Core Content: VS2022 Getting Started | main Function | printf Basics | String '\0' Pitfalls I.What is C Language C is a programming language that bridges humans and computers, allowing us to communicate instructions to machines. Like natural languages such as Chinese or English, it’s part of a larger family of computer languages including C++, Java, and Python. Its design prioritizes efficiency and low-level system access, making it a cornerstone for system programming and embedded systems. II.The History and Glory of C Language Origins (1969–1972): Born from the need to port the Unix OS, C evolved from the B language developed by Ken Thompson and Dennis Ritchie at Bell Labs. Standardization (1988): ANSI formalized C as a standardized language, ensuring consistency across different systems. Modern Relevance: Still widely used in OS development, embedded systems, and performance-critical applications, ranking among the top languages in the TIOBE index.TIOBE III.Choosing a Compiler: VS2022 for Beginners Compilation and Linking C is a compiled language. Source code is a text file and cannot be executed directly. It needs to be translated by a compiler. The .c file is converted into an .obj object file, and then linked by a linker to generate a binary executable file (.exe) that can be run. Compilation Process Source Code (.c) → Compiler (e.g., MSVC) → Object File (.obj) → Linker → Executable (.exe). Example workflow in VS2022: Write code in .c files, compile with Ctrl+F7, run with Ctrl+F5. 2.Compiler Options Comparison Recommendation: Start with VS2022 Community Edition (free, widely used in industry).And you can refer to the installation tutorial at:Bilibili:Big Guy Hang 3.Advantages and Disadvantages of VS2022 The advantages are its powerful functions, including an editor, compiler, and debugger, and it is easy to get started. The disadvantage is that the installation package is large and takes up a lot of space. IV.VS Project Structure: Source Files & Header Files Source Files (.c): Contain actual C code (e.g.,main.c, test.c, utils.c ) //Example: main.c #include int main() { printf("Hello C!\n"); return 0; } Header Files (.h): Declare functions, macros, or data structures (e.g., stdio.h for printf Use #include to import headers: #include (system headers) or #include "my_header.h" (custom headers). V. Your First C Program: The Classic main Function Entry Point: Every C program starts with the main function, which must return an int (conventionally 0 for success). int main() { // Your code here return 0; // Mandatory return statement } Common Pitfalls for Beginners: 1.Spelling Mistake: main ≠ mian (a common typo!). 2.Missing Parentheses: Always use main() (the () are mandatory). 3.Chinese Symbols: Use only English () {} ; — Chinese characters will cause errors! 4.Forgotten Semicolons: Every statement must end with ; (e.g., printf("Hi!\n");). VI.printf and Library Functions: Your First Toolkit Printing to Screen: Use printf from stdio.h to display text or data. printf("Hello, World!\n"); // Simple string int num = 42; printf("The answer is %d\n", num); // Integer placeholder (%d) Library Functions: Pre-written code for common tasks (e.g., scanf for input, strlen for string length). Always include the corresponding header (e.g., #include for I/O functions). VII.Keywords: The Building Blocks of Syntax Reserved Words: 32 core keywords in C (e.g., int, if, return), plus C99 additions like _Bool, inline. Rule: Never use keywords as variable names! int if = 10; // ERROR: "if" is a keyword!! VIII.Characters & ASCII: How Computers Store Text Character Literals: Enclosed in single quotes: 'A', '5', '?'. ASCII Encoding: Maps characters to 7-bit numbers (0–127). Key ranges: 1.Letters: 'A'=65, 'Z'=90; 'a'=97, 'z'=122 2.Digits: '0'=48, '9'=57 3.Special: '\n'=10 (newline), '\0'=0 (null terminator) Code Example: Print a character using its ASCII value: printf("%c\n", 65); // Output: 'A' Key ASCII Ranges (Good to Remember!): 1.Uppercase Letters: 'A'=65 to 'Z'=90 2.Lowercase Letters: 'a'=97 to 'z'=122 (32 more than uppercase!) 3.Digits: '0'=48 to '9'=57 4.Newline: '\n'=10 (used to break lines in printf) 5.Printable vs. Non-Printable: 6.Printable: Characters with ASCII values 32-127 (e.g., letters, numbers, symbols like @#$). 7.Non-Printable: Values 0-31 (e.g., \n, \t, or invisible control characters). IX.Strings & \0: The Hidden Terminator String Literals: Enclosed in double quotes: "Hello", which secretly ends with \0. char good_msg[] = "Hi!"; // Actually stores 'H', 'i', '!', '\0' Always use string literals for safe string handling! Key Example (See the Difference!): char arr1[] = {'a', 'b', 'c'}; // No \0 → undefined behav
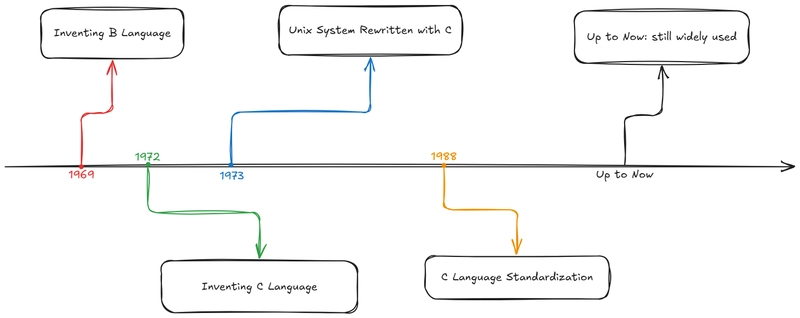
Core Content: VS2022 Getting Started | main Function | printf Basics | String '\0' Pitfalls
I.What is C Language
C is a programming language that bridges humans and computers, allowing us to communicate instructions to machines. Like natural languages such as Chinese or English, it’s part of a larger family of computer languages including C++, Java, and Python. Its design prioritizes efficiency and low-level system access, making it a cornerstone for system programming and embedded systems.
II.The History and Glory of C Language
- Origins (1969–1972): Born from the need to port the Unix OS, C evolved from the B language developed by Ken Thompson and Dennis Ritchie at Bell Labs.
- Standardization (1988): ANSI formalized C as a standardized language, ensuring consistency across different systems.
- Modern Relevance: Still widely used in OS development, embedded systems, and performance-critical applications, ranking among the top languages in the TIOBE index.TIOBE
III.Choosing a Compiler: VS2022 for Beginners
- Compilation and Linking C is a compiled language. Source code is a text file and cannot be executed directly. It needs to be translated by a compiler. The .c file is converted into an .obj object file, and then linked by a linker to generate a binary executable file (.exe) that can be run.
Compilation Process
- Source Code (.c) → Compiler (e.g., MSVC) → Object File (.obj) → Linker → Executable (.exe).
- Example workflow in VS2022: Write code in
.c
files, compile withCtrl+F7
, run withCtrl+F5
.
2.Compiler Options Comparison
Recommendation: Start with VS2022 Community Edition (free, widely used in industry).And you can refer to the installation tutorial at:Bilibili:Big Guy Hang
3.Advantages and Disadvantages of VS2022
The advantages are its powerful functions, including an editor, compiler, and debugger, and it is easy to get started. The disadvantage is that the installation package is large and takes up a lot of space.
IV.VS Project Structure: Source Files & Header Files
- Source Files (.c): Contain actual C code (e.g.,
main.c
,test.c
,utils.c
)
//Example: main.c
#include
int main()
{
printf("Hello C!\n");
return 0;
}
- Header Files (.h): Declare functions, macros, or data structures (e.g.,
stdio.h
forprintf
Use
#include
to import headers:#include
(system headers) or#include "my_header.h"
(custom headers).
V. Your First C Program: The Classic main
Function
-
Entry Point: Every C program starts with the main function, which must return an int (conventionally
0
for success).
int main()
{
// Your code here
return 0; // Mandatory return statement
}
-
Common Pitfalls for Beginners:
1.Spelling Mistake:
main
≠mian
(a common typo!).
2.Missing Parentheses: Always use
main()
(the()
are mandatory).
3.Chinese Symbols: Use only English
() {} ;
— Chinese characters will cause errors!
4.Forgotten Semicolons: Every statement must end with
;
(e.g.,printf("Hi!\n");
).
VI.printf
and Library Functions: Your First Toolkit
-
Printing to Screen: Use
printf
fromstdio.h
to display text or data.
printf("Hello, World!\n"); // Simple string
int num = 42;
printf("The answer is %d\n", num); // Integer placeholder (%d)
-
Library Functions: Pre-written code for common tasks (e.g.,
scanf
for input,strlen
for string length). Always include the corresponding header (e.g., #include for I/O functions).
VII.Keywords: The Building Blocks of Syntax
- Reserved Words: 32 core keywords in C (e.g., int, if, return), plus C99 additions like _Bool, inline.
- Rule: Never use keywords as variable names!
int if = 10; // ERROR: "if" is a keyword!!
VIII.Characters & ASCII: How Computers Store Text
-
Character Literals: Enclosed in single quotes:
'A'
,'5'
,'?'
. -
ASCII Encoding: Maps characters to 7-bit numbers (0–127). Key ranges:
1.Letters:
'A'=65
,'Z'=90
;'a'=97
,'z'=122
2.Digits:
'0'=48
,'9'=57
3.Special:
'\n'=10
(newline),'\0'=0
(null terminator) - Code Example: Print a character using its ASCII value:
printf("%c\n", 65); // Output: 'A'
-
Key ASCII Ranges (Good to Remember!):
1.Uppercase Letters:
'A'=65
to'Z'=90
2.Lowercase Letters:
'a'=97
to'z'=122
(32 more than uppercase!)
3.Digits:
'0'=48
to'9'=57
4.Newline:
'\n'=10
(used to break lines in printf)
5.Printable vs. Non-Printable:
6.Printable: Characters with ASCII values 32-127 (e.g., letters, numbers, symbols like
@#$
).
7.Non-Printable: Values 0-31 (e.g.,
\n
,\t
, or invisible control characters).
IX.Strings & \0
: The Hidden Terminator
-
String Literals: Enclosed in double quotes:
"Hello"
, which secretly ends with\0
.
char good_msg[] = "Hi!"; // Actually stores 'H', 'i', '!', '\0'
Always use string literals for safe string handling!
Key Example (See the Difference!):
char arr1[] = {'a', 'b', 'c'}; // No \0 → undefined behavior (random garbage after "abc")
char arr2[] = "abc"; // Equivalent to {'a','b','c','\0'} → safe and correct
printf("%s\n", arr1); // Output: "abc" followed by random characters (e.g., "abc烫烫烫")
printf("%s\n", arr2); // Output: "abc" (stops at \0)
Why \0
Matters: Without \0
, functions like printf
or strlen
can’t tell where the string ends, leading to unpredictable results (like printing garbage). Always use string literals (e.g., "hello"
) to let C add \0
automatically!
X.Escape Characters: Special Symbols with a Twist
-
What They Do: Change the meaning of a character (e.g.,
\n
= newline,\t
= tab). - Common Escapes:
XI.Statements: The Building Blocks of Code
C code is made of 5 types of statements:
-
Empty Statement: Just a semicolon
;
(useful in loops where no action is needed).
for (int i = 0; i < 10; i++); // Empty statement as the loop body
2.Expression Statement: An expression + ; (e.g., assignments or calculations).
int x = 20; // Assignment statement
x = x * 2; // Arithmetic statement
3.Function Call Statement: A function + ; (e.g., printf or your own functions).
printf("Goodbye!\n"); // Function call statement
4.Compound Statement: Code inside {}, also called a code block.
if (x > 0)
{
printf("Positive!\n"); // Block for the if statement
}
5.Control Statement: Controls flow (covered later!):
- Branches: if, switch
- Loops: for, while, do-while
- Jumps: break, continue, return
XII. Comments: Keep Your Code Human-Friendly
Explain code for others (and future you)! Two types:
1.Block Comments(/* ... */): For multi-line notes (no nesting!).
/*
This function adds two numbers
Returns x + y
*/
int add(int x, int y)
{
return x + y;
}
2.Line Comments (//): For single-line notes (C99 feature).
int age = 18; // User's age (must be positive!)
Important: Comments inside quotes are just text, not comments!
printf("// This is not a comment!"); // Output: // This is not a comment!
Next Lecture Preview
We’ll dive into data types (integers, floats, variables) and operators (+, -, *, etc.). Stay tuned for more level-up lessons!