Designing a Smart Commentator Bot: Applying Software Patterns and Principles
Introduction Imagine a typing race game where participants compete in real-time. What if a commentator bot could enhance the experience — announcing racers, tracking progress, and throwing in quirky jokes? This isn't just a fun feature — it's a chance to apply real-world software design patterns and principles to create a maintainable, extensible solution. In this article, I’ll walk you through designing a smart commentator bot, highlighting how established software practices can guide even seemingly playful features toward clean and scalable implementations. Table of Contents The Problem: Commentating a Typing Race High-Level Architecture Choosing the Right Design Patterns Principles in Action: SOLID and DRY Handling Real-Time Updates via WebSockets Adding Personality: Jokes, Stories, and Behavior Conclusion The Problem: Commentating a Typing Race Your mission is to create a commentator bot that accompanies a real-time typing race. The bot should behave like a sports announcer — introducing participants, giving regular updates on positions, and reporting final results. But it's not just about spitting out messages. The bot needs to: Welcome users and introduce itself Announce participants with details like name and vehicle Track position and report status every 30 seconds Notify when someone is approaching or crossing the finish line Share race results at the end Tell jokes or stories between announcements Avoid offensive language And, importantly — it should do this live, using technologies like HTTP, REST, and WebSockets. The challenge? Making this fun and dynamic feature well-structured, reusable, and testable. That’s where patterns and principles come in. High-Level Architecture Before jumping into code, let's define the architecture. Here's a high-level breakdown of how the commentator bot fits into the system: [Client UI] [WebSocket Server] [Race Engine] | [Commentator Bot] Client UI – Shows the race and displays commentator messages. WebSocket Server – Broadcasts race updates to connected clients and to the bot. Race Engine – Simulates race logic (progress, positions, timing). Commentator Bot – Subscribes to race events and produces dynamic commentary. The commentator bot acts as a consumer of race events and a producer of commentary messages, which are sent back through the same WebSocket pipeline to be rendered in the UI. This separation keeps race logic clean, while allowing the commentator logic to evolve independently. Choosing the Right Design Patterns To keep our bot maintainable and adaptable, we can apply a few classic design patterns:
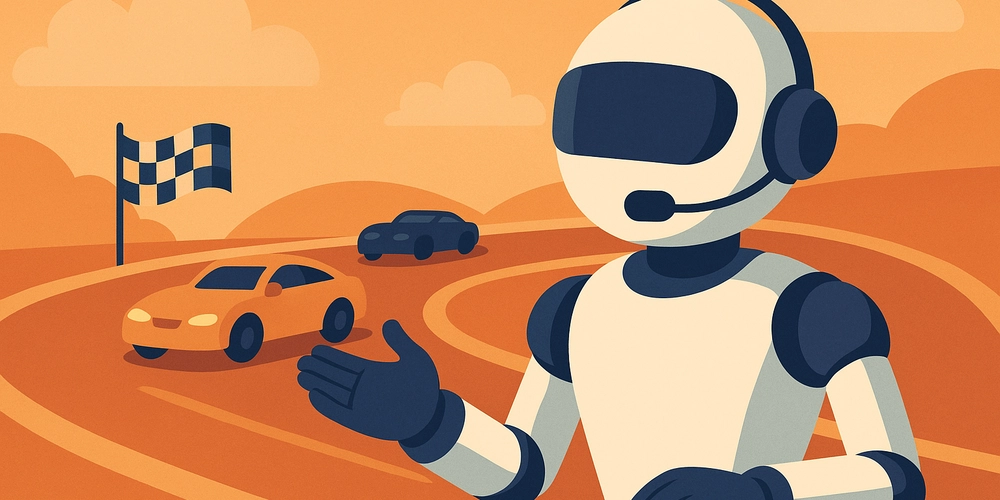
Introduction
Imagine a typing race game where participants compete in real-time. What if a commentator bot could enhance the experience — announcing racers, tracking progress, and throwing in quirky jokes? This isn't just a fun feature — it's a chance to apply real-world software design patterns and principles to create a maintainable, extensible solution.
In this article, I’ll walk you through designing a smart commentator bot, highlighting how established software practices can guide even seemingly playful features toward clean and scalable implementations.
Table of Contents
- The Problem: Commentating a Typing Race
- High-Level Architecture
- Choosing the Right Design Patterns
- Principles in Action: SOLID and DRY
- Handling Real-Time Updates via WebSockets
- Adding Personality: Jokes, Stories, and Behavior
- Conclusion
The Problem: Commentating a Typing Race
Your mission is to create a commentator bot that accompanies a real-time typing race. The bot should behave like a sports announcer — introducing participants, giving regular updates on positions, and reporting final results.
But it's not just about spitting out messages. The bot needs to:
- Welcome users and introduce itself
- Announce participants with details like name and vehicle
- Track position and report status every 30 seconds
- Notify when someone is approaching or crossing the finish line
- Share race results at the end
- Tell jokes or stories between announcements
- Avoid offensive language
And, importantly — it should do this live, using technologies like HTTP, REST, and WebSockets.
The challenge? Making this fun and dynamic feature well-structured, reusable, and testable. That’s where patterns and principles come in.
High-Level Architecture
Before jumping into code, let's define the architecture. Here's a high-level breakdown of how the commentator bot fits into the system:
[Client UI] <--> [WebSocket Server] <--> [Race Engine]
|
[Commentator Bot]
- Client UI – Shows the race and displays commentator messages.
- WebSocket Server – Broadcasts race updates to connected clients and to the bot.
- Race Engine – Simulates race logic (progress, positions, timing).
- Commentator Bot – Subscribes to race events and produces dynamic commentary.
The commentator bot acts as a consumer of race events and a producer of commentary messages, which are sent back through the same WebSocket pipeline to be rendered in the UI.
This separation keeps race logic clean, while allowing the commentator logic to evolve independently.
Choosing the Right Design Patterns
To keep our bot maintainable and adaptable, we can apply a few classic design patterns: