Flawfinder for Infrastructure Security: Practical Implementation Guide
Security in infrastructure development has evolved dramatically in recent years, with Static Application Security Testing (SAST) tools becoming essential components in modern development pipelines. Among these tools, Flawfinder stands out for its specialized approach to analyzing C/C++ code — languages that remain fundamental to many critical infrastructure components. This article explores how Flawfinder can be effectively integrated into Infrastructure as Code (IaC) workflows to strengthen overall security posture. Understanding Flawfinder's Role in Infrastructure Security Flawfinder is a Python-based static analysis tool that examines C/C++ source code to identify potential security vulnerabilities. Unlike tools requiring code compilation, Flawfinder works directly with source files, making it particularly valuable for preliminary analyses or situations where compilation isn't feasible. The tool operates by searching for patterns in code that match calls to functions known to be problematic from a security perspective. For example, it flags buffer overflow-prone functions like strcpy() and gets(), or format string injection vulnerabilities like unchecked printf() calls with user-controlled arguments. While primarily focused on C/C++, Flawfinder's applicability to modern infrastructure is significant because: Critical infrastructure components like web servers, databases, and network services are often written in C/C++ Network devices and hardware run firmware typically developed in C Foundational libraries even in modern cloud environments are frequently implemented in C/C++ Flawfinder Installation To install Flawfinder (a static analysis tool that examines C/C++ source code for security vulnerabilities), you can use one of several methods depending on your operating system: Linux (Using Package Manager) # Debian/Ubuntu sudo apt-get install flawfinder # Fedora sudo dnf install flawfinder # Arch Linux sudo pacman -S flawfinder Using pip (Cross-platform) pip install flawfinder From Source git clone https://github.com/david-a-wheeler/flawfinder.git cd flawfinder sudo make install After installation, verify it worked by running: flawfinder --version Hands-on Demo: Analyzing a Critical Infrastructure Component Let's examine how Flawfinder identifies vulnerabilities in a hypothetical network configuration component: // network_config.c - Network configuration component for infrastructure #include #include #include #include #define MAX_PATH 256 #define CONFIG_FILE "/etc/network/settings.conf" // Function to load network configuration from file void load_network_config(const char* config_path) { char buffer[128]; FILE *config = fopen(config_path, "r"); if (config) { char line[512]; while (fgets(line, sizeof(line), config)) { printf("Loading configuration: %s", line); } fclose(config); } else { printf("Could not open configuration file.\n"); } } // Function to execute a network configuration command void execute_network_command(const char* cmd) { printf("Executing: %s\n", cmd); system(cmd); // Vulnerability: command execution } // Function to set a new SSL certificate void set_ssl_certificate(const char* cert_path) { char command[1024]; sprintf(command, "install_cert.sh %s", cert_path); // Vulnerability: format string execute_network_command(command); } // Function to process user input data void process_user_input(const char* input) { char buffer[64]; strcpy(buffer, input); // Vulnerability: buffer overflow if (strncmp(buffer, "RESET", 5) == 0) { execute_network_command("service network restart"); } } // Main function that configures network devices int main(int argc, char *argv[]) { if (argc < 2) { printf("Usage: %s [arguments...]\n", argv[0]); return 1; } char log_file[MAX_PATH]; sprintf(log_file, "/var/log/netconfig_%s.log", getenv("USER")); if (strcmp(argv[1], "configure") == 0) { load_network_config(CONFIG_FILE); } else if (strcmp(argv[1], "set-cert") == 0 && argc > 2) { set_ssl_certificate(argv[2]); } else if (strcmp(argv[1], "execute") == 0 && argc > 2) { execute_network_command(argv[2]); } else if (strcmp(argv[1], "process") == 0 && argc > 2) { process_user_input(argv[2]); } return 0; } Running Flawfinder Analysis To analyze this code with Flawfinder, we would run: flawfinder network_config.c The output would reveal several vulnerabilities: Flawfinder version 2.0.19, (C) 2001-2019 David A. Wheeler. Number of rules (primarily dangerous function names) in C/C++ ruleset: 222 Examining network_config.c network_config.c:29: [4] (shell) system: This causes a new program to execute and is difficult to use safely. Try using a library call that implements the same functionality.
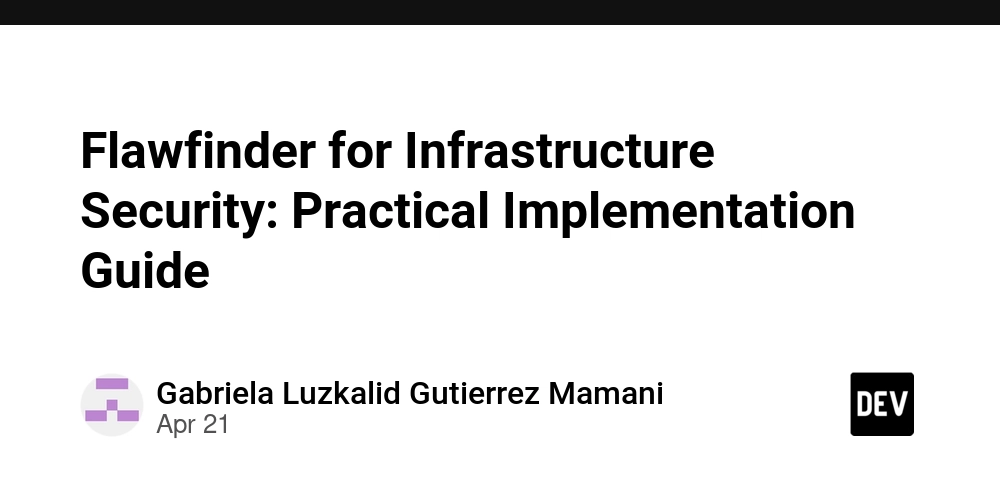
Security in infrastructure development has evolved dramatically in recent years, with Static Application Security Testing (SAST) tools becoming essential components in modern development pipelines. Among these tools, Flawfinder stands out for its specialized approach to analyzing C/C++ code — languages that remain fundamental to many critical infrastructure components. This article explores how Flawfinder can be effectively integrated into Infrastructure as Code (IaC) workflows to strengthen overall security posture.
Understanding Flawfinder's Role in Infrastructure Security
Flawfinder is a Python-based static analysis tool that examines C/C++ source code to identify potential security vulnerabilities. Unlike tools requiring code compilation, Flawfinder works directly with source files, making it particularly valuable for preliminary analyses or situations where compilation isn't feasible.
The tool operates by searching for patterns in code that match calls to functions known to be problematic from a security perspective. For example, it flags buffer overflow-prone functions like strcpy()
and gets()
, or format string injection vulnerabilities like unchecked printf()
calls with user-controlled arguments.
While primarily focused on C/C++, Flawfinder's applicability to modern infrastructure is significant because:
- Critical infrastructure components like web servers, databases, and network services are often written in C/C++
- Network devices and hardware run firmware typically developed in C
- Foundational libraries even in modern cloud environments are frequently implemented in C/C++
Flawfinder Installation
To install Flawfinder (a static analysis tool that examines C/C++ source code for security vulnerabilities), you can use one of several methods depending on your operating system:
Linux (Using Package Manager)
# Debian/Ubuntu
sudo apt-get install flawfinder
# Fedora
sudo dnf install flawfinder
# Arch Linux
sudo pacman -S flawfinder
Using pip (Cross-platform)
pip install flawfinder
From Source
git clone https://github.com/david-a-wheeler/flawfinder.git
cd flawfinder
sudo make install
After installation, verify it worked by running:
flawfinder --version
Hands-on Demo: Analyzing a Critical Infrastructure Component
Let's examine how Flawfinder identifies vulnerabilities in a hypothetical network configuration component:
// network_config.c - Network configuration component for infrastructure
#include
#include
#include
#include
#define MAX_PATH 256
#define CONFIG_FILE "/etc/network/settings.conf"
// Function to load network configuration from file
void load_network_config(const char* config_path) {
char buffer[128];
FILE *config = fopen(config_path, "r");
if (config) {
char line[512];
while (fgets(line, sizeof(line), config)) {
printf("Loading configuration: %s", line);
}
fclose(config);
} else {
printf("Could not open configuration file.\n");
}
}
// Function to execute a network configuration command
void execute_network_command(const char* cmd) {
printf("Executing: %s\n", cmd);
system(cmd); // Vulnerability: command execution
}
// Function to set a new SSL certificate
void set_ssl_certificate(const char* cert_path) {
char command[1024];
sprintf(command, "install_cert.sh %s", cert_path); // Vulnerability: format string
execute_network_command(command);
}
// Function to process user input data
void process_user_input(const char* input) {
char buffer[64];
strcpy(buffer, input); // Vulnerability: buffer overflow
if (strncmp(buffer, "RESET", 5) == 0) {
execute_network_command("service network restart");
}
}
// Main function that configures network devices
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s [arguments...]\n", argv[0]);
return 1;
}
char log_file[MAX_PATH];
sprintf(log_file, "/var/log/netconfig_%s.log", getenv("USER"));
if (strcmp(argv[1], "configure") == 0) {
load_network_config(CONFIG_FILE);
} else if (strcmp(argv[1], "set-cert") == 0 && argc > 2) {
set_ssl_certificate(argv[2]);
} else if (strcmp(argv[1], "execute") == 0 && argc > 2) {
execute_network_command(argv[2]);
} else if (strcmp(argv[1], "process") == 0 && argc > 2) {
process_user_input(argv[2]);
}
return 0;
}
Running Flawfinder Analysis
To analyze this code with Flawfinder, we would run:
flawfinder network_config.c
The output would reveal several vulnerabilities:
Flawfinder version 2.0.19, (C) 2001-2019 David A. Wheeler.
Number of rules (primarily dangerous function names) in C/C++ ruleset: 222
Examining network_config.c
network_config.c:29: [4] (shell) system:
This causes a new program to execute and is difficult to use safely.
Try using a library call that implements the same functionality.
network_config.c:35: [4] (format) sprintf:
Does not check for buffer overflows. Use snprintf instead.
network_config.c:41: [5] (buffer) strcpy:
Does not check for buffer overflows. Consider using strcpy_s,
strncpy, or strlcpy (warning: strncpy is easily misused).
network_config.c:51: [4] (format) sprintf:
Does not check for buffer overflows. Use snprintf instead.
FINAL RESULTS:
network_config.c:41: [5] (buffer) strcpy
network_config.c:29: [4] (shell) system
network_config.c:35: [4] (format) sprintf
network_config.c:51: [4] (format) sprintf
ANALYSIS SUMMARY:
Hits = 4
Lines analyzed = 67 in approximately 0.01 seconds (4876 lines/second)
Physical Source Lines of Code (SLOC) = 58
Hits@level = [0] 0 [1] 0 [2] 0 [3] 0 [4] 3 [5] 1
Hits@level+ = [0+] 4 [1+] 4 [2+] 4 [3+] 4 [4+] 4 [5+] 1
Hits/KSLOC@level+ = [0+] 68.9655 [1+] 68.9655 [2+] 68.9655 [3+] 68.9655 [4+] 68.9655 [5+] 17.2414
Minimum risk level = 1
Interpretation and Remediation
Flawfinder has identified four significant vulnerabilities:
- Buffer overflow (level 5): The strcpy() function on line 41 copies user input to a buffer without size verification.
- Command execution (level 4): Using system() on line 29 may allow arbitrary command execution.
- Format string vulnerabilities (level 4): Two instances of sprintf() on lines 35 and 51 don't check for overflows. ## Fixed Code Implementation
// Corrected version of execute_network_command
void execute_network_command(const char* cmd) {
printf("Executing: %s\n", cmd);
// Restrict commands to a predefined list of safe operations
if (strcmp(cmd, "service network restart") == 0 ||
strcmp(cmd, "ifconfig -a") == 0 ||
strncmp(cmd, "install_cert.sh /etc/certs/", 27) == 0) {
system(cmd);
} else {
printf("Unauthorized command: %s\n", cmd);
}
}
// Corrected version of set_ssl_certificate
void set_ssl_certificate(const char* cert_path) {
char command[1024];
// Validate certificate path first
if (access(cert_path, F_OK) != 0) {
printf("Error: Certificate doesn't exist\n");
return;
}
// Use snprintf to prevent overflows
snprintf(command, sizeof(command), "install_cert.sh %s", cert_path);
execute_network_command(command);
}
// Corrected version of process_user_input
void process_user_input(const char* input) {
char buffer[64];
// Use strncpy and ensure null termination
strncpy(buffer, input, sizeof(buffer) - 1);
buffer[sizeof(buffer) - 1] = '\0';
if (strncmp(buffer, "RESET", 5) == 0) {
execute_network_command("service network restart");
}
}
Strategies for Implementing Flawfinder in Infrastructure Projects
To effectively implement Flawfinder in your infrastructure workflow:
- Early Integration: Include Flawfinder analysis from the earliest development stages
- Threshold Definition: Establish acceptable severity levels for different phases (development, testing, production)
- Team Training: Ensure developers understand Flawfinder reports and how to address identified issues
- Tool Complementation: Use Flawfinder alongside other SAST tools for comprehensive coverage
- Automation: Integrate Flawfinder into your CI/CD pipeline for automatic analysis with each commit
Limitations to Consider
While Flawfinder is valuable, it's important to recognize its limitations:
- False Positives: It may generate alerts for code that is actually secure
- Limited Focus: It exclusively targets C/C++
- Shallow Analysis: It doesn't perform complete data flow analysis, which may result in undetected vulnerabilities
- Not a Replacement for Manual Reviews: Automated analysis should complement manual code reviews
Practical Example: Analyzing an Infrastructure Component
Let's examine how Flawfinder can identify vulnerabilities in a network configuration component:
Conclusion
Flawfinder represents a valuable tool in the security arsenal for teams developing infrastructure with critical C/C++ components. Its ability to quickly identify common vulnerabilities, combined with easy integration into automated pipelines, makes it an important asset for DevSecOps teams.
By incorporating static analysis at every stage of the development lifecycle, organizations can significantly improve the security of their infrastructure, reducing the risk of costly and potentially devastating vulnerabilities. The key lies in early implementation, automation, and using Flawfinder as part of a multi-layered security strategy.