Mastering CSS Grid: Build Responsive Layouts with Ease
Mastering CSS Grid: Build Responsive Layouts with Ease CSS Grid Layout has revolutionized the way we design web interfaces. With its powerful capabilities, it allows developers to create complex responsive layouts without the need for excessive CSS or JavaScript. In this article, we will explore the key features of CSS Grid, how to implement it in your projects, and some best practices to follow. What is CSS Grid? CSS Grid Layout is a two-dimensional layout system for the web. It enables you to control both rows and columns, offering much more flexibility compared to older layout methods like float or inline-block. With Grid, you can design layouts that adapt beautifully to different screen sizes and orientations. Getting Started with CSS Grid To start using CSS Grid, you need to define a grid container by applying the display: grid; property to an element. Here’s a simple example: .grid-container { display: grid; grid-template-columns: repeat(3, 1fr); gap: 10px; } In this example, we are creating a grid container with three equal columns and a gap of 10 pixels between the grid items. Grid Items Once the container is set up, you can add grid items. Here’s how you can structure your HTML: 1 2 3 4 5 6 Responsive Design with CSS Grid One of the major advantages of CSS Grid is its capability to make responsive design seamless. By using media queries, we can adjust our grid layout for different screen sizes. Here’s a modified example: @media (max-width: 600px) { .grid-container { grid-template-columns: 1fr; } } This code sets the grid to a single column layout for screens that are 600 pixels or narrower. Key CSS Grid Properties To effectively use CSS Grid, it's essential to understand its key properties: grid-template-rows and grid-template-columns: Define the structure of rows and columns. grid-area: Allows assigning a specific area of the grid to an item. grid-row and grid-column: Control the placement of grid items. gap: Defines the space between grid items (these can also be defined with row-gap and column-gap). Example: Defining Grid Areas Here is an example of how you can define areas within your grid, making layout design more intuitive: .grid-container { display: grid; grid-template-areas: 'header header header' 'sidebar content content' 'footer footer footer'; } .header { grid-area: header; } .sidebar { grid-area: sidebar; } .content { grid-area: content; } .footer { grid-area: footer; } This defines four areas in the grid: header, sidebar, content, and footer, allowing for a more semantic structure in your HTML. Best Practices 1. Start Simple When first implementing CSS Grid, begin with basic layouts. Gradually add complexity as you become more comfortable. 2. Use Named Grid Areas Using named grid areas can make your CSS clearer and more maintainable. It helps anyone reading the code understand which section of the layout is being referenced. 3. Keep Accessibility in Mind While designing layouts, ensure you keep accessibility in mind. Utilize semantic HTML and ARIA roles where necessary to improve screen reader experiences. 4. Test Across Devices Always test your layouts on multiple devices and screen sizes to ensure they respond correctly under different conditions. Conclusion CSS Grid is a powerful layout system that can significantly enhance your frontend development process. By taking advantage of its features, you can create dynamic and responsive designs with minimal effort. As you practice these techniques and explore its capabilities, you’ll find that CSS Grid is an indispensable tool in your CSS toolbox. Embrace the grid today and elevate your web designs to new heights! Further Reading CSS Tricks: A Complete Guide to Grid MDN Web Docs on CSS Grid A Book Apart - CSS Grid Layout
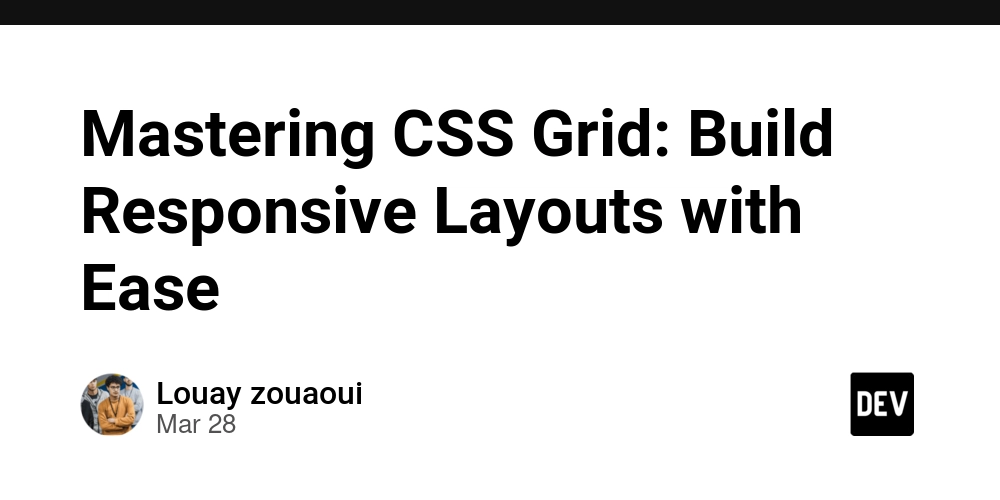
Mastering CSS Grid: Build Responsive Layouts with Ease
CSS Grid Layout has revolutionized the way we design web interfaces. With its powerful capabilities, it allows developers to create complex responsive layouts without the need for excessive CSS or JavaScript. In this article, we will explore the key features of CSS Grid, how to implement it in your projects, and some best practices to follow.
What is CSS Grid?
CSS Grid Layout is a two-dimensional layout system for the web. It enables you to control both rows and columns, offering much more flexibility compared to older layout methods like float or inline-block. With Grid, you can design layouts that adapt beautifully to different screen sizes and orientations.
Getting Started with CSS Grid
To start using CSS Grid, you need to define a grid container by applying the display: grid;
property to an element. Here’s a simple example:
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 10px;
}
In this example, we are creating a grid container with three equal columns and a gap of 10 pixels between the grid items.
Grid Items
Once the container is set up, you can add grid items. Here’s how you can structure your HTML:
class="grid-container">
class="grid-item">1
class="grid-item">2
class="grid-item">3
class="grid-item">4
class="grid-item">5
class="grid-item">6
Responsive Design with CSS Grid
One of the major advantages of CSS Grid is its capability to make responsive design seamless. By using media queries, we can adjust our grid layout for different screen sizes. Here’s a modified example:
@media (max-width: 600px) {
.grid-container {
grid-template-columns: 1fr;
}
}
This code sets the grid to a single column layout for screens that are 600 pixels or narrower.
Key CSS Grid Properties
To effectively use CSS Grid, it's essential to understand its key properties:
-
grid-template-rows
andgrid-template-columns
: Define the structure of rows and columns. -
grid-area
: Allows assigning a specific area of the grid to an item. -
grid-row
andgrid-column
: Control the placement of grid items. -
gap
: Defines the space between grid items (these can also be defined withrow-gap
andcolumn-gap
).
Example: Defining Grid Areas
Here is an example of how you can define areas within your grid, making layout design more intuitive:
.grid-container {
display: grid;
grid-template-areas:
'header header header'
'sidebar content content'
'footer footer footer';
}
.header { grid-area: header; }
.sidebar { grid-area: sidebar; }
.content { grid-area: content; }
.footer { grid-area: footer; }
This defines four areas in the grid: header
, sidebar
, content
, and footer
, allowing for a more semantic structure in your HTML.
Best Practices
1. Start Simple
When first implementing CSS Grid, begin with basic layouts. Gradually add complexity as you become more comfortable.
2. Use Named Grid Areas
Using named grid areas can make your CSS clearer and more maintainable. It helps anyone reading the code understand which section of the layout is being referenced.
3. Keep Accessibility in Mind
While designing layouts, ensure you keep accessibility in mind. Utilize semantic HTML and ARIA roles where necessary to improve screen reader experiences.
4. Test Across Devices
Always test your layouts on multiple devices and screen sizes to ensure they respond correctly under different conditions.
Conclusion
CSS Grid is a powerful layout system that can significantly enhance your frontend development process. By taking advantage of its features, you can create dynamic and responsive designs with minimal effort. As you practice these techniques and explore its capabilities, you’ll find that CSS Grid is an indispensable tool in your CSS toolbox.
Embrace the grid today and elevate your web designs to new heights!