day-30: enumeration in java
What is enumeration? An enum type is a special data type that enables for a variable to be a set of predefined constants. The variable must be equal to one of the values that have been predefined for it. In programming, an "enum type" (or enumeration) is a data type that consists of a set of named constants, allowing you to represent a fixed set of values, such as days of the week or colors. What it is: Fixed Set of Values: Enums define a specific, limited set of possible values for a variable. Named Constants: Each value in the enum is represented by a named constant, making the code more readable and maintainable. Type Safety: Enums provide type safety, ensuring that a variable can only hold one of the defined enum values, preventing errors. Examples: Days of the Week: enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY } Compass Directions: enum Direction { NORTH, SOUTH, EAST, WEST } Color: enum Color { RED, GREEN, BLUE } Status: enum Status { ACTIVE, INACTIVE, PENDING } How it's used: Readability: Enums make code easier to understand by using meaningful names instead of raw numerical values. Maintainability: When the set of possible values changes, you only need to modify the enum definition, not multiple places in the code. Error Prevention: Enums help prevent errors by ensuring that a variable can only hold one of the predefined values. Type Safety: Enums enforce type safety, ensuring that variables of an enum type can only hold values of that enum type. In Different Programming Languages: Java: public enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
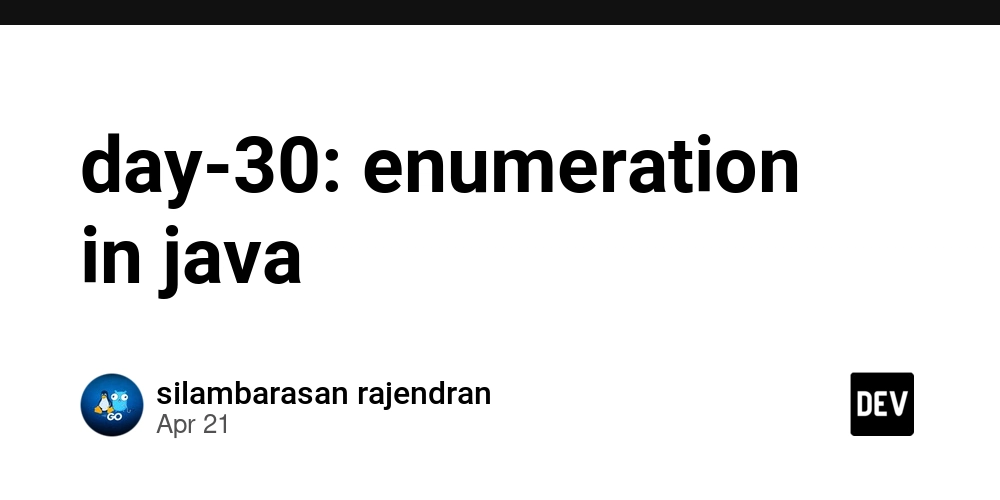
What is enumeration?
An enum type is a special data type that enables for a variable to be a set of predefined constants. The variable must be equal to one of the values that have been predefined for it.
In programming, an "enum type" (or enumeration) is a data type that consists of a set of named constants, allowing you to represent a fixed set of values, such as days of the week or colors.
What it is:
Fixed Set of Values: Enums define a specific, limited set of possible values for a variable.
Named Constants: Each value in the enum is represented by a named constant, making the code more readable and maintainable.
Type Safety: Enums provide type safety, ensuring that a variable can only hold one of the defined enum values, preventing errors.
Examples:
Days of the Week: enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
Compass Directions: enum Direction { NORTH, SOUTH, EAST, WEST }
Color: enum Color { RED, GREEN, BLUE }
Status: enum Status { ACTIVE, INACTIVE, PENDING }
How it's used:
Readability:
Enums make code easier to understand by using meaningful names instead of raw numerical values.
Maintainability:
When the set of possible values changes, you only need to modify the enum definition, not multiple places in the code.
Error Prevention:
Enums help prevent errors by ensuring that a variable can only hold one of the predefined values.
Type Safety:
Enums enforce type safety, ensuring that variables of an enum type can only hold values of that enum type.
In Different Programming Languages:
Java: public enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }