How JavaScript Works
How JavaScript Works JavaScript is a synchronous, single-threaded programming language, meaning it executes code sequentially, one operation at a time, within a single execution thread. To understand how JavaScript processes code, we need to explore its Execution Context and the Call Stack. Execution Context Everything in JavaScript happens inside an Execution Context. When we run a JavaScript program, a new Global Execution Context (GEC) is created. This context consists of two phases: 1. Memory Creation Phase All variables and functions are stored in memory as key-value pairs. Variables are assigned a special placeholder value: undefined. Function declarations are stored as entire function definitions. Example: console.log(x); // Undefined var x = 10; function greet() { console.log("Hello, World!"); } Before executing the code, JavaScript sets up memory: Memory: x -> undefined greet -> function definition 2. Code Execution Phase Code is executed line by line. Variables are assigned actual values. When a function is invoked, a new Function Execution Context (FEC) is created. Once the function finishes execution, its execution context is deleted. Example: function add(a, b) { return a + b; } let result = add(5, 3); console.log(result); Steps: Global Execution Context (GEC) is created. Memory is allocated for add and result. When add(5,3) is called, a Function Execution Context (FEC) is created. Once the function returns a value, its context is removed from memory. Call Stack The Call Stack is a data structure that manages execution contexts. When a function is invoked, it is pushed onto the stack. When the function completes, it is popped off the stack. Once all functions finish execution, the Global Execution Context (GEC) is removed. Example: function first() { console.log("First function"); second(); console.log("First function again"); } function second() { console.log("Second function"); } first(); Call Stack Execution: 1. GEC is created 2. first() is called -> Pushed onto the stack 3. "First function" is logged 4. second() is called -> Pushed onto the stack 5. "Second function" is logged, second() completes -> Popped off the stack 6. "First function again" is logged, first() completes -> Popped off the stack 7. GEC is removed Final Output: First function Second function First function again Summary JavaScript is synchronous and single-threaded. Execution Context has two phases: Memory Creation and Code Execution. Call Stack maintains function execution order. When execution finishes, the Global Execution Context is removed. Understanding these fundamentals is key to mastering JavaScript, especially when dealing with asynchronous operations like callbacks, promises, and the event loop.
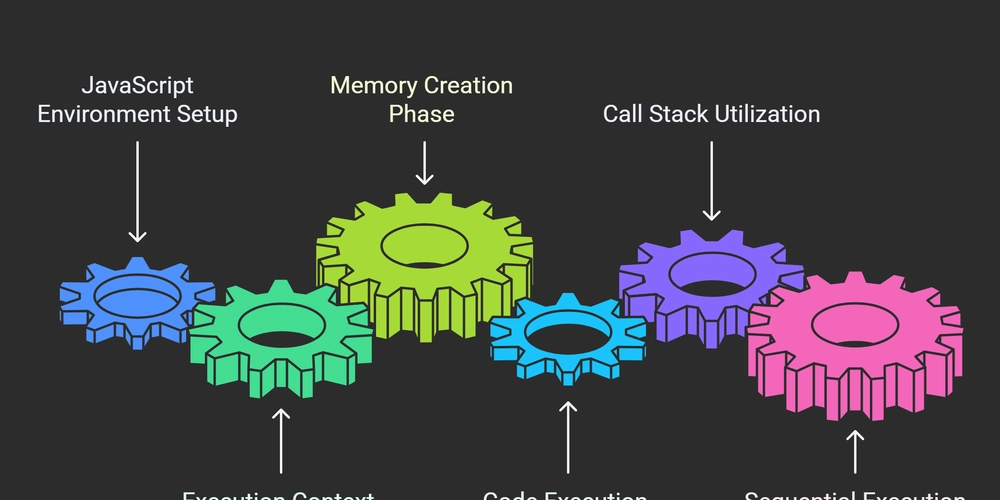
How JavaScript Works
JavaScript is a synchronous, single-threaded programming language, meaning it executes code sequentially, one operation at a time, within a single execution thread. To understand how JavaScript processes code, we need to explore its Execution Context and the Call Stack.
Execution Context
Everything in JavaScript happens inside an Execution Context. When we run a JavaScript program, a new Global Execution Context (GEC) is created. This context consists of two phases:
1. Memory Creation Phase
- All variables and functions are stored in memory as key-value pairs.
- Variables are assigned a special placeholder value:
undefined
. - Function declarations are stored as entire function definitions.
Example:
console.log(x); // Undefined
var x = 10;
function greet() {
console.log("Hello, World!");
}
Before executing the code, JavaScript sets up memory:
Memory:
x -> undefined
greet -> function definition
2. Code Execution Phase
- Code is executed line by line.
- Variables are assigned actual values.
- When a function is invoked, a new Function Execution Context (FEC) is created.
- Once the function finishes execution, its execution context is deleted.
Example:
function add(a, b) {
return a + b;
}
let result = add(5, 3);
console.log(result);
Steps:
- Global Execution Context (GEC) is created.
-
Memory is allocated for
add
andresult
. - When
add(5,3)
is called, a Function Execution Context (FEC) is created. - Once the function returns a value, its context is removed from memory.
Call Stack
The Call Stack is a data structure that manages execution contexts.
- When a function is invoked, it is pushed onto the stack.
- When the function completes, it is popped off the stack.
- Once all functions finish execution, the Global Execution Context (GEC) is removed.
Example:
function first() {
console.log("First function");
second();
console.log("First function again");
}
function second() {
console.log("Second function");
}
first();
Call Stack Execution:
1. GEC is created
2. first() is called -> Pushed onto the stack
3. "First function" is logged
4. second() is called -> Pushed onto the stack
5. "Second function" is logged, second() completes -> Popped off the stack
6. "First function again" is logged, first() completes -> Popped off the stack
7. GEC is removed
Final Output:
First function
Second function
First function again
Summary
- JavaScript is synchronous and single-threaded.
- Execution Context has two phases: Memory Creation and Code Execution.
- Call Stack maintains function execution order.
- When execution finishes, the Global Execution Context is removed.
Understanding these fundamentals is key to mastering JavaScript, especially when dealing with asynchronous operations like callbacks, promises, and the event loop.