Frontend at Scale: Building Maintainable Enterprise Apps with Angular
In today’s fast-paced web ecosystem, building scalable, maintainable, and performant enterprise-grade frontends has become a non-negotiable requirement. As applications grow in size and complexity, poorly architected frontends can lead to technical debt, sluggish performance, and challenging developer onboarding. This article is based on my experience building large-scale Angular applications for industrial IoT platforms, where real-time performance, modularity, and long-term maintainability were crucial. Architect for Scale — Not Just Features Angular supports scalable architecture out of the box, but it’s easy to fall into a monolithic trap. We follow a domain-driven approach with clear module separation using lazy loading. Example folder structure: src/app/ ├── core/ ├── shared/ ├── features/ ├── dashboard/ ├── analytics/ └── settings/ Each feature module is lazy-loaded via loadChildren and can declare its own services, guards, and routing. Use Smart-Dumb Component Patterns Smart components handle data fetching and business logic, while dumb components focus purely on presentation. This drastically improves testability and reusability. Smart Component Example: ngOnInit() { this.store.select(selectUserData).subscribe(data => this.user = data); } Dumb Component Example: Handle State Thoughtfully — You Don’t Always Need NgRx For enterprise apps, predictable state is essential, but that doesn’t mean you must default to NgRx. Start simple — use RxJS with services and move to state libraries only when needed. For mid-sized teams, NGXS or Akita can strike a better balance between structure and verbosity. Performance First — Always Use OnPush Use ChangeDetectionStrategy.OnPush for all presentational components. It avoids unnecessary DOM checks and makes change detection predictable. At an IOT application I worked, using OnPush reduced UI render time by 40% in a dashboard showing real-time metrics. Real-Time and Reactive UX If your app depends on live data (as ours did), Socket.IO + Redis Pub/Sub is a killer combo. Integrate with Angular services and update the UI via observables. CI/CD and Linting is Not Optional Use Husky + ESLint + Prettier in pre-commit hooks. Set up GitHub Actions or Azure DevOps pipelines to auto-run tests, build artifacts, and deploy to environments. Think Global From Day One For multilingual support, structure your i18n translation keys early. Use a dynamic translation service and split translation files per feature module. Protect Your App — Security Considerations Use Angular’s built-in DomSanitizer, always sanitize third-party inputs, and implement granular route guards. Build a proper RBAC (Role-Based Access Control) system if your app has role-specific UIs. Logging and Monitoring Instrument logs to Application Insights (Azure) or CloudWatch (AWS). Track route changes, API failures, user drop-offs, and custom metrics for dashboards. Keep Your UX Future-Proof Introduce feature flags using ngx-feature-toggle, track usage metrics, and incrementally deprecate legacy features. Conclusion: Scale is a Mindset Building scalable Angular apps isn’t about picking the latest libraries — it’s about designing for change. Build for readability, adaptability, and modularity. Use tools, conventions, and patterns that help your team move fast without breaking things. Further Reading — Angular Change Detection — State Management in Angular — High Performance Angular Apps
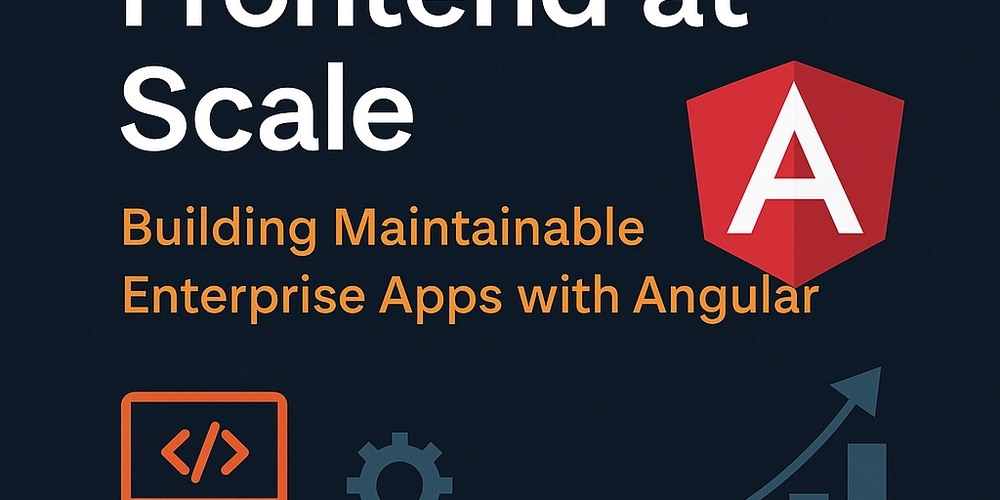
In today’s fast-paced web ecosystem, building scalable, maintainable, and performant enterprise-grade frontends has become a non-negotiable requirement. As applications grow in size and complexity, poorly architected frontends can lead to technical debt, sluggish performance, and challenging developer onboarding.
This article is based on my experience building large-scale Angular applications for industrial IoT platforms, where real-time performance, modularity, and long-term maintainability were crucial.
- Architect for Scale — Not Just Features Angular supports scalable architecture out of the box, but it’s easy to fall into a monolithic trap. We follow a domain-driven approach with clear module separation using lazy loading.
Example folder structure:
src/app/
├── core/
├── shared/
├── features/
├── dashboard/
├── analytics/
└── settings/
Each feature module is lazy-loaded via loadChildren
and can declare its own services, guards, and routing.
- Use Smart-Dumb Component Patterns Smart components handle data fetching and business logic, while dumb components focus purely on presentation. This drastically improves testability and reusability.
Smart Component Example:
ngOnInit() {
this.store.select(selectUserData).subscribe(data => this.user = data);
}
Dumb Component Example:
- Handle State Thoughtfully — You Don’t Always Need NgRx For enterprise apps, predictable state is essential, but that doesn’t mean you must default to NgRx. Start simple — use RxJS with services and move to state libraries only when needed.
For mid-sized teams, NGXS or Akita can strike a better balance between structure and verbosity.
- Performance First — Always Use OnPush
Use
ChangeDetectionStrategy.OnPush
for all presentational components. It avoids unnecessary DOM checks and makes change detection predictable.
At an IOT application I worked, using OnPush reduced UI render time by 40% in a dashboard showing real-time metrics.
Real-Time and Reactive UX
If your app depends on live data (as ours did), Socket.IO + Redis Pub/Sub is a killer combo. Integrate with Angular services and update the UI via observables.CI/CD and Linting is Not Optional
Use Husky + ESLint + Prettier in pre-commit hooks.
Set up GitHub Actions or Azure DevOps pipelines to auto-run tests, build artifacts, and deploy to environments.Think Global From Day One
For multilingual support, structure youri18n
translation keys early.
Use a dynamic translation service and split translation files per feature module.Protect Your App — Security Considerations
Use Angular’s built-in DomSanitizer, always sanitize third-party inputs, and implement granular route guards. Build a proper RBAC (Role-Based Access Control) system if your app has role-specific UIs.Logging and Monitoring
Instrument logs to Application Insights (Azure) or CloudWatch (AWS). Track route changes, API failures, user drop-offs, and custom metrics for dashboards.Keep Your UX Future-Proof
Introduce feature flags usingngx-feature-toggle
, track usage metrics, and incrementally deprecate legacy features.
Conclusion: Scale is a Mindset
Building scalable Angular apps isn’t about picking the latest libraries — it’s about designing for change. Build for readability, adaptability, and modularity. Use tools, conventions, and patterns that help your team move fast without breaking things.
Further Reading
— Angular Change Detection
— State Management in Angular
— High Performance Angular Apps