HarmonyOS NEXT Practical: Counter
Goal: Set up a counter by clicking on the plus or minus digits. Knowledge points: Counter Counter component, providing corresponding increase or decrease counting operations. Counter attribute enableInc(value: boolean) //Set the add button to disable or enable. Default value: true enableDec(value: boolean)//Set the decrease button to disable or enable. Default value: true Counter event onInc(event: () => void) //Monitor the event of increasing numerical values. onDec(event: () => void) //Monitor the event of decreasing numerical values. CounterComponent Define the counter component. CounterComponent({ options: CounterOptions }) CounterOptions defines the type and specific style parameters of Counter. CounterOptions attribute Type: Specify the type of the current Counter. Direction: Layout direction. Default value: Direction.Auto Number Options: Styles for list and compact counters. InlineOptions: The style of a regular numeric inline adjustable counter. DateOptions: Style of date type inline counter. CounterType CounterType specifies the type of Counter, such as column phenotype Counter. CommonOptions CommonOptions defines the common properties and events of Counter. CommonOptions attribute Focused: Set whether Counter can focus. Explanation: This attribute is effective for list and compact Counter. Default value: true。 true: Set Counter to focus; false: Setting Counter cannot focus. Step: Set the step size for Counter. Value range: integers greater than or equal to 1. Default value: 1 OnOverIncrease: The add button when the mouse enters or exits the Counter component triggers this callback. OnOverDecrease: The decrease button when the mouse enters or exits the Counter component triggers this callback. InlineStyleOptions InlineStyleOptions defines the properties and events of Inline Style (numerical inline counter). InlineStyleOptions property value: Set the initial value of Counter. Default value: 0 min: Set the minimum value for Counter. Default value: 0 max: Set the maximum value of Counter. Default value: 999 textWidth: Set the width of numerical text. Default value: 0 onChange: When the value changes, return the current value. DateStyleOptions DateStyleOptions defines the properties and events of Date style (date inline Counter). DateStyleOptions property year: Set date inline initial year. Default value: 1 month: Set the date inline type initial month. Default value: 1 day: Set an inline initial date. Default value: 1 onDateChange (date: DateData)=>void: When the date changes, return the current date. date: The currently displayed date value. DateData DateData defines the universal properties and methods of Date, such as year, month, and day. DateData property year: Set date inline initial year. month: Set the date inline type initial month. day: Set an inline initial date. Actual combat:CounterDemoPage import { CounterComponent, CounterType } from '@kit.ArkUI' @Entry @Component struct CounterDemoPage { @State value: number = 1 build() { Column({ space: 20 }) { Text('计数器Demo') Counter() { Text(this.value.toString()) } .onInc(() => { this.value++ }) .onDec(() => { this.value-- }) CounterComponent({ options: { type: CounterType.COMPACT, numberOptions: { label: "数量", value: 10, min: 1, max: 100, step: 1 } } }) Text('通常用于购物车、页码等业务模块') } .height('100%') .width('100%') } }
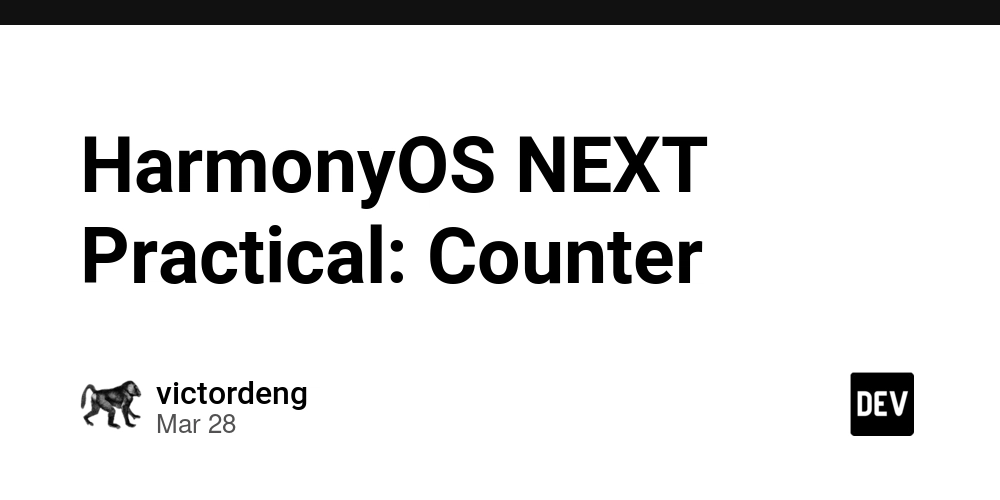
Goal: Set up a counter by clicking on the plus or minus digits.
Knowledge points:
Counter
Counter component, providing corresponding increase or decrease counting operations.
Counter attribute
enableInc(value: boolean) //Set the add button to disable or enable. Default value: true
enableDec(value: boolean)//Set the decrease button to disable or enable. Default value: true
Counter event
onInc(event: () => void) //Monitor the event of increasing numerical values.
onDec(event: () => void) //Monitor the event of decreasing numerical values.
CounterComponent
Define the counter component.
CounterComponent({ options: CounterOptions })
CounterOptions defines the type and specific style parameters of Counter.
CounterOptions attribute
- Type: Specify the type of the current Counter.
- Direction: Layout direction. Default value: Direction.Auto
- Number Options: Styles for list and compact counters.
- InlineOptions: The style of a regular numeric inline adjustable counter.
- DateOptions: Style of date type inline counter.
CounterType
CounterType specifies the type of Counter, such as column phenotype Counter.
CommonOptions
CommonOptions defines the common properties and events of Counter.
CommonOptions attribute
- Focused: Set whether Counter can focus. Explanation: This attribute is effective for list and compact Counter. Default value: true。 true: Set Counter to focus; false: Setting Counter cannot focus.
- Step: Set the step size for Counter. Value range: integers greater than or equal to 1. Default value: 1
- OnOverIncrease: The add button when the mouse enters or exits the Counter component triggers this callback.
- OnOverDecrease: The decrease button when the mouse enters or exits the Counter component triggers this callback.
InlineStyleOptions
InlineStyleOptions defines the properties and events of Inline Style (numerical inline counter).
InlineStyleOptions property
value: Set the initial value of Counter. Default value: 0
min: Set the minimum value for Counter. Default value: 0
max: Set the maximum value of Counter. Default value: 999
textWidth: Set the width of numerical text. Default value: 0
onChange: When the value changes, return the current value.
DateStyleOptions
DateStyleOptions defines the properties and events of Date style (date inline Counter).
DateStyleOptions property
year: Set date inline initial year. Default value: 1
month: Set the date inline type initial month. Default value: 1
day: Set an inline initial date. Default value: 1
onDateChange (date: DateData)=>void: When the date changes, return the current date. date: The currently displayed date value.
DateData
DateData defines the universal properties and methods of Date, such as year, month, and day.
DateData property
year: Set date inline initial year.
month: Set the date inline type initial month.
day: Set an inline initial date.
Actual combat:CounterDemoPage
import { CounterComponent, CounterType } from '@kit.ArkUI'
@Entry
@Component
struct CounterDemoPage {
@State value: number = 1
build() {
Column({ space: 20 }) {
Text('计数器Demo')
Counter() {
Text(this.value.toString())
}
.onInc(() => {
this.value++
})
.onDec(() => {
this.value--
})
CounterComponent({
options: {
type: CounterType.COMPACT,
numberOptions: {
label: "数量",
value: 10,
min: 1,
max: 100,
step: 1
}
}
})
Text('通常用于购物车、页码等业务模块')
}
.height('100%')
.width('100%')
}
}