Introducing ConsoleInk.NET: Streaming Markdown Rendering for .NET Console Apps
Today I'm excited to announce the first release of ConsoleInk.NET, a lightweight, zero-dependency .NET library for rendering Markdown directly in console applications using ANSI formatting. What is ConsoleInk.NET? ConsoleInk.NET transforms Markdown text into beautifully formatted console output, with a streaming-first design that processes content incrementally as it arrives - perfect for real-time display of documentation, chat messages, or any text that benefits from Markdown's readability. // Simple example string markdown = "# Hello Console!\nThis is **bold** and *italic* text."; MarkdownConsole.Render(markdown, Console.Out); Why Another Markdown Library? Most Markdown libraries focus on HTML conversion or require complex dependency chains. ConsoleInk.NET addresses a specific need: direct console rendering with: Zero external dependencies - just the .NET BCL Streaming-first architecture - process content as it arrives ANSI formatting - for modern terminal experiences Simple API - intuitive for both one-shot and streaming scenarios Features ConsoleInk.NET supports a wide range of Markdown syntax: Text Formatting: Headings, paragraphs, emphasis (bold, italic) Structured Content: Lists (ordered, unordered, task lists), blockquotes Code: Inline code, indented code blocks, fenced code blocks Links: Inline links with true OSC-8 hyperlinks (terminal-dependent) Tables: Basic GFM table support Theming: Customizable ANSI color schemes Hyperlinks One standout feature is support for true clickable hyperlinks in terminals that support the OSC-8 spec: var options = new MarkdownRenderOptions { UseHyperlinks = true }; string markdown = "Visit [GitHub](https://github.com/) for more info."; MarkdownConsole.Render(markdown, Console.Out, options); In terminals without hyperlink support, it falls back to styled text with the URL displayed in parentheses. Code Blocks Both indented and fenced code blocks are supported with careful handling of line breaks and indentation: // Example showing fenced code blocks string markdown = @" # Code Example csharp // This will be rendered with proper spacing if (x > 0) { Console.WriteLine(""Hello""); } "; MarkdownConsole.Render(markdown, Console.Out); What's Not Supported (Yet) Advanced HTML: HTML tags are stripped by default Syntax Highlighting: Code blocks are styled but not syntax-highlighted Math Notation: LaTeX/MathJax content is rendered as plain text Diagrams: Mermaid and similar diagram markup is displayed as plain text Implementation Approach ConsoleInk.NET uses a state machine processor that handles Markdown incrementally and renders directly as it processes content. This approach is optimized for line-by-line rendering and enables live display of content as it arrives. The streaming design follows a clean TextWriter-like pattern: // Streaming example using (var writer = new MarkdownConsoleWriter(Console.Out, options)) { // Process content as it arrives writer.WriteLine("# Streaming Demo"); writer.WriteLine("Content is processed **incrementally**."); // Disposal handles completion automatically } Getting Started Install from NuGet: dotnet add package ConsoleInk.Net --version 0.1.2 Basic usage: using ConsoleInk.Net; // One-shot rendering string markdown = "# Hello\n\nThis is a **test**."; MarkdownConsole.Render(markdown, Console.Out); // With custom options var options = new MarkdownRenderOptions { ConsoleWidth = 80, Theme = ConsoleTheme.Default, UseHyperlinks = true }; MarkdownConsole.Render(markdown, Console.Out, options); Future Plans This is just the beginning for ConsoleInk.NET. Future releases will add: A dedicated PowerShell module for seamless PS integration Extended GFM support More theming options Improved table handling Better rendering of complex nested structures Try It Today ConsoleInk.NET is open source (MIT license) and available on NuGet. The source code is on GitHub. Give it a try for your next console application that needs beautiful documentation, help text, or any Markdown-formatted content! Have you used Markdown rendering in console applications before? What features would you like to see in future releases? Let me know in the comments!
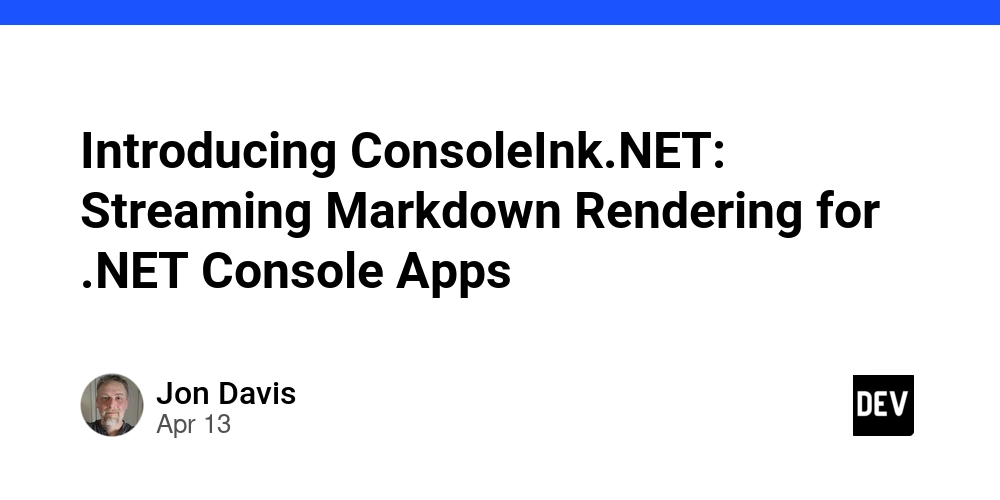
Today I'm excited to announce the first release of ConsoleInk.NET, a lightweight, zero-dependency .NET library for rendering Markdown directly in console applications using ANSI formatting.
What is ConsoleInk.NET?
ConsoleInk.NET transforms Markdown text into beautifully formatted console output, with a streaming-first design that processes content incrementally as it arrives - perfect for real-time display of documentation, chat messages, or any text that benefits from Markdown's readability.
// Simple example
string markdown = "# Hello Console!\nThis is **bold** and *italic* text.";
MarkdownConsole.Render(markdown, Console.Out);
Why Another Markdown Library?
Most Markdown libraries focus on HTML conversion or require complex dependency chains. ConsoleInk.NET addresses a specific need: direct console rendering with:
- Zero external dependencies - just the .NET BCL
- Streaming-first architecture - process content as it arrives
- ANSI formatting - for modern terminal experiences
- Simple API - intuitive for both one-shot and streaming scenarios
Features
ConsoleInk.NET supports a wide range of Markdown syntax:
- Text Formatting: Headings, paragraphs, emphasis (bold, italic)
- Structured Content: Lists (ordered, unordered, task lists), blockquotes
- Code: Inline code, indented code blocks, fenced code blocks
- Links: Inline links with true OSC-8 hyperlinks (terminal-dependent)
- Tables: Basic GFM table support
- Theming: Customizable ANSI color schemes
Hyperlinks
One standout feature is support for true clickable hyperlinks in terminals that support the OSC-8 spec:
var options = new MarkdownRenderOptions { UseHyperlinks = true };
string markdown = "Visit [GitHub](https://github.com/) for more info.";
MarkdownConsole.Render(markdown, Console.Out, options);
In terminals without hyperlink support, it falls back to styled text with the URL displayed in parentheses.
Code Blocks
Both indented and fenced code blocks are supported with careful handling of line breaks and indentation:
// Example showing fenced code blocks
string markdown = @"
# Code Example
csharp
// This will be rendered with proper spacing
if (x > 0)
{
Console.WriteLine(""Hello"");
}
";
MarkdownConsole.Render(markdown, Console.Out);
What's Not Supported (Yet)
- Advanced HTML: HTML tags are stripped by default
- Syntax Highlighting: Code blocks are styled but not syntax-highlighted
- Math Notation: LaTeX/MathJax content is rendered as plain text
- Diagrams: Mermaid and similar diagram markup is displayed as plain text
Implementation Approach
ConsoleInk.NET uses a state machine processor that handles Markdown incrementally and renders directly as it processes content. This approach is optimized for line-by-line rendering and enables live display of content as it arrives.
The streaming design follows a clean TextWriter-like pattern:
// Streaming example
using (var writer = new MarkdownConsoleWriter(Console.Out, options))
{
// Process content as it arrives
writer.WriteLine("# Streaming Demo");
writer.WriteLine("Content is processed **incrementally**.");
// Disposal handles completion automatically
}
Getting Started
Install from NuGet:
dotnet add package ConsoleInk.Net --version 0.1.2
Basic usage:
using ConsoleInk.Net;
// One-shot rendering
string markdown = "# Hello\n\nThis is a **test**.";
MarkdownConsole.Render(markdown, Console.Out);
// With custom options
var options = new MarkdownRenderOptions
{
ConsoleWidth = 80,
Theme = ConsoleTheme.Default,
UseHyperlinks = true
};
MarkdownConsole.Render(markdown, Console.Out, options);
Future Plans
This is just the beginning for ConsoleInk.NET. Future releases will add:
- A dedicated PowerShell module for seamless PS integration
- Extended GFM support
- More theming options
- Improved table handling
- Better rendering of complex nested structures
Try It Today
ConsoleInk.NET is open source (MIT license) and available on NuGet. The source code is on GitHub.
Give it a try for your next console application that needs beautiful documentation, help text, or any Markdown-formatted content!
Have you used Markdown rendering in console applications before? What features would you like to see in future releases? Let me know in the comments!