Java Collections Framework – Part 1: ArrayList, LinkedList, Queue, Stack, and Deque
Java’s Collections Framework provides powerful data structures to manage and manipulate data efficiently. In this Part 1, we’ll cover ArrayList, LinkedList, Queue, Stack, and Deque, exploring all their methods with explanations and code snippets. 1. ArrayList in Java ArrayList is a dynamic array implementation that allows resizing and fast random access. Declaration and Initialization import java.util.ArrayList; import java.util.List; List list = new ArrayList(); Methods of ArrayList Method Description add(E e) Adds an element to the end. add(int index, E element) Inserts an element at the given index. get(int index) Retrieves the element at the given index. set(int index, E element) Updates the element at the specified index. remove(int index) Removes an element at the specified index. remove(Object o) Removes the first occurrence of an element. clear() Removes all elements. contains(Object o) Checks if an element is in the list. size() Returns the number of elements. indexOf(Object o) Returns the first occurrence index. lastIndexOf(Object o) Returns the last occurrence index. isEmpty() Checks if the list is empty. toArray() Converts the list to an array. subList(int fromIndex, int toIndex) Returns a portion of the list. sort(Comparator c) Retains only elements in the given collection. removeAll(Collection c) Removes elements present in another collection. Example: import java.util.ArrayList; import java.util.Collections; public class ArrayListExample { public static void main(String[] args) { ArrayList numbers = new ArrayList(); numbers.add(10); numbers.add(20); numbers.add(30); numbers.add(1, 15); // Insert at index 1 System.out.println(numbers.get(2)); // 20 numbers.remove(0); // Remove first element Collections.sort(numbers); // Sort the list System.out.println(numbers); } } 2. LinkedList in Java LinkedList is a doubly linked list implementation that provides fast insertions and deletions. Declaration and Initialization import java.util.LinkedList; import java.util.List; List linkedList = new LinkedList(); Methods of LinkedList Method Description add(E e) Adds an element at the end. add(int index, E element) Inserts an element at a given index. get(int index) Retrieves an element at an index. set(int index, E element) Replaces an element. remove(int index) Removes an element at an index. remove(Object o) Removes the first occurrence of an element. size() Returns the number of elements. clear() Clears all elements. contains(Object o) Checks if an element exists. isEmpty() Checks if the list is empty. toArray() Converts the list to an array. Deque-Specific Methods (Available in LinkedList) Method Description addFirst(E e) Adds an element at the front. addLast(E e) Adds an element at the end. removeFirst() Removes the first element. removeLast() Removes the last element. getFirst() Retrieves the first element without removing. getLast() Retrieves the last element without removing. Example: import java.util.LinkedList; public class LinkedListExample { public static void main(String[] args) { LinkedList names = new LinkedList(); names.add("Alice"); names.add("Bob"); names.addFirst("John"); names.addLast("Emma"); System.out.println(names.getFirst()); // John System.out.println(names.getLast()); // Emma names.removeFirst(); names.removeLast(); System.out.println(names); // [Alice, Bob] } } 3. Queue in Java A Queue follows FIFO (First In, First Out) order. Declaration and Initialization import java.util.Queue; import java.util.LinkedList; Queue queue = new LinkedList(); Methods of Queue Method Description offer(E e) Adds an element to the queue. poll() Retrieves and removes the head of the queue. peek() Retrieves the head but does not remove it. remove() Removes the head element. element() Retrieves but throws an exception if empty. Example: import java.util.LinkedList; import java.util.Queue; public class QueueExample { public static void main(String[] args) { Queue queue = new LinkedList(); queue.offer("Alice"); queue.offer("Bob"); queue.offer("Charlie"); System.out.println(queue.peek()); // Alice queue.poll(); System.out.println(queue); // [Bob, Charlie] } } 4. Stack in Java A Stack follows LIFO (Last In, First Out) order. Declaration and Initialization import java.util.Stack; Stack stack = new Stack(); Methods of Stack Method Description push(E item) Pushes an element onto the stack. pop() Removes
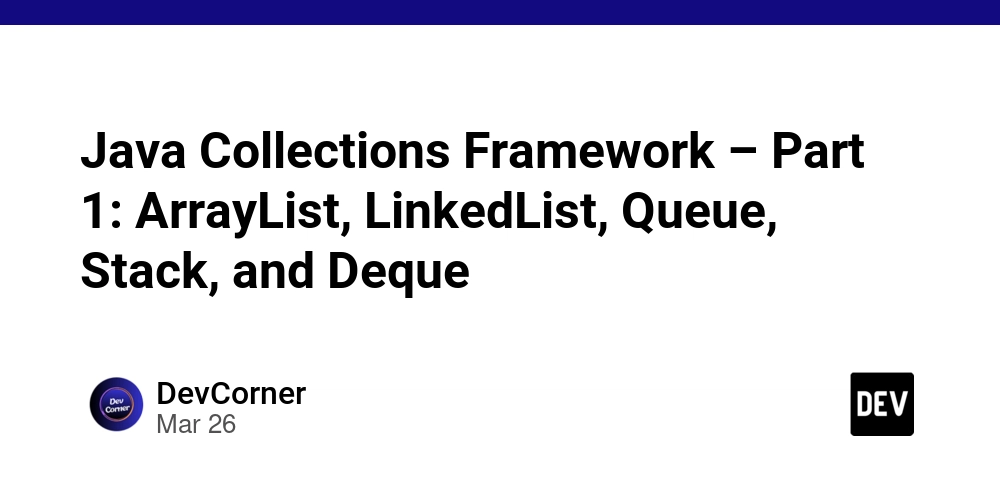
Java’s Collections Framework provides powerful data structures to manage and manipulate data efficiently. In this Part 1, we’ll cover ArrayList, LinkedList, Queue, Stack, and Deque, exploring all their methods with explanations and code snippets.
1. ArrayList in Java
ArrayList
is a dynamic array implementation that allows resizing and fast random access.
Declaration and Initialization
import java.util.ArrayList;
import java.util.List;
List<String> list = new ArrayList<>();
Methods of ArrayList
Method | Description |
---|---|
add(E e) |
Adds an element to the end. |
add(int index, E element) |
Inserts an element at the given index. |
get(int index) |
Retrieves the element at the given index. |
set(int index, E element) |
Updates the element at the specified index. |
remove(int index) |
Removes an element at the specified index. |
remove(Object o) |
Removes the first occurrence of an element. |
clear() |
Removes all elements. |
contains(Object o) |
Checks if an element is in the list. |
size() |
Returns the number of elements. |
indexOf(Object o) |
Returns the first occurrence index. |
lastIndexOf(Object o) |
Returns the last occurrence index. |
isEmpty() |
Checks if the list is empty. |
toArray() |
Converts the list to an array. |
subList(int fromIndex, int toIndex) |
Returns a portion of the list. |
sort(Comparator super E> c) |
Sorts the list based on a comparator. |
retainAll(Collection> c) |
Retains only elements in the given collection. |
removeAll(Collection> c) |
Removes elements present in another collection. |
Example:
import java.util.ArrayList;
import java.util.Collections;
public class ArrayListExample {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
numbers.add(30);
numbers.add(1, 15); // Insert at index 1
System.out.println(numbers.get(2)); // 20
numbers.remove(0); // Remove first element
Collections.sort(numbers); // Sort the list
System.out.println(numbers);
}
}
2. LinkedList in Java
LinkedList
is a doubly linked list implementation that provides fast insertions and deletions.
Declaration and Initialization
import java.util.LinkedList;
import java.util.List;
List<String> linkedList = new LinkedList<>();
Methods of LinkedList
Method | Description |
---|---|
add(E e) |
Adds an element at the end. |
add(int index, E element) |
Inserts an element at a given index. |
get(int index) |
Retrieves an element at an index. |
set(int index, E element) |
Replaces an element. |
remove(int index) |
Removes an element at an index. |
remove(Object o) |
Removes the first occurrence of an element. |
size() |
Returns the number of elements. |
clear() |
Clears all elements. |
contains(Object o) |
Checks if an element exists. |
isEmpty() |
Checks if the list is empty. |
toArray() |
Converts the list to an array. |
Deque-Specific Methods (Available in LinkedList)
Method | Description |
---|---|
addFirst(E e) |
Adds an element at the front. |
addLast(E e) |
Adds an element at the end. |
removeFirst() |
Removes the first element. |
removeLast() |
Removes the last element. |
getFirst() |
Retrieves the first element without removing. |
getLast() |
Retrieves the last element without removing. |
Example:
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
LinkedList<String> names = new LinkedList<>();
names.add("Alice");
names.add("Bob");
names.addFirst("John");
names.addLast("Emma");
System.out.println(names.getFirst()); // John
System.out.println(names.getLast()); // Emma
names.removeFirst();
names.removeLast();
System.out.println(names); // [Alice, Bob]
}
}
3. Queue in Java
A Queue follows FIFO (First In, First Out) order.
Declaration and Initialization
import java.util.Queue;
import java.util.LinkedList;
Queue<Integer> queue = new LinkedList<>();
Methods of Queue
Method | Description |
---|---|
offer(E e) |
Adds an element to the queue. |
poll() |
Retrieves and removes the head of the queue. |
peek() |
Retrieves the head but does not remove it. |
remove() |
Removes the head element. |
element() |
Retrieves but throws an exception if empty. |
Example:
import java.util.LinkedList;
import java.util.Queue;
public class QueueExample {
public static void main(String[] args) {
Queue<String> queue = new LinkedList<>();
queue.offer("Alice");
queue.offer("Bob");
queue.offer("Charlie");
System.out.println(queue.peek()); // Alice
queue.poll();
System.out.println(queue); // [Bob, Charlie]
}
}
4. Stack in Java
A Stack follows LIFO (Last In, First Out) order.
Declaration and Initialization
import java.util.Stack;
Stack<Integer> stack = new Stack<>();
Methods of Stack
Method | Description |
---|---|
push(E item) |
Pushes an element onto the stack. |
pop() |
Removes and returns the top element. |
peek() |
Returns the top element without removing it. |
empty() |
Checks if the stack is empty. |
search(Object o) |
Returns the 1-based position of an element. |
Example:
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
stack.push(10);
stack.push(20);
stack.push(30);
System.out.println(stack.peek()); // 30
System.out.println(stack.pop()); // 30
System.out.println(stack.empty()); // false
}
}
5. Deque in Java
A Deque (Double-Ended Queue) allows insertions/removals at both ends.
Declaration and Initialization
import java.util.Deque;
import java.util.LinkedList;
Deque<Integer> deque = new LinkedList<>();
Methods of Deque
Method | Description |
---|---|
addFirst(E e) |
Adds an element at the front. |
addLast(E e) |
Adds an element at the end. |
removeFirst() |
Removes the first element. |
removeLast() |
Removes the last element. |
getFirst() |
Retrieves the first element. |
getLast() |
Retrieves the last element. |
Example:
import java.util.Deque;
import java.util.LinkedList;
public class DequeExample {
public static void main(String[] args) {
Deque<String> deque = new LinkedList<>();
deque.addFirst("Alice");
deque.addLast("Bob");
deque.addLast("Charlie");
System.out.println(deque.getFirst()); // Alice
System.out.println(deque.getLast()); // Charlie
}
}