Faster Database Queries With This One Trick
Ever wonder why your Laravel app slows to a crawl with just a few hundred records? The answer might surprise you—and it's hiding in plain sight. Let me take you on a journey through one of Laravel's most underappreciated features: the exists() method. This little powerhouse can dramatically improve your application's performance while making your code cleaner and more expressive. I've seen this simple change cut query times by up to 79% in real-world applications. The Hidden Cost of count() If you're like most Laravel developers, you've probably written code like this countless times: if (User::where('email', $email)->count() > 0) { // The user exists } It seems innocent enough, right? But there's a performance penalty lurking beneath the surface that can become significant as your application scales. When you call count(), Laravel generates a SQL query that looks something like this: SELECT COUNT(*) FROM users WHERE email = 'user@example.com'; This forces the database to scan through records, count them all, and return that number—even though you only care if there's at least one match. It's like asking someone to count every apple in a basket when all you need to know is whether there are any apples at all. Enter exists(): The Performance Hero
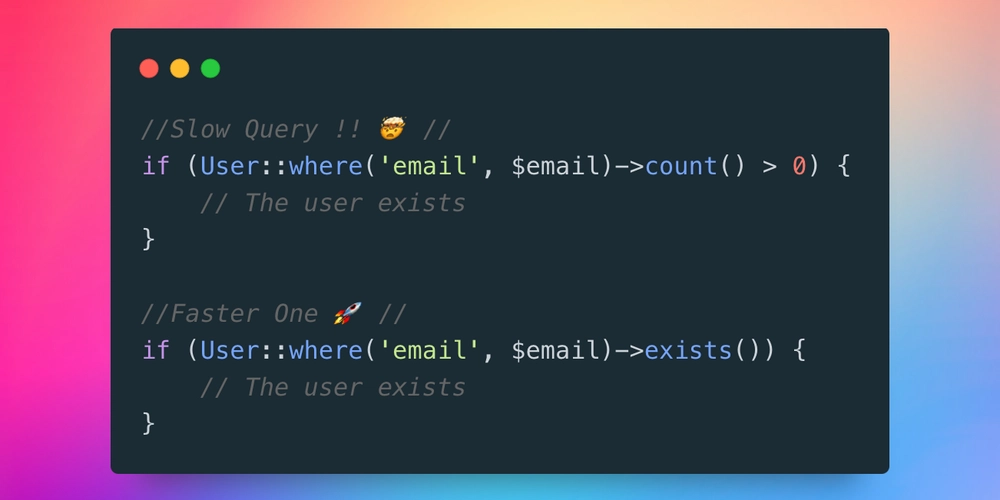
Ever wonder why your Laravel app slows to a crawl with just a few hundred records? The answer might surprise you—and it's hiding in plain sight.
Let me take you on a journey through one of Laravel's most underappreciated features: the exists()
method. This little powerhouse can dramatically improve your application's performance while making your code cleaner and more expressive. I've seen this simple change cut query times by up to 79% in real-world applications.
The Hidden Cost of count()
If you're like most Laravel developers, you've probably written code like this countless times:
if (User::where('email', $email)->count() > 0) {
// The user exists
}
It seems innocent enough, right? But there's a performance penalty lurking beneath the surface that can become significant as your application scales.
When you call count()
, Laravel generates a SQL query that looks something like this:
SELECT COUNT(*) FROM users WHERE email = 'user@example.com';
This forces the database to scan through records, count them all, and return that number—even though you only care if there's at least one match. It's like asking someone to count every apple in a basket when all you need to know is whether there are any apples at all.