Exploring the World of Game Development with Unity
Exploring the World of Game Development with Unity Game development has become one of the most exciting and rewarding fields in the tech industry. With the rise of indie games and the increasing demand for interactive content, learning game development can open up a world of opportunities. Among the many tools available, Unity stands out as one of the most popular and versatile game engines. Whether you're a beginner or an experienced developer, Unity offers a robust platform to bring your creative ideas to life. In this article, we’ll explore the world of game development with Unity, discuss its key features, and provide some practical tips to get started. Why Choose Unity for Game Development? Unity is a cross-platform game engine that powers over 50% of all mobile games and a significant portion of console and PC games. Its popularity stems from its ease of use, flexibility, and extensive community support. Here are some reasons why Unity is a great choice for game development: Cross-Platform Support: Unity allows you to build games for multiple platforms, including Windows, macOS, iOS, Android, and even consoles like PlayStation and Xbox. This means you can write your game once and deploy it across various devices with minimal changes. Rich Asset Store: Unity’s Asset Store is a treasure trove of pre-built assets, scripts, and tools that can significantly speed up your development process. Whether you need 3D models, sound effects, or plugins, the Asset Store has you covered. Strong Community and Documentation: Unity has a massive community of developers who are always willing to help. Additionally, Unity’s official documentation is comprehensive and beginner-friendly, making it easier to learn and troubleshoot. Powerful Scripting with C#: Unity uses C# as its primary scripting language, which is both powerful and easy to learn. If you’re already familiar with C#, you’ll find it easy to transition into Unity development. Getting Started with Unity To start your journey with Unity, you’ll need to download and install the Unity Hub, which allows you to manage different versions of the Unity Editor and your projects. You can download it from the official Unity website. Once you’ve installed Unity, create a new project and choose a template that suits your game idea. Unity offers templates for 2D, 3D, and other types of games. For beginners, starting with a simple 2D project is recommended. Basic Unity Concepts Before diving into coding, it’s essential to understand some fundamental Unity concepts: GameObjects: Everything in Unity is a GameObject, whether it’s a character, a light, or a camera. GameObjects are the building blocks of your game. Components: GameObjects have components that define their behavior. For example, a GameObject can have a Transform component (for position, rotation, and scale) and a Sprite Renderer component (for displaying 2D images). Scenes: A Scene is a container for your GameObjects. Think of it as a level or a screen in your game. Writing Your First Script Unity uses C# for scripting, and you can attach scripts to GameObjects to control their behavior. Let’s create a simple script to move a GameObject. In the Unity Editor, right-click in the Project window and select Create > C# Script. Name it PlayerMovement. Double-click the script to open it in your preferred code editor (Visual Studio is recommended). Here’s a basic script to move a GameObject using the arrow keys: csharp Copy using UnityEngine; public class PlayerMovement : MonoBehaviour { public float speed = 5f; void Update() { float moveX = Input.GetAxis("Horizontal") * speed * Time.deltaTime; float moveY = Input.GetAxis("Vertical") * speed * Time.deltaTime; transform.Translate(moveX, moveY, 0); } } Attach this script to a GameObject in your scene, and you’ll be able to move it using the arrow keys. This is just the beginning—Unity’s scripting capabilities allow you to create complex gameplay mechanics, AI, and more. Building Your Game Once you’ve developed your game, it’s time to build and deploy it. Unity makes this process straightforward: Go to File > Build Settings. Select your target platform (e.g., PC, Mac, Android). Click Build and choose a location to save your game. Unity will compile your game into an executable file or an app, depending on the platform you’ve chosen. Monetizing Your Skills If you’re a web developer looking to monetize your skills, game development can be a lucrative avenue. However, if you’re more interested in leveraging your web programming expertise, consider exploring opportunities like MillionFormula. This platform offers a unique way to make money using your web development skills. Check it out here. Tips for Success in Unity Game Development Start Small: Begin with simple projects to understand the basics. As you gain confidence,
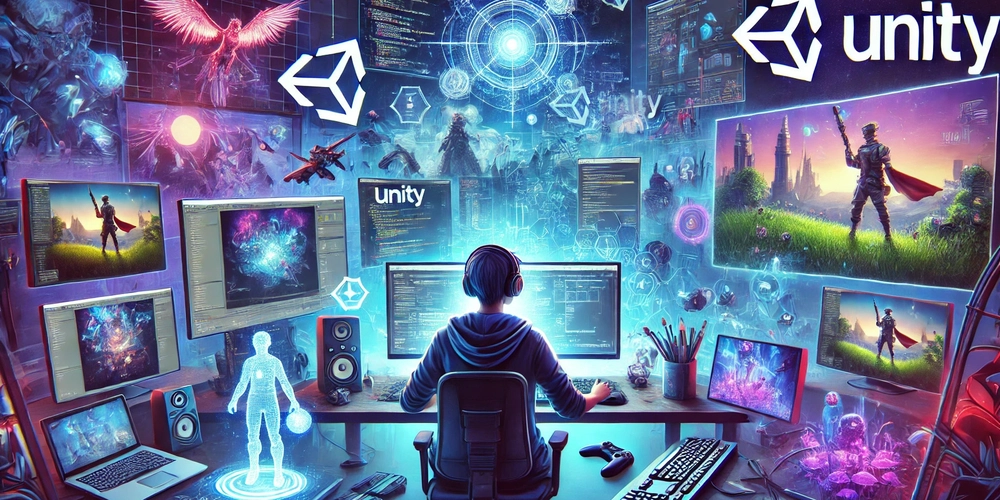
Exploring the World of Game Development with Unity
Game development has become one of the most exciting and rewarding fields in the tech industry. With the rise of indie games and the increasing demand for interactive content, learning game development can open up a world of opportunities. Among the many tools available, Unity stands out as one of the most popular and versatile game engines. Whether you're a beginner or an experienced developer, Unity offers a robust platform to bring your creative ideas to life. In this article, we’ll explore the world of game development with Unity, discuss its key features, and provide some practical tips to get started.
Why Choose Unity for Game Development?
Unity is a cross-platform game engine that powers over 50% of all mobile games and a significant portion of console and PC games. Its popularity stems from its ease of use, flexibility, and extensive community support. Here are some reasons why Unity is a great choice for game development:
- Cross-Platform Support: Unity allows you to build games for multiple platforms, including Windows, macOS, iOS, Android, and even consoles like PlayStation and Xbox. This means you can write your game once and deploy it across various devices with minimal changes.
- Rich Asset Store: Unity’s Asset Store is a treasure trove of pre-built assets, scripts, and tools that can significantly speed up your development process. Whether you need 3D models, sound effects, or plugins, the Asset Store has you covered.
- Strong Community and Documentation: Unity has a massive community of developers who are always willing to help. Additionally, Unity’s official documentation is comprehensive and beginner-friendly, making it easier to learn and troubleshoot.
- Powerful Scripting with C#: Unity uses C# as its primary scripting language, which is both powerful and easy to learn. If you’re already familiar with C#, you’ll find it easy to transition into Unity development.
Getting Started with Unity
To start your journey with Unity, you’ll need to download and install the Unity Hub, which allows you to manage different versions of the Unity Editor and your projects. You can download it from the official Unity website.
Once you’ve installed Unity, create a new project and choose a template that suits your game idea. Unity offers templates for 2D, 3D, and other types of games. For beginners, starting with a simple 2D project is recommended.
Basic Unity Concepts
Before diving into coding, it’s essential to understand some fundamental Unity concepts:
- GameObjects: Everything in Unity is a GameObject, whether it’s a character, a light, or a camera. GameObjects are the building blocks of your game.
- Components: GameObjects have components that define their behavior. For example, a GameObject can have a Transform component (for position, rotation, and scale) and a Sprite Renderer component (for displaying 2D images).
- Scenes: A Scene is a container for your GameObjects. Think of it as a level or a screen in your game.
Writing Your First Script
Unity uses C# for scripting, and you can attach scripts to GameObjects to control their behavior. Let’s create a simple script to move a GameObject.
- In the Unity Editor, right-click in the Project window and select Create > C# Script. Name it
PlayerMovement
. - Double-click the script to open it in your preferred code editor (Visual Studio is recommended).
Here’s a basic script to move a GameObject using the arrow keys:
csharp
Copy
using UnityEngine; public class PlayerMovement : MonoBehaviour { public float speed = 5f; void Update() { float moveX = Input.GetAxis("Horizontal") * speed * Time.deltaTime; float moveY = Input.GetAxis("Vertical") * speed * Time.deltaTime; transform.Translate(moveX, moveY, 0); } }
Attach this script to a GameObject in your scene, and you’ll be able to move it using the arrow keys. This is just the beginning—Unity’s scripting capabilities allow you to create complex gameplay mechanics, AI, and more.
Building Your Game
Once you’ve developed your game, it’s time to build and deploy it. Unity makes this process straightforward:
- Go to File > Build Settings.
- Select your target platform (e.g., PC, Mac, Android).
- Click Build and choose a location to save your game.
Unity will compile your game into an executable file or an app, depending on the platform you’ve chosen.
Monetizing Your Skills
If you’re a web developer looking to monetize your skills, game development can be a lucrative avenue. However, if you’re more interested in leveraging your web programming expertise, consider exploring opportunities like MillionFormula. This platform offers a unique way to make money using your web development skills. Check it out here.
Tips for Success in Unity Game Development
- Start Small: Begin with simple projects to understand the basics. As you gain confidence, you can tackle more complex games.
- Leverage the Asset Store: Don’t reinvent the wheel. Use assets and plugins from the Unity Asset Store to save time.
- Join the Community: Participate in Unity forums, Discord servers, and local meetups to learn from others and stay motivated.
- Experiment and Iterate: Game development is an iterative process. Don’t be afraid to experiment and refine your ideas.
Conclusion
Unity is a powerful and accessible tool for game development, offering endless possibilities for creativity and innovation. Whether you’re building a simple 2D game or a complex 3D experience, Unity provides the tools and resources you need to succeed. And if you’re looking to monetize your web programming skills, platforms like MillionFormula can offer alternative opportunities.
So, what are you waiting for? Dive into the world of Unity game development and start creating your dream game today!