Designing a Scalable Architecture - with Some Spring Boot Examples
Scalability is a critical factor when designing modern backend systems. A well-structured Spring Boot application should be able to handle increasing loads efficiently, ensuring reliability and performance. In this article, we’ll explore the best practices for building a scalable architecture. What Makes an Architecture Scalable? A scalable system can handle increasing workloads by either scaling up (vertical scaling) or scaling down (horizontal scaling) while maintaining performance. The key factors that contribute to scalability include: Efficient resource management Stateless microservices Proper database scaling strategies Asynchronous processing Load balancing and caching Scaling Approaches Scaling Up (Vertical Scaling) – Increasing the power of a single machine by adding more CPU, RAM, or storage. Scaling Down (Horizontal Scaling) – Adding more machines (nodes/instances) to distribute the load. Key Components of a Scalable Architecture 1. Adopting a Microservices Architecture Instead of a monolithic approach, designing a microservices-based system allows independent scaling of each service. Break the application into smaller, independent microservices. Use Spring Cloud for configuration management. This helps update configurations dynamically without redeploying services. Deploy services independently (dockerize). 2. Stateless and Horizontally Scalable Services In a stateless architecture, the service doesn't store session data between requests. Each request is treated as an independent transaction. Instead, any necessary state information is passed with each request, typically through parameters, headers, or tokens. JWT. It enables stateless authentication by embedding user info within the token itself. How does JWT Work in a Stateless API? Client logs in → The authentication server issues a JWT upon successful login. Client includes JWT in requests → The token is passed in the Authorization header Authorization: Bearer xxxxx Service verifies JWT → The backend validates the token using a secret key or public key. Request is processed → If valid, the request is processed without needing session lookups. 3. Database Scaling Strategies A single database can become a bottleneck as traffic grows. Consider the following approaches: Database Partitioning (Sharding) – Splitting large datasets into smaller, manageable parts. Read Replicas – Creating read-only database replicas to reduce the load on the primary database.The primary database only handles writes, while multiple read replicas handle read operations, improving performance NoSQL Databases – Using MongoDB, Cassandra, or DynamoDB for high scalability needs. However, it's important to note that NoSQL databases may favor availability and partition tolerance over strong consistency. The choice between NoSQL and relational databases should depend on your application's needs, whether you prioritize consistent data for critical transactions or need high availability and fault tolerance for large-scale, distributed applications. 4. Asynchronous Processing with Message Queues Asynchronous services are often preferred over synchronous ones in scalable architectures because of their efficiency, scalability, and ability to handle high traffic volumes without blocking processes. Spring Boot integrates well with popular message brokers like Kafka, RabbitMQ and Amazon SQS. Synchronous requests block the execution until a task is completed (higher latency), so it can be more appropriate when immediate responses are needed, or when the task being performed is lightweight and doesn’t involve waiting on external resources. @Async, Spring Boot allows methods to be executed asynchronously using this annotation. Spring will run the method in a separate thread, allowing the main thread to continue without waiting for the method to complete. @Service public class EmailService { @Async public void sendEmail(String emailAddress) { // Simulate sending an email... } } ... public void execute() { emailService.sendEmail("test@gmail.com"); // The main thread continues without waiting for the email to be sent } ... 5. Load Balancing and API Gateway Distribute traffic efficiently across multiple instances to prevent overloading a single service and ensure high availability. API Gateway basically acts as a single entry point for all client requests. Lets see some of the main features. Routing & Load Balancing – Directs requests to the correct microservice based on URL paths. Authentication & Security – Implements OAuth, JWT, API keys, and rate limiting. Request Transformation – Modifies request headers, parameters, or payloads before forwarding. Caching & Compression – Improves performance by reducing redundant API calls. Circuit Breaker & Retry Mechanism – Prevents cascading failures and enhances fault tolerance. U
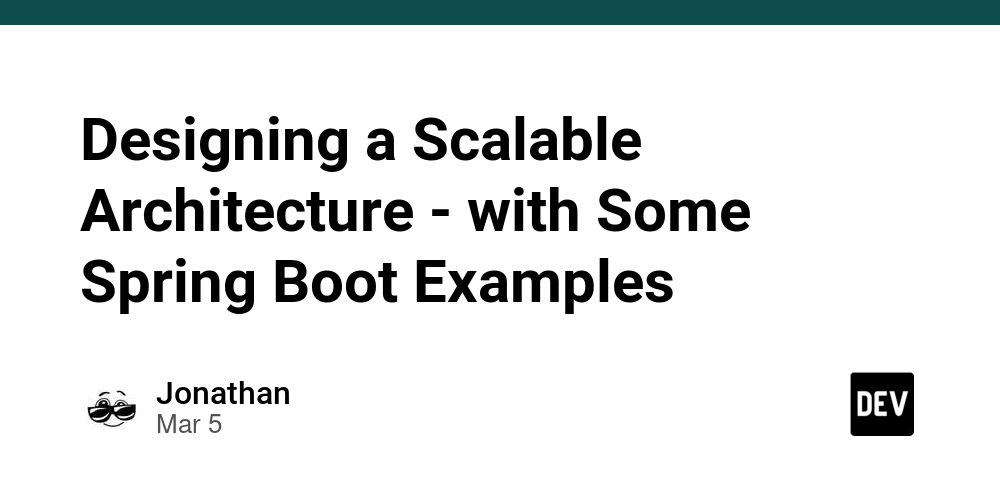
Scalability is a critical factor when designing modern backend systems. A well-structured Spring Boot application should be able to handle increasing loads efficiently, ensuring reliability and performance. In this article, we’ll explore the best practices for building a scalable architecture.
What Makes an Architecture Scalable?
A scalable system can handle increasing workloads by either scaling up (vertical scaling) or scaling down (horizontal scaling) while maintaining performance. The key factors that contribute to scalability include:
- Efficient resource management
- Stateless microservices
- Proper database scaling strategies
- Asynchronous processing
- Load balancing and caching
Scaling Approaches
- Scaling Up (Vertical Scaling) – Increasing the power of a single machine by adding more CPU, RAM, or storage.
- Scaling Down (Horizontal Scaling) – Adding more machines (nodes/instances) to distribute the load.
Key Components of a Scalable Architecture
1. Adopting a Microservices Architecture
Instead of a monolithic approach, designing a microservices-based system allows independent scaling of each service.
- Break the application into smaller, independent microservices.
- Use Spring Cloud for configuration management. This helps update configurations dynamically without redeploying services.
- Deploy services independently (dockerize).
2. Stateless and Horizontally Scalable Services
In a stateless architecture, the service doesn't store session data between requests. Each request is treated as an independent transaction. Instead, any necessary state information is passed with each request, typically through parameters, headers, or tokens.
- JWT. It enables stateless authentication by embedding user info within the token itself. How does JWT Work in a Stateless API?
- Client logs in → The authentication server issues a JWT upon successful login.
- Client includes JWT in requests → The token is passed in the Authorization header
Authorization: Bearer xxxxx
- Service verifies JWT → The backend validates the token using a secret key or public key.
- Request is processed → If valid, the request is processed without needing session lookups.
3. Database Scaling Strategies
A single database can become a bottleneck as traffic grows. Consider the following approaches:
Database Partitioning (Sharding) – Splitting large datasets into smaller, manageable parts.
Read Replicas – Creating read-only database replicas to reduce the load on the primary database.The primary database only handles writes, while multiple read replicas handle read operations, improving performance
NoSQL Databases – Using MongoDB, Cassandra, or DynamoDB for high scalability needs. However, it's important to note that NoSQL databases may favor availability and partition tolerance over strong consistency. The choice between NoSQL and relational databases should depend on your application's needs, whether you prioritize consistent data for critical transactions or need high availability and fault tolerance for large-scale, distributed applications.
4. Asynchronous Processing with Message Queues
Asynchronous services are often preferred over synchronous ones in scalable architectures because of their efficiency, scalability, and ability to handle high traffic volumes without blocking processes. Spring Boot integrates well with popular message brokers like Kafka, RabbitMQ and Amazon SQS. Synchronous requests block the execution until a task is completed (higher latency), so it can be more appropriate when immediate responses are needed, or when the task being performed is lightweight and doesn’t involve waiting on external resources.
@Async
, Spring Boot allows methods to be executed asynchronously using this annotation. Spring will run the method in a separate thread, allowing the main thread to continue without waiting for the method to complete.
@Service
public class EmailService {
@Async
public void sendEmail(String emailAddress) {
// Simulate sending an email...
}
}
...
public void execute() {
emailService.sendEmail("test@gmail.com");
// The main thread continues without waiting for the email to be sent
}
...
5. Load Balancing and API Gateway
Distribute traffic efficiently across multiple instances to prevent overloading a single service and ensure high availability.
API Gateway basically acts as a single entry point for all client requests. Lets see some of the main features.
- Routing & Load Balancing – Directs requests to the correct microservice based on URL paths.
- Authentication & Security – Implements OAuth, JWT, API keys, and rate limiting.
- Request Transformation – Modifies request headers, parameters, or payloads before forwarding.
- Caching & Compression – Improves performance by reducing redundant API calls.
- Circuit Breaker & Retry Mechanism – Prevents cascading failures and enhances fault tolerance.
Using both, Load Balancers and API Gateways ensures a resilient, scalable, and secure architecture
Clients --> Load Balancer --> API Gateway --> service A/service B....
6. Caching for Performance Optimization
Reduce database queries by caching frequently accessed data.
- Redis, Amazon ElastiCache, Spring cache Abstraction.
Spring Cache Abstraction allows you to define caching behavior declaratively using annotations. This removes the need to write low-level caching logic manually. Most commonly:
@Cacheable
: Caches the result of a method based on the method parameters. If the same method is called again with the same parameters, the cached result is returned instead of executing the method.
@Cacheable("users") // 'users' is the cache name and the method params the key
public User getUserById(Long id) {
return userRepository.findById(id);
}
@CachePut
: Updates the cache with the result of a method, but always executes the method, unlike @Cacheable
, which may skip execution if the result is cached.
@CachePut(value = "users", key = "#user.id")
public User updateUser(User user) {
return userRepository.save(user);
}
@CacheEvict
: Removes entries from the cache. It can be used to clear the cache based on conditions or manually evict data when it’s no longer needed.
@CacheEvict(value = "users", key = "#id")
public void deleteUser(Long id) {
userRepository.deleteById(id);
}
Conclusion
The previous points are some of the key considerations when designing scalable architectures. However, other important factors, such as logging, security, monitoring, error handling, retry mechanisms, etc etc.., should also be considered. Incorporating these aspects ensures that your application remains robust, secure, and easy to maintain as it grows and evolves.