As a developer, ensuring that your React app is optimized for performance, scalability, and user experience is crucial. Whether you're working on a small project or a large-scale application, the following best practices will help you create a more efficient and responsive React application. 1️⃣ Enhancing Rendering & Component Performance Rendering inefficiencies can significantly impact your app's performance. Here’s how you can optimize it: Use React.memo() to prevent unnecessary re-renders of functional components. Utilize useCallback() & useMemo() to optimize function and value references in child components. Implement Lazy Loading using React.lazy() & Suspense to reduce the initial bundle size and improve loading speeds. Avoid Inline Functions & Objects in JSX as they create new references on every render, leading to performance overhead. Leverage Automatic Batching in React 18+ to update multiple states in a single render pass, reducing re-renders. 2️⃣ Efficient State Management Managing state properly is essential for app scalability and performance. Use Context API Wisely – Avoid unnecessary re-renders by wrapping only relevant components in a context provider. Choose the Right State Management Solution – Consider Redux Toolkit, Zustand, or Recoil instead of excessive prop drilling. Minimize Unnecessary State Updates – Keep transient UI states (like modals, dropdowns) within local component state rather than global state. 3️⃣ Reducing Bundle Size & Optimizing Asset Loading Large bundle sizes can slow down page loads. Optimize your assets with these techniques: Enable Tree Shaking to remove unused code by properly structuring ES6 module imports. Use Code Splitting to load components dynamically using React.lazy() and import(). Optimize Images by using modern formats like WebP, lazy loading (loading="lazy"), and CDNs for faster delivery. Use SVG Instead of PNGs where applicable for scalable and lightweight graphics. Compress and Minify Files using tools like Terser, UglifyJS, and Brotli compression to reduce payload size. 4️⃣ Optimizing API Calls & Data Fetching Efficient data handling reduces unnecessary network requests and improves app responsiveness. Leverage SWR or React Query for API caching, pagination, and automatic background re-fetching. Debounce API Requests using lodash debounce() to minimize redundant network calls in search bars and fast user interactions. Use GraphQL to fetch only required data instead of over-fetching REST responses. Implement WebSockets for real-time updates instead of inefficient polling techniques. 5️⃣ Improving Page Load Speed & User Experience User experience is critical for retention and engagement. Here’s how to improve it: Preload Essential Resources using for critical assets like fonts and scripts. Remove Unused CSS & JavaScript with tools like PurgeCSS to avoid unnecessary bloat. Optimize Web Vitals by improving metrics such as Time to Interactive (TTI) and First Contentful Paint (FCP) with Lighthouse audits. Enable HTTP/2 & GZIP/Brotli Compression to accelerate network performance and reduce load times. 6️⃣ Debugging & Performance Monitoring Continuous monitoring and debugging help identify issues before they affect users. Use React Developer Tools to analyze component re-renders and identify bottlenecks. Monitor Performance with Lighthouse, WebPageTest, and React Profiler. Implement Error Boundaries to prevent app crashes and provide fallback UI when errors occur. Use Sentry or LogRocket to capture real-time errors and enhance debugging workflows.
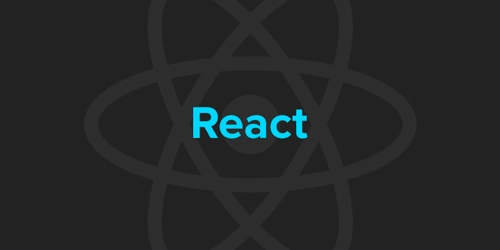
As a developer, ensuring that your React app is optimized for performance, scalability, and user experience is crucial. Whether you're working on a small project or a large-scale application, the following best practices will help you create a more efficient and responsive React application.
1️⃣ Enhancing Rendering & Component Performance
Rendering inefficiencies can significantly impact your app's performance. Here’s how you can optimize it:
-
Use
React.memo()
to prevent unnecessary re-renders of functional components. -
Utilize
useCallback()
&useMemo()
to optimize function and value references in child components. -
Implement Lazy Loading using
React.lazy()
&Suspense
to reduce the initial bundle size and improve loading speeds. - Avoid Inline Functions & Objects in JSX as they create new references on every render, leading to performance overhead.
- Leverage Automatic Batching in React 18+ to update multiple states in a single render pass, reducing re-renders.
2️⃣ Efficient State Management
Managing state properly is essential for app scalability and performance.
- Use Context API Wisely – Avoid unnecessary re-renders by wrapping only relevant components in a context provider.
- Choose the Right State Management Solution – Consider Redux Toolkit, Zustand, or Recoil instead of excessive prop drilling.
- Minimize Unnecessary State Updates – Keep transient UI states (like modals, dropdowns) within local component state rather than global state.
3️⃣ Reducing Bundle Size & Optimizing Asset Loading
Large bundle sizes can slow down page loads. Optimize your assets with these techniques:
- Enable Tree Shaking to remove unused code by properly structuring ES6 module imports.
-
Use Code Splitting to load components dynamically using
React.lazy()
andimport()
. -
Optimize Images by using modern formats like WebP, lazy loading (
loading="lazy"
), and CDNs for faster delivery. - Use SVG Instead of PNGs where applicable for scalable and lightweight graphics.
- Compress and Minify Files using tools like Terser, UglifyJS, and Brotli compression to reduce payload size.
4️⃣ Optimizing API Calls & Data Fetching
Efficient data handling reduces unnecessary network requests and improves app responsiveness.
- Leverage SWR or React Query for API caching, pagination, and automatic background re-fetching.
-
Debounce API Requests using lodash
debounce()
to minimize redundant network calls in search bars and fast user interactions. - Use GraphQL to fetch only required data instead of over-fetching REST responses.
- Implement WebSockets for real-time updates instead of inefficient polling techniques.
5️⃣ Improving Page Load Speed & User Experience
User experience is critical for retention and engagement. Here’s how to improve it:
-
Preload Essential Resources using
for critical assets like fonts and scripts.
- Remove Unused CSS & JavaScript with tools like PurgeCSS to avoid unnecessary bloat.
- Optimize Web Vitals by improving metrics such as Time to Interactive (TTI) and First Contentful Paint (FCP) with Lighthouse audits.
- Enable HTTP/2 & GZIP/Brotli Compression to accelerate network performance and reduce load times.
6️⃣ Debugging & Performance Monitoring
Continuous monitoring and debugging help identify issues before they affect users.
- Use React Developer Tools to analyze component re-renders and identify bottlenecks.
- Monitor Performance with Lighthouse, WebPageTest, and React Profiler.
- Implement Error Boundaries to prevent app crashes and provide fallback UI when errors occur.
- Use Sentry or LogRocket to capture real-time errors and enhance debugging workflows.