Debouncing and Throttling with examples
Debouncing and throttling are two techniques used in JavaScript to optimize the execution of functions that are triggered frequently, especially in response to user interactions like scrolling, resizing, or typing. Debouncing Debouncing ensures that a function runs only after a specified delay following the last trigger event. This prevents excessive function calls when an event fires repeatedly. Real-world Example: Typing in a Search Bar Imagine a user typing into a search bar. Without debouncing, every keystroke would trigger an API request, potentially overwhelming the server. Instead, with debouncing, the function waits until the user stops typing for a short period before making the request. JavaScript Example (Debouncing a Search Input): function debounce(func, delay) { let timer; return function (...args) { clearTimeout(timer); timer = setTimeout(() => func.apply(this, args), delay); }; } const searchAPI = (query) => { console.log("Fetching search results for:", query); }; const debouncedSearch = debounce(searchAPI, 500); document.getElementById("searchBox").addEventListener("input", (event) => { debouncedSearch(event.target.value); });
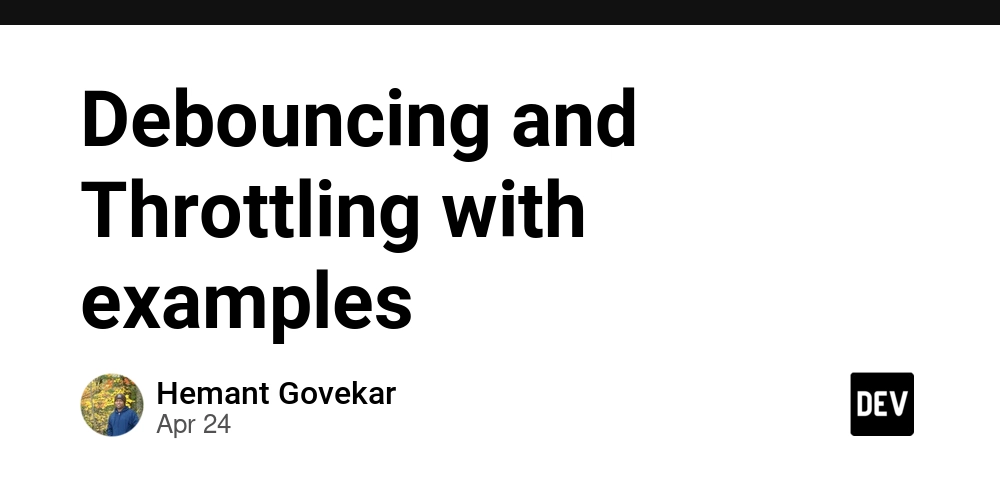
Debouncing and throttling are two techniques used in JavaScript to optimize the execution of functions that are triggered frequently, especially in response to user interactions like scrolling, resizing, or typing.
Debouncing
Debouncing ensures that a function runs only after a specified delay following the last trigger event. This prevents excessive function calls when an event fires repeatedly.
Real-world Example: Typing in a Search Bar
Imagine a user typing into a search bar. Without debouncing, every keystroke would trigger an API request, potentially overwhelming the server. Instead, with debouncing, the function waits until the user stops typing for a short period before making the request.
JavaScript Example (Debouncing a Search Input):
function debounce(func, delay) {
let timer;
return function (...args) {
clearTimeout(timer);
timer = setTimeout(() => func.apply(this, args), delay);
};
}
const searchAPI = (query) => {
console.log("Fetching search results for:", query);
};
const debouncedSearch = debounce(searchAPI, 500);
document.getElementById("searchBox").addEventListener("input", (event) => {
debouncedSearch(event.target.value);
});