How to Build a REST API with Node.js
Title: Building a REST API with Node.js: A Comprehensive Guide Introduction Node.js is one of the most popular technologies used in modern web development today. It is an open-source, cross-platform JavaScript runtime environment that allows developers to build server-side and networking applications. In this blog post, we will walk you through the steps to build a REST API using Node.js. We will cover setting up Express, defining routes, and using middleware. Setting Up Express Express.js is a fast, unopinionated, minimalist web framework for Node.js. It is the de facto standard server framework for Node.js. To start with, you need to have Node.js and npm (Node Package Manager) installed on your machine. You can download it from the official website: https://nodejs.org/en/download/. Once you have Node.js installed, create a new folder for your project, navigate into it, and initiate a new Node.js project using the following command: npm init -y Now, install Express using npm: npm install express --save This command will install Express in your project and save it in your dependencies. Defining Routes After setting up Express, we need to set up our routes. Routes are the endpoints that define how an API responds to client requests. In an Express app, we define routes using methods of an Express application instance. Create a new file named 'app.js' in your project root and require the express module: const express = require('express'); const app = express(); const port = 3000; Now, let's define a basic route: app.get('/', (req, res) => { res.send('Hello World!') }) This code creates a route handler for HTTP GET requests to the application’s home page. The callback function takes a request and a response object as arguments, and simply responds by sending a text message. Using Middleware Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. Middleware functions can perform the following tasks: Execute any code. Make changes to the request and the response objects. End the request-response cycle. Call the next middleware function in the stack. To use a middleware, we can use the app.use() function. Here's an example of how to use a middleware function at the application level: app.use(function (req, res, next) { console.log('Time:', Date.now()) next() }) This middleware function simply prints the current time and then passes control to the next middleware function in the stack. Conclusion Building a REST API with Node.js and Express.js is a straightforward process. This blog post only covers the basics, but there’s a lot more you can do, such as adding a database, validating user input, handling errors, and more. With a good understanding of Express and Node.js, you can build highly scalable and efficient web applications. The key is to understand how routes and middleware work, as they form the core of any Express application. Happy coding! https://youtu.be/OYkmIIKfWq4?si=xLtWbtVJTF131vqL To master Login/Signup with MERN stack, please have a look this video
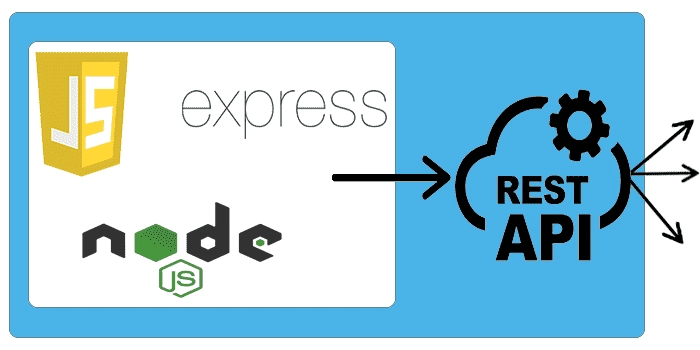
Title: Building a REST API with Node.js: A Comprehensive Guide
Introduction
Node.js is one of the most popular technologies used in modern web development today. It is an open-source, cross-platform JavaScript runtime environment that allows developers to build server-side and networking applications. In this blog post, we will walk you through the steps to build a REST API using Node.js. We will cover setting up Express, defining routes, and using middleware.
- Setting Up Express
Express.js is a fast, unopinionated, minimalist web framework for Node.js. It is the de facto standard server framework for Node.js.
To start with, you need to have Node.js and npm (Node Package Manager) installed on your machine. You can download it from the official website: https://nodejs.org/en/download/.
Once you have Node.js installed, create a new folder for your project, navigate into it, and initiate a new Node.js project using the following command:
npm init -y
Now, install Express using npm:
npm install express --save
This command will install Express in your project and save it in your dependencies.
- Defining Routes
After setting up Express, we need to set up our routes. Routes are the endpoints that define how an API responds to client requests. In an Express app, we define routes using methods of an Express application instance.
Create a new file named 'app.js' in your project root and require the express module:
const express = require('express');
const app = express();
const port = 3000;
Now, let's define a basic route:
app.get('/', (req, res) => {
res.send('Hello World!')
})
This code creates a route handler for HTTP GET requests to the application’s home page. The callback function takes a request and a response object as arguments, and simply responds by sending a text message.
- Using Middleware
Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. Middleware functions can perform the following tasks:
- Execute any code.
- Make changes to the request and the response objects.
- End the request-response cycle.
- Call the next middleware function in the stack.
To use a middleware, we can use the app.use() function. Here's an example of how to use a middleware function at the application level:
app.use(function (req, res, next) {
console.log('Time:', Date.now())
next()
})
This middleware function simply prints the current time and then passes control to the next middleware function in the stack.
Conclusion
Building a REST API with Node.js and Express.js is a straightforward process. This blog post only covers the basics, but there’s a lot more you can do, such as adding a database, validating user input, handling errors, and more. With a good understanding of Express and Node.js, you can build highly scalable and efficient web applications. The key is to understand how routes and middleware work, as they form the core of any Express application. Happy coding!
https://youtu.be/OYkmIIKfWq4?si=xLtWbtVJTF131vqL
To master Login/Signup with MERN stack, please have a look this video