Day - 5 at payilagam "Types of arguments"
Default argument: def login (username, password = 'test@123') print(username,'logged in with password',password) login('admin','abcd1234') login('admin') output: admin logged in with password abcd1234 admin logged in with password test@123 If argument is not given, default value present in function parameter present in function parameter will be considered. Positional argument: def login (username,password): print(username, 'logged in with password',password) login('admin','abcd1234') output: admin logged in with password abcd1234 Only if the both parameters are given, then only the code proceeds to give the output.This is the argument which is widely used. Keyword argument: def login(username,password): print(username,'logged in with password',password) login(password = 'abcd1234', username = 'admin') output: admin logged in with password abcd1234 in this argument the value of the parameter is passed by keyword. The keyword defines the parameter value. variable-length argument: def dhoni_score (*no_of_matches) print(no_of_matches) print(type(no_of_matches) dhoni_score(32) dhoni_score(32,16) dhoni_score(32,16,4) output: (32,) (32, 16) (32, 16, 4) This argument can take many parameters as it only considers as variables only.This parameter can take many parameters. type function defines the type of the datatypes. id function defines the memory location of the datatypes. how to know the type of the function: def add(no1,no2): print(no1+no2) print(add) output: def add(no1,no2): print(no1+no2) print(add(20,30)) output: 50 None Just print the function you'll get the function type. Keyword variable length Arguments: def dhoni_results (**score): print(score) dhoni_results(mi=30,kkr=32,rr=57) output: {'mi': 30, 'kkr': 32, 'rr': 57} Just the combination of keyword and variable length arguments. Task: Function with positional, default, and keyword arguments def add(a, b=5): result = a + b print(result) Positional Argument (b is default 5) add(10) # Output: 15 Positional Argument (explicit value for b) add(10, 20) # Output: 30 Function with variable-length arguments (*args) def sum_numbers(*args): total = 0 for num in args: total += num print(total) Variable-length Argument (passing multiple values) sum_numbers(1, 2, 3) # Output: 6 sum_numbers(5, 10, 15) # Output: 30 Function with keyword variable-length arguments (kwargs) def sum_with_weights(kwargs): total = 0 for value in kwargs.values(): total += value print(total) Keyword Variable-length Argument (passing multiple key-value pairs) sum_with_weights(a=1, b=2, c=3) # Output: 6
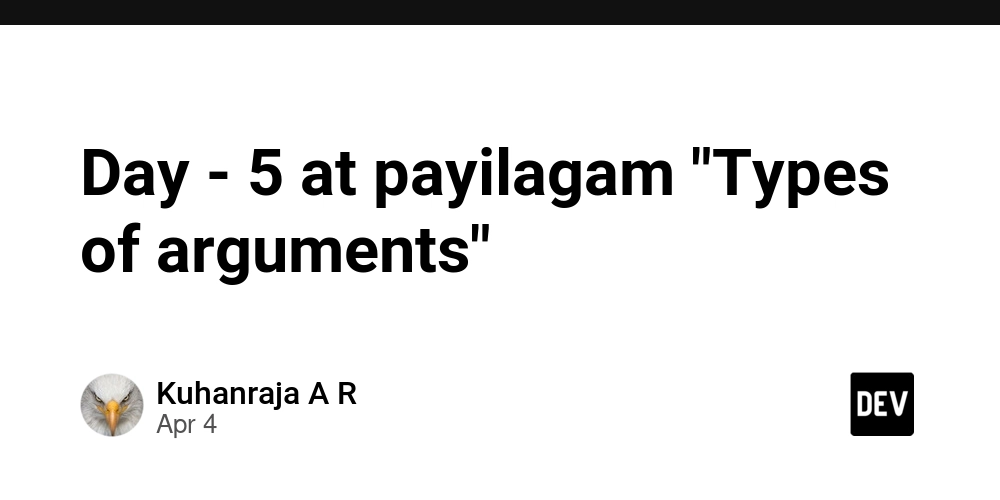
Default argument:
def login (username, password = 'test@123')
print(username,'logged in with password',password)
login('admin','abcd1234')
login('admin')
output:
admin logged in with password abcd1234
admin logged in with password test@123
If argument is not given, default value present in function parameter present in function parameter will be considered.
Positional argument:
def login (username,password):
print(username, 'logged in with password',password)
login('admin','abcd1234')
output:
admin logged in with password abcd1234
Only if the both parameters are given, then only the code proceeds to give the output.This is the argument which is widely used.
Keyword argument:
def login(username,password):
print(username,'logged in with password',password)
login(password = 'abcd1234', username = 'admin')
output:
admin logged in with password abcd1234
in this argument the value of the parameter is passed by keyword.
The keyword defines the parameter value.
variable-length argument:
def dhoni_score (*no_of_matches)
print(no_of_matches)
print(type(no_of_matches)
dhoni_score(32)
dhoni_score(32,16)
dhoni_score(32,16,4)
output:
(32,)
(32, 16)
(32, 16, 4)
This argument can take many parameters as it only considers as variables only.This parameter can take many parameters.
type function defines the type of the datatypes.
id function defines the memory location of the datatypes.
how to know the type of the function:
def add(no1,no2):
print(no1+no2)
print(add)
output:
def add(no1,no2):
print(no1+no2)
print(add(20,30))
output:
50
None
Just print the function you'll get the function type.
Keyword variable length Arguments:
def dhoni_results (**score):
print(score)
dhoni_results(mi=30,kkr=32,rr=57)
output:
{'mi': 30, 'kkr': 32, 'rr': 57}
Just the combination of keyword and variable length arguments.
Task:
Function with positional, default, and keyword arguments
def add(a, b=5):
result = a + b
print(result)
Positional Argument (b is default 5)
add(10) # Output: 15
Positional Argument (explicit value for b)
add(10, 20) # Output: 30
Function with variable-length arguments (*args)
def sum_numbers(*args):
total = 0
for num in args:
total += num
print(total)
Variable-length Argument (passing multiple values)
sum_numbers(1, 2, 3) # Output: 6
sum_numbers(5, 10, 15) # Output: 30
Function with keyword variable-length arguments (kwargs)
def sum_with_weights(kwargs):
total = 0
for value in kwargs.values():
total += value
print(total)
Keyword Variable-length Argument (passing multiple key-value pairs)
sum_with_weights(a=1, b=2, c=3) # Output: 6