Have you ever needed to periodically fetch data from a remote API in your React Native app? Maybe you're building a chat client that needs to poll for new messages, or a dashboard that refreshes every few seconds with updated metrics? Most developers end up writing something like this: useEffect(() => { const interval = setInterval(async () => { const response = await fetch("https://your-api.com/status"); const data = await response.json(); // do something }, 1000); return () => clearInterval(interval); }, []); This works... until it doesn’t: Multiple polling tasks run in parallel and get messy ☠️ You start hitting battery and performance issues
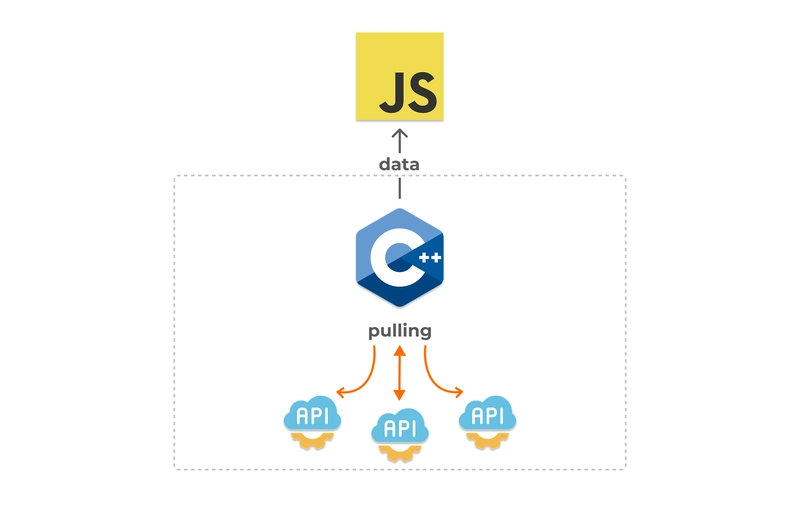
Have you ever needed to periodically fetch data from a remote API in your React Native app? Maybe you're building a chat client that needs to poll for new messages, or a dashboard that refreshes every few seconds with updated metrics?
Most developers end up writing something like this:
useEffect(() => {
const interval = setInterval(async () => {
const response = await fetch("https://your-api.com/status");
const data = await response.json();
// do something
}, 1000);
return () => clearInterval(interval);
}, []);
This works... until it doesn’t:
- Multiple polling tasks run in parallel and get messy ☠️
- You start hitting battery and performance issues