Mastering software architecture: 6 patterns every developer should know and actually use
Skip the buzzwords Here’s what really works when building scalable, maintainable systems, explained with real world examples. Introduction Let’s be real: most of us didn’t wake up one morning thinking, “Today, I will become a software architect!” Usually, it starts with a small project turning into a monster, code screaming from every corner, and you realizing, “Maybe I should have structured this better.” Software architecture isn’t about sounding fancy in meetings. It’s about building systems that don’t collapse the minute your app hits 1000 users… or your boss says, “Hey, can we add this tiny feature?” In this article, I’ll break down six architecture patterns that aren’t just academic they actually work. Real-world examples, simple breakdowns, a few hard truths no buzzwords, just stuff you can use to survive (and maybe even enjoy) your next big project. 1. Monolithic Architecture If software architecture was a video game, monolithic would be the “starter pack” you can’t skip. It’s the simplest way to build something: one codebase, one deployment, and one server to (sometimes) rule them all. In a monolithic architecture, your UI, business logic, and database access are all bundled together like one massive burrito. It’s fast to start, easy to debug, and perfect for MVPs or small projects. When Monoliths Work Well: Early-stage startups (you need speed, not complexity). Internal tools (only 10 people will ever use it). Systems where speed of deployment beats scalability. Where Monoliths Can Hurt: Growing teams every update risks breaking unrelated parts. Scaling specific services you can’t just scale one feature. Deployments become “all or nothing” (aka deployment roulette). Real-world example: Instagram started as a monolith. Only after millions of users came flooding in did they begin splitting things into services. Lesson? Start simple, scale later. 2. Layered (N-Tier) Architecture Think of layered architecture like a lasagna. Each layer has its own job and you better not mix them unless you want a soggy disaster. At its core, layered architecture breaks your app into logical layers: Presentation Layer (UI, frontend) Business Logic Layer (rules, workflows) Data Access Layer (database communication) Sometimes you’ll hear it called N-Tier Architecture when each layer physically runs on different machines or servers. When Layered Architecture Works Well: Traditional web apps (think: ecommerce, banking portals). Teams that need clear boundaries (“frontend” vs “backend” teams). Systems where changes are isolated (you can swap UI without breaking the database). Where It Can Hurt: Too many layers = slower development. Tight coupling if you’re not careful, one broken service can cause domino collapses. Overengineering for simple apps. Real-world example: Classic Spring Boot Java apps or ASP.NET projects are often layered neatly to make maintenance a breeze. 3. Microservices Architecture Microservices is like breaking your giant game map into tiny, modular levels each with its own rules, enemies, and checkpoints. Instead of one giant codebase, you split your app into independent services. Each service does one thing well (in theory) and can be deployed, updated, and scaled separately. When Microservices Work Well: Big teams working on different parts of the app. High-scale apps needing independent scaling (like search vs payments). When you need flexibility to use different tech stacks for different services. Where It Can Hurt: Communication between services can become nightmare spaghetti. Debugging across 20+ microservices? Good luck. Deployment pipelines need serious automation manual deploys = instant chaos. Real-world example: Netflix is the poster child for microservices thousands of services handling everything from recommendations to billing. But guess what? It took them years to do it properly. 4. Event Driven Architecture Event Driven Architecture is like building a giant system out of trapdoors. Instead of making a direct call (“Hey, do this!”), you just shout an event into the void (“OrderPlaced!”) and whoever is listening can react however they want. An event is just a tiny fact: “Something happened.” Services subscribe to events and decide what to do next, keeping everything loosely coupled and super flexible. When Event-Driven Architecture Works Well: Systems needing massive scalability (like e-commerce checkouts, real time notifications). When services must react independently to actions (like billing after a subscription purchase). Handling high-volume data flows without clogging a single system. Where It Can Hurt: Debugging turns into detective work: “Wait… who triggered this again?!” Data consistency can get tricky fast. If events aren’t well documented, you’ll need a psychic to figure them out later. Real-world example: Amazon uses heavy event-driven architecture behind things like order processing and inventory management every “order placed” triggers multiple indepen
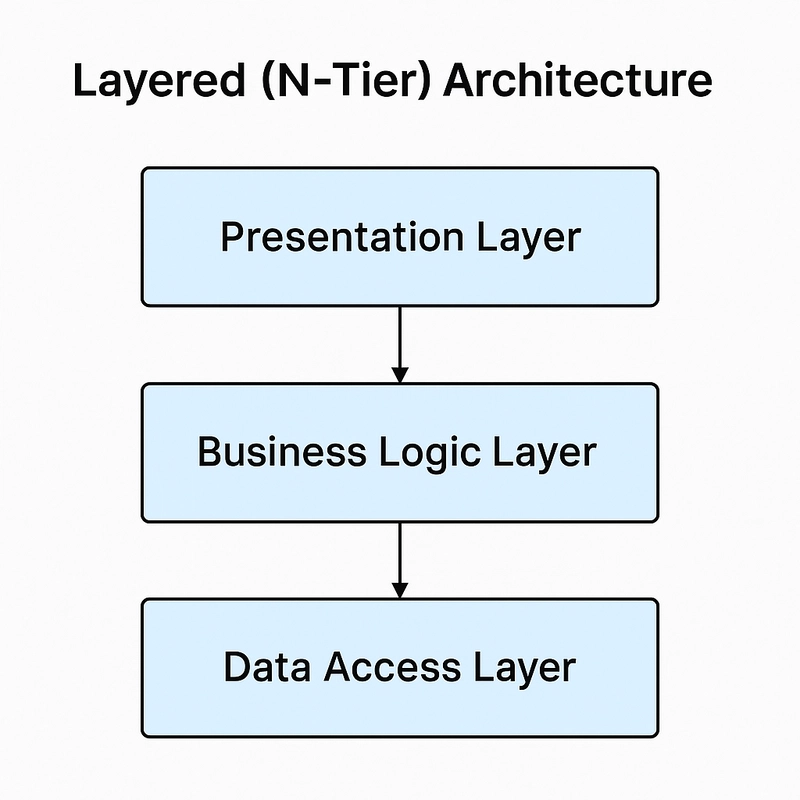
Skip the buzzwords Here’s what really works when building scalable, maintainable systems, explained with real world examples.
Introduction
Let’s be real: most of us didn’t wake up one morning thinking,
“Today, I will become a software architect!”
Usually, it starts with a small project turning into a monster, code screaming from every corner, and you realizing, “Maybe I should have structured this better.”
Software architecture isn’t about sounding fancy in meetings. It’s about building systems that don’t collapse the minute your app hits 1000 users… or your boss says, “Hey, can we add this tiny feature?”
In this article, I’ll break down six architecture patterns that aren’t just academic they actually work. Real-world examples, simple breakdowns, a few hard truths no buzzwords, just stuff you can use to survive (and maybe even enjoy) your next big project.
1. Monolithic Architecture
If software architecture was a video game, monolithic would be the “starter pack” you can’t skip.
It’s the simplest way to build something: one codebase, one deployment, and one server to (sometimes) rule them all.
In a monolithic architecture, your UI, business logic, and database access are all bundled together like one massive burrito. It’s fast to start, easy to debug, and perfect for MVPs or small projects.
When Monoliths Work Well:
- Early-stage startups (you need speed, not complexity).
- Internal tools (only 10 people will ever use it).
- Systems where speed of deployment beats scalability.
Where Monoliths Can Hurt:
- Growing teams every update risks breaking unrelated parts.
- Scaling specific services you can’t just scale one feature.
- Deployments become “all or nothing” (aka deployment roulette).
Real-world example:
Instagram started as a monolith. Only after millions of users came flooding in did they begin splitting things into services. Lesson? Start simple, scale later.
2. Layered (N-Tier) Architecture
Think of layered architecture like a lasagna.
Each layer has its own job and you better not mix them unless you want a soggy disaster.
At its core, layered architecture breaks your app into logical layers:
- Presentation Layer (UI, frontend)
- Business Logic Layer (rules, workflows)
- Data Access Layer (database communication)
Sometimes you’ll hear it called N-Tier Architecture when each layer physically runs on different machines or servers.
When Layered Architecture Works Well:
- Traditional web apps (think: ecommerce, banking portals).
- Teams that need clear boundaries (“frontend” vs “backend” teams).
- Systems where changes are isolated (you can swap UI without breaking the database).
Where It Can Hurt:
- Too many layers = slower development.
- Tight coupling if you’re not careful, one broken service can cause domino collapses.
- Overengineering for simple apps.
Real-world example:
Classic Spring Boot Java apps or ASP.NET projects are often layered neatly to make maintenance a breeze.
3. Microservices Architecture
Microservices is like breaking your giant game map into tiny, modular levels each with its own rules, enemies, and checkpoints.
Instead of one giant codebase, you split your app into independent services. Each service does one thing well (in theory) and can be deployed, updated, and scaled separately.
When Microservices Work Well:
- Big teams working on different parts of the app.
- High-scale apps needing independent scaling (like search vs payments).
- When you need flexibility to use different tech stacks for different services.
Where It Can Hurt:
- Communication between services can become nightmare spaghetti.
- Debugging across 20+ microservices? Good luck.
- Deployment pipelines need serious automation manual deploys = instant chaos.
Real-world example:
Netflix is the poster child for microservices thousands of services handling everything from recommendations to billing. But guess what? It took them years to do it properly.
4. Event Driven Architecture
Event Driven Architecture is like building a giant system out of trapdoors.
Instead of making a direct call (“Hey, do this!”), you just shout an event into the void (“OrderPlaced!”) and whoever is listening can react however they want.
An event is just a tiny fact: “Something happened.”
Services subscribe to events and decide what to do next, keeping everything loosely coupled and super flexible.
When Event-Driven Architecture Works Well:
- Systems needing massive scalability (like e-commerce checkouts, real time notifications).
- When services must react independently to actions (like billing after a subscription purchase).
- Handling high-volume data flows without clogging a single system.
Where It Can Hurt:
- Debugging turns into detective work: “Wait… who triggered this again?!”
- Data consistency can get tricky fast.
- If events aren’t well documented, you’ll need a psychic to figure them out later.
Real-world example:
Amazon uses heavy event-driven architecture behind things like order processing and inventory management every “order placed” triggers multiple independent downstream services.
5. Serverless Architecture
Serverless sounds like magic no servers, just pure code running in the cloud!
Reality check: there are still servers… you just don’t manage them anymore.
In serverless architecture, you write small functions that cloud providers (like AWS Lambda, Azure Functions) run on demand. You pay only for execution time, not idle server hours.
When Serverless Works Well:
- Apps with unpredictable traffic (huge spikes, long lulls).
- Lightweight APIs, background jobs, automation scripts.
- Startups needing MVPs without massive infrastructure costs.
Where It Can Hurt:
- Cold starts = waiting awkwardly while your function wakes up.
- Complex applications become hard to coordinate if you split into too many tiny functions.
- Vendor lock-in: moving away from AWS/GCP/Azure later can be painful.
Real-world example:
Netflix uses serverless for real-time encoding, user notification triggers, and data processing pipelines especially when workloads spike unpredictably.
Quick Reminder:
Serverless doesn’t mean “no ops.” It just means “someone else’s ops.” (And they still fail sometimes.)
6. Hexagonal (Ports and Adapters) Architecture
Hexagonal Architecture sounds complicated but it’s secretly one of the most developer-friendly patterns ever invented.
Nicknamed Ports and Adapters, it’s about protecting your core business logic from messy outside worlds (like databases, APIs, UI frameworks).
Imagine your app is a castle