day-26: Encapsulation and Abstraction in Java
Encapsulation: In Java that involves bundling the data (variables) and the methods (functions) that operate on the data into a single unit, known as a class. It restricts direct access to some of an object's components and can prevent the accidental modification of data. 1) private - Accessible within the same class only 2) default - Accessible within the same package (no keyword used) 3) protected - Accessible within the same package AND subclasses in other packages 4) public - Accessible from anywhere Use this When you... --> import Want to use a class from a different package --> extends Want to inherit functionality from another class Example code for protected: package housingloandpackage; public class HDFC { protected void education_load () { System.out.println("Educational load"); } public static void main(String[] args) { // TODO Auto-generated method stub } } package educationloanpackage; import housingloandpackage.HDFC; public class IOB extends HDFC { public static void main(String[] args) { IOB iob = new IOB(); iob.education_load(); } } package educationloanpackage; import housingloandpackage.HDFC; public class SBI { public static void main(String[] args) { HDFC hdfc = new HDFC(); hdfc.emp_salary(); } } IS-A Relationship only we can use extends key. Note: --> If we extent the class we can use our own object or else we need to create new object for the class. Abstraction Abstraction in Java is a fundamental object-oriented programming concept that involves hiding complex implementation details and presenting only the essential features to the user. public abstract class Parents { public abstract void study(); public void grow() { System.out.println("Take Care"); } public static void main(String[] args) { //Parents parents = new Parents(); //parents.study(); Can't do that. } } Here: 1). Class and Method can be abstract. 2). It cannot be instantiated directly. - Can't create Object. -------------------------- End of the Blog ------------------------
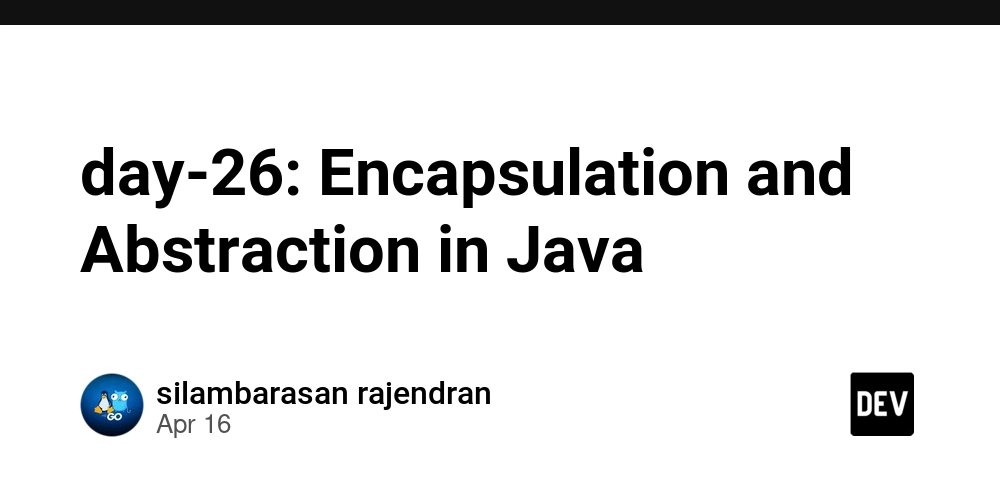
Encapsulation:
In Java that involves bundling the data (variables) and the methods (functions) that operate on the data into a single unit, known as a class. It restricts direct access to some of an object's components and can prevent the accidental modification of data.
1) private - Accessible within the same class only
2) default - Accessible within the same package (no keyword used)
3) protected - Accessible within the same package AND subclasses in other packages
4) public - Accessible from anywhere
Use this When you...
--> import Want to use a class from a different package
--> extends Want to inherit functionality from another class
Example code for protected:
package housingloandpackage;
public class HDFC {
protected void education_load () {
System.out.println("Educational load");
}
public static void main(String[] args) {
// TODO Auto-generated method stub
}
}
package educationloanpackage;
import housingloandpackage.HDFC;
public class IOB extends HDFC {
public static void main(String[] args) {
IOB iob = new IOB();
iob.education_load();
}
}
package educationloanpackage;
import housingloandpackage.HDFC;
public class SBI {
public static void main(String[] args) {
HDFC hdfc = new HDFC();
hdfc.emp_salary();
}
}
IS-A Relationship only we can use extends key.
Note:
--> If we extent the class we can use our own object
or else we need to create new object for the class.
Abstraction
Abstraction in Java is a fundamental object-oriented programming concept that involves hiding complex implementation details and presenting only the essential features to the user.
public abstract class Parents {
public abstract void study();
public void grow() {
System.out.println("Take Care");
}
public static void main(String[] args) {
//Parents parents = new Parents();
//parents.study(); Can't do that.
}
}
Here:
1). Class and Method can be abstract.
2). It cannot be instantiated directly. - Can't create Object.
-------------------------- End of the Blog ------------------------