How to solve CORS error while fetching an external API? (Solution Explained)
You are building a front end that calls an external API directly from the browser and then sends the data to your backend for storage. Everything appears fine during local testing, especially when you run a “disable CORS” browser extension, but once the app runs on your production domain every request fails with a CORS error and nothing reaches your backend. Problem Statement In production, the developer console reports that no Access‑Control‑Allow‑Origin header is present on the resource you are fetching. You tried relying on the extension that turns CORS checks off, which fixes the issue only inside your own browser session, and you also added Access‑Control‑Allow‑Origin: * to the headers you pass into fetch, but the browser still blocks the response. Both attempts fail because the header must arrive in the response from the external API, not in the request you send, and that API simply does not include it. No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:3000' is therefore not allowed access. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled. Solving "How to solve CORS error while fetching an external API?" CORS policy happens entirely inside the browser. When a page at https://your‑frontend.app requests data from https://SomeExternalAPI.com, the browser expects the response to contain an Access‑Control‑Allow‑Origin header that echoes back your origin or uses the wildcard *. If that header is absent, the browser discards the response before JavaScript can read it. Because you do not control the external API, the only workable solutions are to move the request to a server you do control or to use a proxy to make the request for you. One option is to relay the request through your backend. The browser communicates with your own origin, so CORS becomes a non‑issue: // /api/external/users.js – Express relay example import express from "express"; const router = express.Router(); router.get("/users", async (_, res) => { const upstream = await fetch("https://SomeExternalAPI.com/users"); const data = await upstream.json(); res.json(data); // same‑origin response: no CORS check }); export default router; On the client you simply call that internal endpoint: const users = await fetch("/api/external/users").then((r) => r.json()); If you do not want any server code, you can instead forward the browser request to a CORS proxy. The proxy fetches the external API, attaches the missing header, and returns the result to the browser: const proxyUrl = "https://corsfix.com/?https://SomeExternalAPI.com/users"; const users = await fetch(proxyUrl).then((r) => r.json()); With this approach, you do not need to set up your own server. The proxy handles the CORS headers for you. Conclusion The external API blocks your front end because it never supplies the Access‑Control‑Allow‑Origin header, and modern browsers strictly enforce that rule. Local extensions and request‑side header tweaks do not help real users. A reliable production fix requires either routing the call through your own backend, which returns the response as same‑origin, or using a CORS proxy that inserts the proper header on your behalf. Try Corsfix with unlimited monthly requests for free in your development environment. When you are ready to deploy, upgrade to a paid plan for production use.
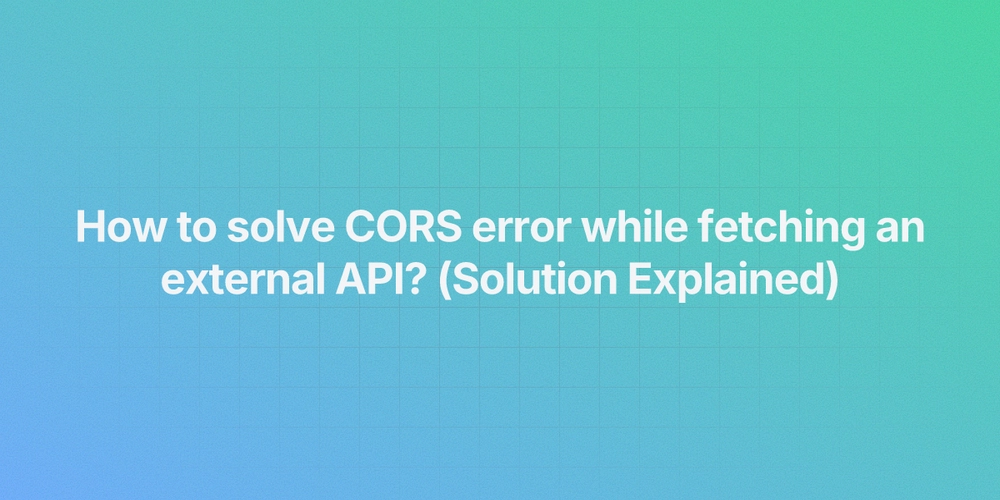
You are building a front end that calls an external API directly from the browser and then sends the data to your backend for storage. Everything appears fine during local testing, especially when you run a “disable CORS” browser extension, but once the app runs on your production domain every request fails with a CORS error and nothing reaches your backend.
Problem Statement
In production, the developer console reports that no Access‑Control‑Allow‑Origin
header is present on the resource you are fetching. You tried relying on the extension that turns CORS checks off, which fixes the issue only inside your own browser session, and you also added Access‑Control‑Allow‑Origin: *
to the headers you pass into fetch
, but the browser still blocks the response. Both attempts fail because the header must arrive in the response from the external API, not in the request you send, and that API simply does not include it.
No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:3000' is therefore not allowed access. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.
Solving "How to solve CORS error while fetching an external API?"
CORS policy happens entirely inside the browser. When a page at https://your‑frontend.app
requests data from https://SomeExternalAPI.com
, the browser expects the response to contain an Access‑Control‑Allow‑Origin
header that echoes back your origin or uses the wildcard *
. If that header is absent, the browser discards the response before JavaScript can read it. Because you do not control the external API, the only workable solutions are to move the request to a server you do control or to use a proxy to make the request for you.
One option is to relay the request through your backend. The browser communicates with your own origin, so CORS becomes a non‑issue:
// /api/external/users.js – Express relay example
import express from "express";
const router = express.Router();
router.get("/users", async (_, res) => {
const upstream = await fetch("https://SomeExternalAPI.com/users");
const data = await upstream.json();
res.json(data); // same‑origin response: no CORS check
});
export default router;
On the client you simply call that internal endpoint:
const users = await fetch("/api/external/users").then((r) => r.json());
If you do not want any server code, you can instead forward the browser request to a CORS proxy. The proxy fetches the external API, attaches the missing header, and returns the result to the browser:
const proxyUrl = "https://corsfix.com/?https://SomeExternalAPI.com/users";
const users = await fetch(proxyUrl).then((r) => r.json());
With this approach, you do not need to set up your own server. The proxy handles the CORS headers for you.
Conclusion
The external API blocks your front end because it never supplies the Access‑Control‑Allow‑Origin
header, and modern browsers strictly enforce that rule. Local extensions and request‑side header tweaks do not help real users. A reliable production fix requires either routing the call through your own backend, which returns the response as same‑origin, or using a CORS proxy that inserts the proper header on your behalf.
Try Corsfix with unlimited monthly requests for free in your development environment. When you are ready to deploy, upgrade to a paid plan for production use.