Mastering `ignore_changes` with `count`, `for_each`, and `lifecycle` in Terraform for Scalable Infrastructure
Terraform is a cornerstone of Infrastructure as Code (IaC), enabling engineers to define and manage cloud infrastructure with precision and scalability. However, mastering Terraform requires a deep understanding of its core constructs, such as count, for_each, and lifecycle. One particularly tricky challenge is dynamically managing the ignore_changes directive within the lifecycle block. This blog dives into how to tackle this challenge by combining these features effectively. We’ll cover: How count, for_each, and lifecycle work in Terraform The challenge of dynamically setting ignore_changes How conditional resource creation solves this problem When to use count vs. for_each in dynamic scenarios Best practices for maintainable and scalable Terraform configurations Understanding count, for_each, and lifecycle in Terraform count: Creating Multiple Resource Instances The count parameter allows you to create multiple instances of a resource by specifying a numeric value. It’s ideal for scenarios where you need identical resources with minor variations, such as multiple EC2 instances. resource "aws_instance" "example" { count = 3 ami = "ami-123456" instance_type = "t2.micro" } This creates three identical EC2 instances. You can reference each instance using aws_instance.example[0], aws_instance.example[1], etc. for_each: Managing Unique Resource Configurations The for_each parameter is used to create resources based on a map or set. Each resource instance can have unique configurations, making it ideal for managing distinct environments or configurations. resource "aws_instance" "example" { for_each = toset(["dev", "prod", "test"]) ami = "ami-123456" instance_type = "t2.micro" tags = { Name = each.key } } Here, three EC2 instances are created, each tagged with a unique name (dev, prod, test). lifecycle: Controlling Resource Behavior The lifecycle block allows you to define how Terraform should handle resource updates. One of its most useful features is ignore_changes, which prevents Terraform from modifying specific attributes after creation. resource "aws_instance" "example" { ami = "ami-123456" instance_type = "t2.micro" lifecycle { ignore_changes = [tags] } } In this example, Terraform will ignore changes to the tags attribute, ensuring that manual updates to tags don’t trigger unnecessary resource updates. The Challenge: Dynamically Setting ignore_changes While ignore_changes is powerful, it lacks native support for dynamic configurations. For example, you might want to enable or disable ignore_changes based on a condition, such as the environment or a feature flag. Unfortunately, Terraform doesn’t allow dynamic expressions directly inside the lifecycle block. Why This Matters? Imagine a scenario where you want to ignore changes to the tags attribute in production but not in development. Without dynamic ignore_changes, you’d need to duplicate resources or manually manage configurations, leading to bloated and hard-to-maintain code. The Solution: Conditional Resource Creation To work around this limitation, you can use conditional resource creation with count or for_each. The idea is to create two versions of the same resource—one with ignore_changes and one without—and conditionally create only the appropriate version. Example: Conditional ignore_changes variable "use_ignore_changes" { type = bool default = true } resource "aws_instance" "with_ignore_changes" { count = var.use_ignore_changes ? 1 : 0 ami = "ami-123456" instance_type = "t2.micro" lifecycle { ignore_changes = [tags] } } resource "aws_instance" "without_ignore_changes" { count = var.use_ignore_changes ? 0 : 1 ami = "ami-123456" instance_type = "t2.micro" } In this example: If var.use_ignore_changes is true, the with_ignore_changes resource is created. If var.use_ignore_changes is false, the without_ignore_changes resource is created. This approach ensures that only the desired resource configuration is applied, avoiding duplication and maintaining clean code. count vs. for_each: Choosing the Right Tool Both count and for_each are powerful, but they serve different purposes. Choosing the right one depends on your use case. When to Use count Use Case: Creating multiple identical resources or enabling/disabling resources based on a condition. Example: Enabling ignore_changes for a specific environment. Pros: Simple and straightforward for boolean logic. Cons: Limited flexibility for unique configurations. When to Use for_each Use Case: Managing resources with unique configurations, such as different environments or settings. Example: Creating EC2 instances with distinct tags for dev, prod, and test. Pros: Highly flexible for dynamic and
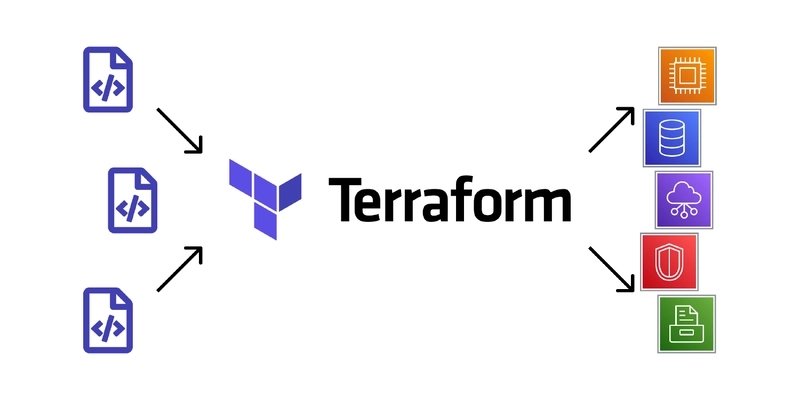
Terraform is a cornerstone of Infrastructure as Code (IaC), enabling engineers to define and manage cloud infrastructure with precision and scalability. However, mastering Terraform requires a deep understanding of its core constructs, such as count
, for_each
, and lifecycle
. One particularly tricky challenge is dynamically managing the ignore_changes
directive within the lifecycle
block. This blog dives into how to tackle this challenge by combining these features effectively.
We’ll cover:
- How
count
,for_each
, andlifecycle
work in Terraform - The challenge of dynamically setting
ignore_changes
- How conditional resource creation solves this problem
- When to use
count
vs.for_each
in dynamic scenarios - Best practices for maintainable and scalable Terraform configurations
Understanding count
, for_each
, and lifecycle
in Terraform
count
: Creating Multiple Resource Instances
The count
parameter allows you to create multiple instances of a resource by specifying a numeric value. It’s ideal for scenarios where you need identical resources with minor variations, such as multiple EC2 instances.
resource "aws_instance" "example" {
count = 3
ami = "ami-123456"
instance_type = "t2.micro"
}
This creates three identical EC2 instances. You can reference each instance using aws_instance.example[0]
, aws_instance.example[1]
, etc.
for_each
: Managing Unique Resource Configurations
The for_each
parameter is used to create resources based on a map or set. Each resource instance can have unique configurations, making it ideal for managing distinct environments or configurations.
resource "aws_instance" "example" {
for_each = toset(["dev", "prod", "test"])
ami = "ami-123456"
instance_type = "t2.micro"
tags = {
Name = each.key
}
}
Here, three EC2 instances are created, each tagged with a unique name (dev
, prod
, test
).
lifecycle
: Controlling Resource Behavior
The lifecycle
block allows you to define how Terraform should handle resource updates. One of its most useful features is ignore_changes
, which prevents Terraform from modifying specific attributes after creation.
resource "aws_instance" "example" {
ami = "ami-123456"
instance_type = "t2.micro"
lifecycle {
ignore_changes = [tags]
}
}
In this example, Terraform will ignore changes to the tags
attribute, ensuring that manual updates to tags don’t trigger unnecessary resource updates.
The Challenge: Dynamically Setting ignore_changes
While ignore_changes
is powerful, it lacks native support for dynamic configurations. For example, you might want to enable or disable ignore_changes
based on a condition, such as the environment or a feature flag. Unfortunately, Terraform doesn’t allow dynamic expressions directly inside the lifecycle
block.
Why This Matters?
Imagine a scenario where you want to ignore changes to the tags
attribute in production but not in development. Without dynamic ignore_changes
, you’d need to duplicate resources or manually manage configurations, leading to bloated and hard-to-maintain code.
The Solution: Conditional Resource Creation
To work around this limitation, you can use conditional resource creation with count
or for_each
. The idea is to create two versions of the same resource—one with ignore_changes
and one without—and conditionally create only the appropriate version.
Example: Conditional ignore_changes
variable "use_ignore_changes" {
type = bool
default = true
}
resource "aws_instance" "with_ignore_changes" {
count = var.use_ignore_changes ? 1 : 0
ami = "ami-123456"
instance_type = "t2.micro"
lifecycle {
ignore_changes = [tags]
}
}
resource "aws_instance" "without_ignore_changes" {
count = var.use_ignore_changes ? 0 : 1
ami = "ami-123456"
instance_type = "t2.micro"
}
In this example:
- If
var.use_ignore_changes
istrue
, thewith_ignore_changes
resource is created. - If
var.use_ignore_changes
isfalse
, thewithout_ignore_changes
resource is created.
This approach ensures that only the desired resource configuration is applied, avoiding duplication and maintaining clean code.
count
vs. for_each
: Choosing the Right Tool
Both count
and for_each
are powerful, but they serve different purposes. Choosing the right one depends on your use case.
When to Use count
- Use Case: Creating multiple identical resources or enabling/disabling resources based on a condition.
-
Example: Enabling
ignore_changes
for a specific environment. - Pros: Simple and straightforward for boolean logic.
- Cons: Limited flexibility for unique configurations.
When to Use for_each
- Use Case: Managing resources with unique configurations, such as different environments or settings.
-
Example: Creating EC2 instances with distinct tags for
dev
,prod
, andtest
. - Pros: Highly flexible for dynamic and unique configurations.
-
Cons: Slightly more complex to set up compared to
count
.
Best Practices for Dynamic ignore_changes
-
Use Variables for Flexibility: Define variables like
use_ignore_changes
to make your configuration reusable across environments. - Leverage Modules: Encapsulate conditional logic in modules to keep your root configuration clean and maintainable.
-
Document Your Logic: Clearly document why and how
ignore_changes
is being used to avoid confusion for future maintainers. - Test Thoroughly: Use Terraform’s plan and apply commands to validate that the correct resources are being created.
Summary
Dynamically managing ignore_changes
in Terraform requires creativity and a solid understanding of count
, for_each
, and lifecycle
. By using conditional resource creation, you can achieve dynamic behavior while keeping your code clean and maintainable. Whether you choose count
or for_each
depends on your specific use case, but both approaches offer powerful ways to enhance your Terraform configurations.