, {user.name}!; }
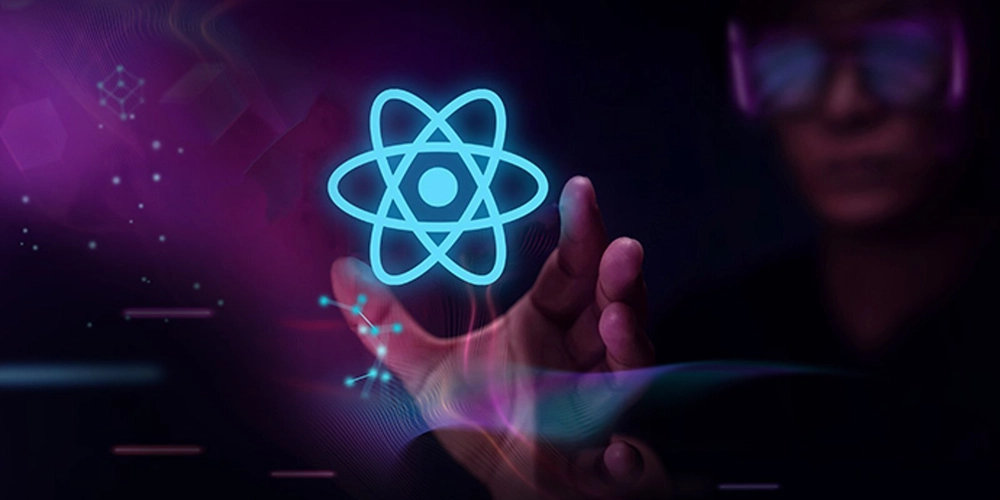
Introduction
React has always been at the forefront of web development, making UI development efficient and scalable. One of the most exciting new features in React is React Server Components (RSC). This feature aims to improve performance by allowing certain components to run on the server instead of the client, reducing JavaScript bundle size and improving page load speeds.
In this blog post, we’ll explore what React Server Components are, how they work, why they are beneficial, and how to integrate them into modern applications.
What Are React Server Components?
React Server Components (RSC) allow developers to write components that only run on the server, never being sent to the client. This means they don't increase the client-side JavaScript bundle size, leading to faster load times and improved performance.
Key Problems Solved by RSCs:
- Large JavaScript Bundles: Traditional React apps send the entire component tree to the client, making initial loads slow.
- Data Fetching Inefficiencies: Fetching data in client components leads to unnecessary re-renders and poor user experience.
- SEO Challenges: Server-rendered content is more SEO-friendly compared to client-heavy React applications.
- Reduced Hydration Cost: Since server components don’t need hydration, client-side interactivity is reduced, leading to improved performance.
How Do React Server Components Work?
React now differentiates between three types of components:
-
Server Components (
.server.js
) - Rendered on the server, never sent to the client. -
Client Components (
.client.js
) - Traditional React components that run in the browser. - Shared Components - Can be used on both the server and client.
Example of a Server Component
// app/components/UserProfile.server.js
export default async function UserProfile({ userId }) {
const user = await fetch(`https://api.example.com/user/${userId}`).then(res => res.json());
return <h1>Welcome, {user.name}!h1>;
}