Performance in Vue.js: Breaking Down Conditional Code ⚡️
Introduction As Vue developers, we often strive to write clean and efficient code. But as components grow, especially with conditional rendering (v-if and v-else), things can quickly get messy. In this article, I’ll show you a simple technique that not only boosts performance but also improves the maintainability of large conditional blocks into separate components. The Problem Let’s say you have something like this in your template: At first, this works fine. But as the logic grows, this single file becomes harder to navigate, test, and maintain. The Solution: Split It Into Components Instead of stuffing everything into a single file, we can break down the v-if and v-else blocks into their own components. Now your template is clean, easy to read, and each part of the UI is in its own dedicated file. Performance Benefits Vue’s component-based architecture allows Vue to be smarter about updates. When you separate logic into components: Smaller render trees per component Lazy loading becomes possible (with defineAsyncComponent) Better reusability Better reusability Bonus Tip: Use Async Components You can go one step further by loading components only when needed: import { defineAsyncComponent } from 'vue'; export default { components: { AdminPanel: defineAsyncComponent(() => import('./AdminPanel.vue')), UserPanel: defineAsyncComponent(() => import('./UserPanel.vue')), }, }; This is especially useful if one side of the condition contains a lot of logic that’s rarely used (e.g., admin screens). Conclusion Breaking down conditional rendering into components is a small change with a big impact. It improves readability, boosts performance, and aligns with the core philosophy of Vue—building apps with small, reusable pieces. I hope you found it helpful. Leave a comment if you have used those directives before, and stay tuned for more Vue tips. Thanks for reading.
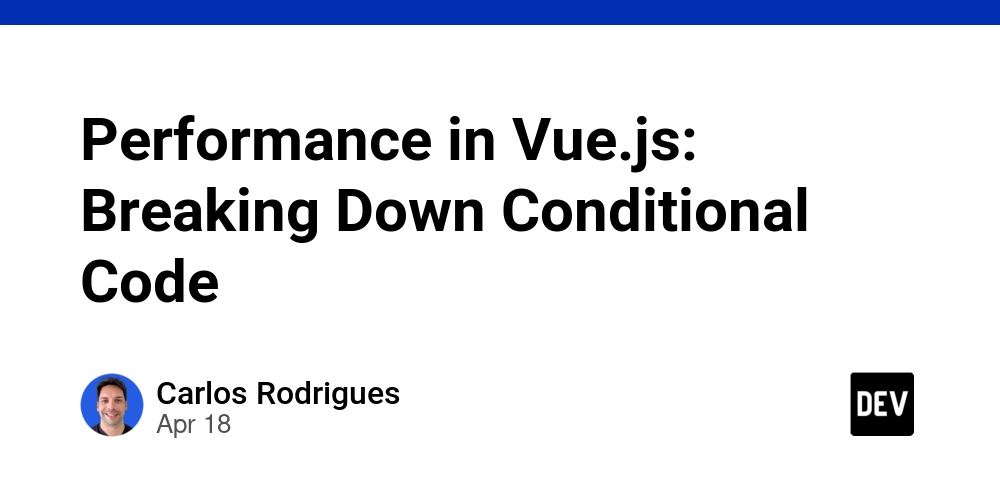
Introduction
As Vue developers, we often strive to write clean and efficient code. But as components grow, especially with conditional rendering (v-if
and v-else
), things can quickly get messy. In this article, I’ll show you a simple technique that not only boosts performance but also improves the maintainability of large conditional blocks into separate components.
The Problem
Let’s say you have something like this in your template:
v-if="isAdmin">
v-else>
At first, this works fine. But as the logic grows, this single file becomes harder to navigate, test, and maintain.
The Solution: Split It Into Components
Instead of stuffing everything into a single file, we can break down the v-if
and v-else
blocks into their own components.
v-if="isAdmin" />
v-else />
Now your template is clean, easy to read, and each part of the UI is in its own dedicated file.
Performance Benefits
Vue’s component-based architecture allows Vue to be smarter about updates. When you separate logic into components:
- Smaller render trees per component
- Lazy loading becomes possible (with defineAsyncComponent)
- Better reusability
- Better reusability
Bonus Tip: Use Async Components
You can go one step further by loading components only when needed:
import { defineAsyncComponent } from 'vue';
export default {
components: {
AdminPanel: defineAsyncComponent(() => import('./AdminPanel.vue')),
UserPanel: defineAsyncComponent(() => import('./UserPanel.vue')),
},
};
This is especially useful if one side of the condition contains a lot of logic that’s rarely used (e.g., admin screens).
Conclusion
Breaking down conditional rendering into components is a small change with a big impact. It improves readability, boosts performance, and aligns with the core philosophy of Vue—building apps with small, reusable pieces.
I hope you found it helpful. Leave a comment if you have used those directives before, and stay tuned for more Vue tips.
Thanks for reading.