Introduction Django REST Framework (DRF) is a powerful toolkit for building APIs with Django. In this tutorial, we'll cover how to install DRF and set up interactive API documentation, making your APIs more accessible and developer-friendly. Prerequisites Before we get started, ensure you have the following: Python installed (preferably Python 3.8+) Django installed (pip install django) Basic knowledge of Django Step 1: Install Django REST Framework To install DRF, open your terminal and run: pip install djangorestframework Once installed, add rest_framework to your INSTALLED_APPS in settings.py: INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'rest_framework', # Add this line ] Step 2: Setting Up API Documentation 1️⃣ Enable Browsable API DRF provides an interactive browsable API by default. To enable it, ensure your DEFAULT_RENDERER_CLASSES includes: REST_FRAMEWORK = { 'DEFAULT_RENDERER_CLASSES': ( 'rest_framework.renderers.JSONRenderer', 'rest_framework.renderers.BrowsableAPIRenderer', # Enables interactive UI ), } 2️⃣ Install drf-yasg for Swagger & ReDoc Documentation To generate API documentation, install drf-yasg: pip install drf-yasg Then, update your urls.py: from django.urls import path, re_path from rest_framework import permissions from drf_yasg.views import get_schema_view from drf_yasg import openapi schema_view = get_schema_view( openapi.Info( title="My API", default_version='v1', description="API documentation", ), public=True, permission_classes=(permissions.AllowAny,), ) urlpatterns = [ path('swagger/', schema_view.with_ui('swagger', cache_timeout=0), name='schema-swagger-ui'), path('redoc/', schema_view.with_ui('redoc', cache_timeout=0), name='schema-redoc'), ] Step 3: Run and Test the API Docs Start the Django development server: python manage.py runserver Now, visit: Swagger UI: http://127.0.0.1:8000/swagger/ ReDoc UI: http://127.0.0.1:8000/redoc/ Conclusion You have successfully installed Django REST Framework and set up interactive API documentation. This setup will help developers explore your API seamlessly.
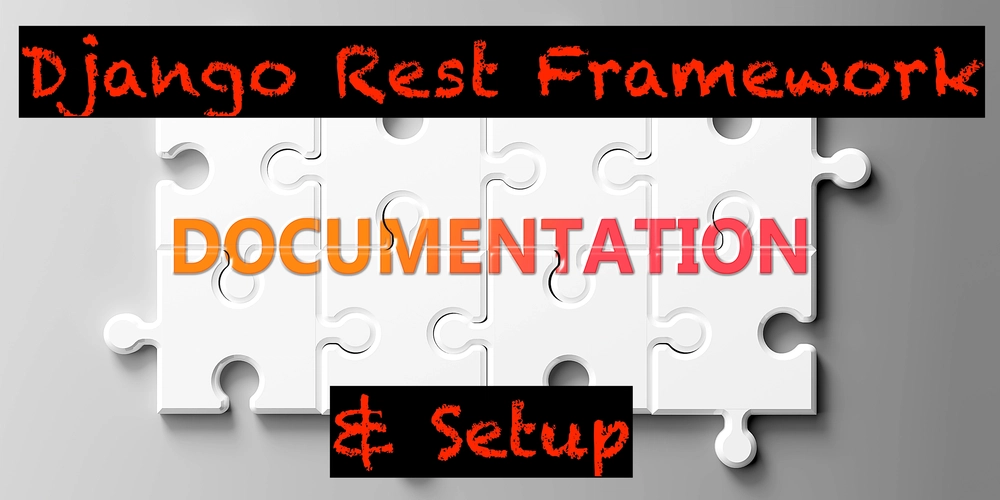
Introduction
Django REST Framework (DRF) is a powerful toolkit for building APIs with Django. In this tutorial, we'll cover how to install DRF and set up interactive API documentation, making your APIs more accessible and developer-friendly.
Prerequisites
Before we get started, ensure you have the following:
- Python installed (preferably Python 3.8+)
- Django installed (
pip install django
) - Basic knowledge of Django
Step 1: Install Django REST Framework
To install DRF, open your terminal and run:
pip install djangorestframework
Once installed, add rest_framework
to your INSTALLED_APPS in settings.py
:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework', # Add this line
]
Step 2: Setting Up API Documentation
1️⃣ Enable Browsable API
DRF provides an interactive browsable API by default. To enable it, ensure your DEFAULT_RENDERER_CLASSES
includes:
REST_FRAMEWORK = {
'DEFAULT_RENDERER_CLASSES': (
'rest_framework.renderers.JSONRenderer',
'rest_framework.renderers.BrowsableAPIRenderer', # Enables interactive UI
),
}
2️⃣ Install drf-yasg for Swagger & ReDoc Documentation
To generate API documentation, install drf-yasg
:
pip install drf-yasg
Then, update your urls.py
:
from django.urls import path, re_path
from rest_framework import permissions
from drf_yasg.views import get_schema_view
from drf_yasg import openapi
schema_view = get_schema_view(
openapi.Info(
title="My API",
default_version='v1',
description="API documentation",
),
public=True,
permission_classes=(permissions.AllowAny,),
)
urlpatterns = [
path('swagger/', schema_view.with_ui('swagger', cache_timeout=0), name='schema-swagger-ui'),
path('redoc/', schema_view.with_ui('redoc', cache_timeout=0), name='schema-redoc'),
]
Step 3: Run and Test the API Docs
Start the Django development server:
python manage.py runserver
Now, visit:
- Swagger UI:
http://127.0.0.1:8000/swagger/
- ReDoc UI:
http://127.0.0.1:8000/redoc/
Conclusion
You have successfully installed Django REST Framework and set up interactive API documentation. This setup will help developers explore your API seamlessly.