Neo4j Fundamentals: Introduction to Graph Databases
Introduction Welcome to the world of graph databases! This tutorial is based on the first video in the "Neo4j Graph Database Tutorial" series and provides a comprehensive introduction to Neo4j and graph database concepts. Whether you're a database professional looking to expand your knowledge or a complete beginner, this guide will help you understand why graph databases are becoming increasingly important in today's data-driven world. What Are Graph Databases? Graph databases represent a paradigm shift in how we store and query connected data. Unlike traditional relational databases that use tables and rows, graph databases use a structure that directly maps to how we naturally think about information—as interconnected entities. Core Components of a Graph Database: Nodes - Represent entities (such as people, businesses, accounts, or any other item you might want to track) Relationships - Connect nodes and represent how entities are related to each other Properties - Attributes that provide information about the nodes and relationships Labels - Used to group and categorize nodes Why Neo4j? Neo4j is the world's leading graph database platform, designed from the ground up to leverage not just the data but also the relationships between data. Here's why Neo4j stands out: Key Benefits: Native Graph Storage: Optimized for storing and querying connected data Flexible Schema: Adapt to changing business requirements without expensive migrations Cypher Query Language: An intuitive, declarative query language specifically designed for graphs ACID Compliance: Ensures data reliability and integrity High Performance: Efficient for traversing relationships, even at scale Rich Ecosystem: Drivers for popular programming languages, visualization tools, and more Understanding the Graph Data Model Let's explore the graph data model using a simple example that illustrates how real-world entities and their relationships can be represented in Neo4j. Example: Social Network Imagine we want to model a basic social network with people, their relationships, and interests: Nodes: People (Alice, Bob, Charlie) and Interests (Cycling, Reading, Movies) Relationships: FRIENDS_WITH (between people), INTERESTED_IN (connecting people to interests) Properties: For people: name, age; For interests: name, category Here's how this might look in Neo4j's visual representation: (Alice:Person {name: "Alice", age: 30})-[:FRIENDS_WITH]->(Bob:Person {name: "Bob", age: 32}) (Alice)-[:FRIENDS_WITH]->(Charlie:Person {name: "Charlie", age: 25}) (Bob)-[:FRIENDS_WITH]->(Charlie) (Alice)-[:INTERESTED_IN]->(Cycling:Interest {name: "Cycling", category: "Sports"}) (Bob)-[:INTERESTED_IN]->(Reading:Interest {name: "Reading", category: "Hobby"}) (Charlie)-[:INTERESTED_IN]->(Movies:Interest {name: "Movies", category: "Entertainment"}) (Charlie)-[:INTERESTED_IN]->(Cycling) Graph Databases vs. Relational Databases To understand the advantages of graph databases, let's compare them with traditional relational databases: Relational Approach: In a relational database, we might have: A people table with columns for id, name, age A interests table with columns for id, name, category A friendships table linking people_id to people_id A person_interests table linking people_id to interests_id To find "friends of friends who share my interests" would require: Multiple JOIN operations Complex SQL queries Potentially slow performance as the data grows Graph Approach: In Neo4j, the same query is intuitive and efficient: MATCH (me:Person {name: "Alice"})-[:FRIENDS_WITH]->(:Person)-[:FRIENDS_WITH]->(fof:Person), (fof)-[:INTERESTED_IN]->(interest:Interest)(b) Querying the Graph: // Find all people who are interested in Cycling MATCH (p:Person)-[:INTERESTED_IN]->(i:Interest {name: "Cycling"}) RETURN p.name, p.age Updating Properties: // Update Alice's age MATCH (p:Person {name: "Alice"}) SET p.age = 31 RETURN p Deleting Nodes and Relationships: // Delete a relationship MATCH (a:Person {name: "Alice"})-[r:FRIENDS_WITH]->(b:Person {name: "Bob"}) DELETE r // Delete a node and its relationships MATCH (p:Person {name: "David"}) DETACH DELETE p Real-World Use Cases Graph databases excel in many domains where relationships between entities are crucial: Recommendation Engines: Product recommendations based on purchase history and similar customers Content recommendations for media platforms Friend and connection suggestions in social networks Fraud Detection: Identifying suspicious patterns in financial transactions Detecting rings of fraudulent accounts Recognizing unusual behavior in real-time Network and IT Operations: Mapping infrastructure dependencies Impact analysis for changes and outages Root cause analy
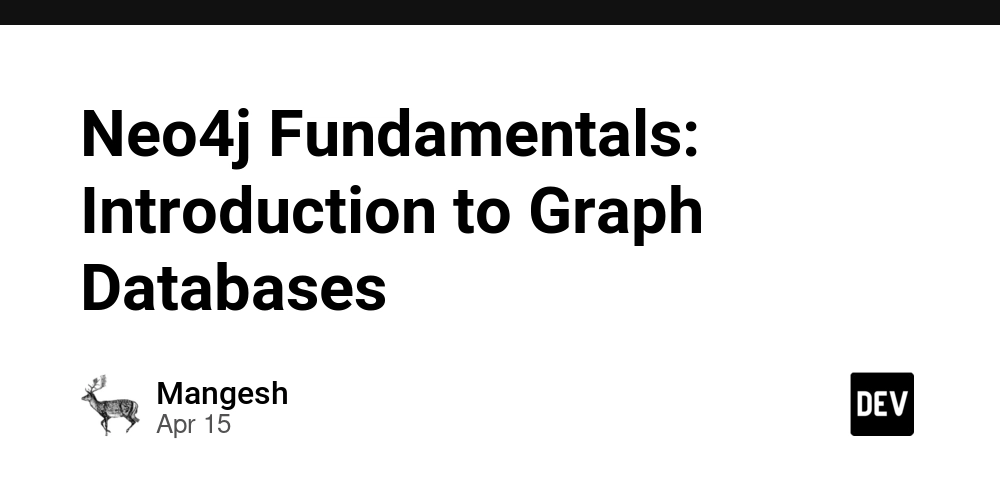
Introduction
Welcome to the world of graph databases! This tutorial is based on the first video in the "Neo4j Graph Database Tutorial" series and provides a comprehensive introduction to Neo4j and graph database concepts. Whether you're a database professional looking to expand your knowledge or a complete beginner, this guide will help you understand why graph databases are becoming increasingly important in today's data-driven world.
What Are Graph Databases?
Graph databases represent a paradigm shift in how we store and query connected data. Unlike traditional relational databases that use tables and rows, graph databases use a structure that directly maps to how we naturally think about information—as interconnected entities.
Core Components of a Graph Database:
- Nodes - Represent entities (such as people, businesses, accounts, or any other item you might want to track)
- Relationships - Connect nodes and represent how entities are related to each other
- Properties - Attributes that provide information about the nodes and relationships
- Labels - Used to group and categorize nodes
Why Neo4j?
Neo4j is the world's leading graph database platform, designed from the ground up to leverage not just the data but also the relationships between data. Here's why Neo4j stands out:
Key Benefits:
- Native Graph Storage: Optimized for storing and querying connected data
- Flexible Schema: Adapt to changing business requirements without expensive migrations
- Cypher Query Language: An intuitive, declarative query language specifically designed for graphs
- ACID Compliance: Ensures data reliability and integrity
- High Performance: Efficient for traversing relationships, even at scale
- Rich Ecosystem: Drivers for popular programming languages, visualization tools, and more
Understanding the Graph Data Model
Let's explore the graph data model using a simple example that illustrates how real-world entities and their relationships can be represented in Neo4j.
Example: Social Network
Imagine we want to model a basic social network with people, their relationships, and interests:
- Nodes: People (Alice, Bob, Charlie) and Interests (Cycling, Reading, Movies)
- Relationships: FRIENDS_WITH (between people), INTERESTED_IN (connecting people to interests)
- Properties: For people: name, age; For interests: name, category
Here's how this might look in Neo4j's visual representation:
(Alice:Person {name: "Alice", age: 30})-[:FRIENDS_WITH]->(Bob:Person {name: "Bob", age: 32})
(Alice)-[:FRIENDS_WITH]->(Charlie:Person {name: "Charlie", age: 25})
(Bob)-[:FRIENDS_WITH]->(Charlie)
(Alice)-[:INTERESTED_IN]->(Cycling:Interest {name: "Cycling", category: "Sports"})
(Bob)-[:INTERESTED_IN]->(Reading:Interest {name: "Reading", category: "Hobby"})
(Charlie)-[:INTERESTED_IN]->(Movies:Interest {name: "Movies", category: "Entertainment"})
(Charlie)-[:INTERESTED_IN]->(Cycling)
Graph Databases vs. Relational Databases
To understand the advantages of graph databases, let's compare them with traditional relational databases:
Relational Approach:
In a relational database, we might have:
- A
people
table with columns for id, name, age - A
interests
table with columns for id, name, category - A
friendships
table linking people_id to people_id - A
person_interests
table linking people_id to interests_id
To find "friends of friends who share my interests" would require:
- Multiple JOIN operations
- Complex SQL queries
- Potentially slow performance as the data grows
Graph Approach:
In Neo4j, the same query is intuitive and efficient:
MATCH (me:Person {name: "Alice"})-[:FRIENDS_WITH]->(:Person)-[:FRIENDS_WITH]->(fof:Person),
(fof)-[:INTERESTED_IN]->(interest:Interest)<-[:INTERESTED_IN]-(me)
RETURN fof.name, interest.name
This query directly follows the path through the graph, making it:
- More intuitive to write and understand
- Significantly faster to execute, especially as data grows
- More flexible when adding new types of relationships
Getting Started with Neo4j
Now that we understand the basics, let's look at how to get started with Neo4j:
Installation Options:
- Neo4j Desktop: A user-friendly application that includes everything you need to develop with Neo4j locally
- Neo4j Aura: A fully managed cloud service (includes a free tier)
- Neo4j Server: Self-hosted option for on-premises deployment
- Neo4j Sandbox: Free, temporary environments for learning and testing
First Steps with Neo4j Desktop:
- Download and install Neo4j Desktop from Neo4j's website
- Create a new project
- Add a database to your project
- Start the database
- Open Neo4j Browser (the built-in visualization and query tool)
Introduction to Cypher Query Language
Cypher is Neo4j's graph query language, designed to be both human-readable and efficient. Let's look at some basic Cypher queries:
Creating Nodes:
// Create a person node
CREATE (p:Person {name: "David", age: 42})
Creating Relationships:
// First find two nodes
MATCH (a:Person {name: "Alice"}), (b:Person {name: "Bob"})
// Then create a relationship between them
CREATE (a)-[:FRIENDS_WITH {since: 2020}]->(b)
Querying the Graph:
// Find all people who are interested in Cycling
MATCH (p:Person)-[:INTERESTED_IN]->(i:Interest {name: "Cycling"})
RETURN p.name, p.age
Updating Properties:
// Update Alice's age
MATCH (p:Person {name: "Alice"})
SET p.age = 31
RETURN p
Deleting Nodes and Relationships:
// Delete a relationship
MATCH (a:Person {name: "Alice"})-[r:FRIENDS_WITH]->(b:Person {name: "Bob"})
DELETE r
// Delete a node and its relationships
MATCH (p:Person {name: "David"})
DETACH DELETE p
Real-World Use Cases
Graph databases excel in many domains where relationships between entities are crucial:
Recommendation Engines:
- Product recommendations based on purchase history and similar customers
- Content recommendations for media platforms
- Friend and connection suggestions in social networks
Fraud Detection:
- Identifying suspicious patterns in financial transactions
- Detecting rings of fraudulent accounts
- Recognizing unusual behavior in real-time
Network and IT Operations:
- Mapping infrastructure dependencies
- Impact analysis for changes and outages
- Root cause analysis
Knowledge Graphs:
- Connecting disparate information for a unified view
- Powering intelligent search and discovery
- Building enterprise knowledge bases
Best Practices for Neo4j Beginners
As you start working with Neo4j, keep these best practices in mind:
- Model for queries: Design your graph to optimize for the questions you'll ask
- Use meaningful labels: Clear labels make queries more intuitive
- Be consistent with relationships: Standardize relationship types and directions
- Start small: Begin with a subset of your data to validate your model
- Learn the Cypher style: Use the visual pattern-matching nature of Cypher to your advantage
Conclusion
Graph databases like Neo4j offer a powerful way to work with connected data that aligns with how we naturally think about relationships. By representing both entities and their connections as first-class citizens, Neo4j enables faster queries, more flexible data models, and deeper insights into complex relationships.
This introduction has covered the fundamentals of graph databases and Neo4j, but there's much more to explore as you continue your journey.