React 19 Memoization: Is useMemo & useCallback No Longer Necessary?
React 19 Brings Automatic Optimization For years, React developers have relied on useMemo and useCallback to optimize performance and prevent unnecessary re-renders. However, React 19 introduces a game-changing feature: the React Compiler, which eliminates the need for manual memoization in most cases. In this article, we’ll explore how memoization worked before React 19, how the new React Compiler optimizes performance, and when (if ever) you still need useMemo and useCallback. The Problem with Manual Memoization What is Memoization in React? Memoization is a performance optimization technique that caches the results of expensive function calls, preventing redundant calculations when the same inputs occur again. Why Did We Use useMemo and useCallback Before React 19? React used to recreate functions and recalculate values on every render, even when unnecessary. To avoid performance issues, developers had to manually optimize their code by using: useMemo to memoize expensive calculations. useCallback to prevent unnecessary function re-creations. Example (Before React 19): import { useState, useMemo, useCallback } from "react"; function ExpensiveComponent({ num }) { const expensiveValue = useMemo(() => { console.log("Computing...!"); return num * 2; }, [num]); const handleClick = useCallback(() => { console.log("Button clicked!"); }, []); return ( Computed Value: {expensiveValue} Click Me ); } ✅ Optimization Done Manually: useMemo prevents recalculating expensiveValue on every render. useCallback ensures handleClick isn’t recreated unnecessarily.
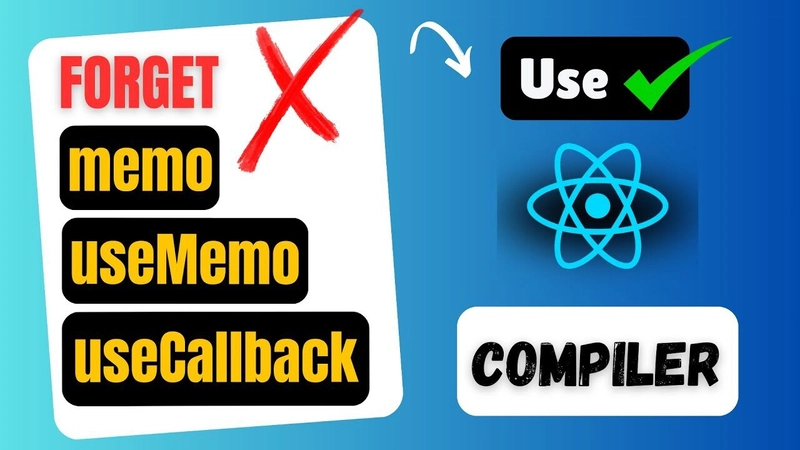
React 19 Brings Automatic Optimization
For years, React developers have relied on useMemo
and useCallback
to optimize performance and prevent unnecessary re-renders. However, React 19 introduces a game-changing feature: the React Compiler, which eliminates the need for manual memoization in most cases.
In this article, we’ll explore how memoization worked before React 19, how the new React Compiler optimizes performance, and when (if ever) you still need useMemo
and useCallback
.
The Problem with Manual Memoization
What is Memoization in React?
Memoization is a performance optimization technique that caches the results of expensive function calls, preventing redundant calculations when the same inputs occur again.
Why Did We Use useMemo
and useCallback
Before React 19?
React used to recreate functions and recalculate values on every render, even when unnecessary. To avoid performance issues, developers had to manually optimize their code by using:
-
useMemo
to memoize expensive calculations. -
useCallback
to prevent unnecessary function re-creations.
Example (Before React 19):
import { useState, useMemo, useCallback } from "react";
function ExpensiveComponent({ num }) {
const expensiveValue = useMemo(() => {
console.log("Computing...!");
return num * 2;
}, [num]);
const handleClick = useCallback(() => {
console.log("Button clicked!");
}, []);
return (
<div>
<p>Computed Value: {expensiveValue}p>
<button onClick={handleClick}>Click Mebutton>
div>
);
}
✅ Optimization Done Manually:
-
useMemo
prevents recalculatingexpensiveValue
on every render. -
useCallback
ensureshandleClick
isn’t recreated unnecessarily.