Downloooooad PDFs without using web Browsers with python
Install the library first: (pip install googlesearch-python) import os import requests from googlesearch import search def search_pdfs(query, num_results=10): pdf_links = [] query += " filetype:pdf" for result in search(query, num_results=num_results): if result.lower().endswith(".pdf"): pdf_links.append(result) return pdf_links def download_pdf(url, save_dir="pdf_downloads"): if not os.path.exists(save_dir): os.makedirs(save_dir) try: filename = url.split("/")[-1].split("?")[0] filepath = os.path.join(save_dir, filename) response = requests.get(url, stream=True) response.raise_for_status() with open(filepath, "wb") as f: for chunk in response.iter_content(chunk_size=8192): if chunk: f.write(chunk) print(f"✅ Downloaded: {filename}") except Exception as e: print(f"❌ Failed to download {url} - {e}") Example usage if name == "main": query = input("Enter search term: ") pdf_links = search_pdfs(query, num_results=10) print("\n
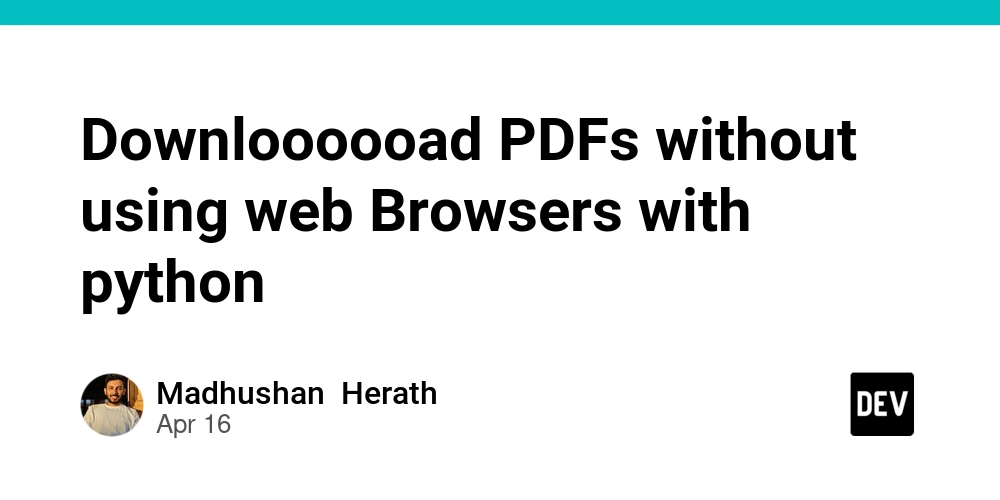
Install the library first: (pip install googlesearch-python)
import os
import requests
from googlesearch import search
def search_pdfs(query, num_results=10):
pdf_links = []
query += " filetype:pdf"
for result in search(query, num_results=num_results):
if result.lower().endswith(".pdf"):
pdf_links.append(result)
return pdf_links
def download_pdf(url, save_dir="pdf_downloads"):
if not os.path.exists(save_dir):
os.makedirs(save_dir)
try:
filename = url.split("/")[-1].split("?")[0]
filepath = os.path.join(save_dir, filename)
response = requests.get(url, stream=True)
response.raise_for_status()
with open(filepath, "wb") as f:
for chunk in response.iter_content(chunk_size=8192):
if chunk:
f.write(chunk)
print(f"✅ Downloaded: {filename}")
except Exception as e:
print(f"❌ Failed to download {url} - {e}")
Example usage
if name == "main":
query = input("Enter search term: ")
pdf_links = search_pdfs(query, num_results=10)
print("\n